React Context API
Using Context with the `useContext` Hook
The useContext
Hook is one of the simplest and most effective ways to consume contexts in React functional components. Introduced in React 16.8, useContext
significantly improves code readability and conciseness. In this chapter, we will delve into the use of the useContext
Hook.
What is the useContext
Hook?
The useContext
Hook allows us to access context values directly within a functional component, eliminating the need for the Consumer
component and the render props pattern. This makes the code simpler and easier to maintain.
Basic Example
Let's look at a basic example using useContext
:
jsx
In this example, useContext(UserContext)
is called within the UserProfile
component to access the context data.
Advantages of useContext
- Simplicity:
useContext
eliminates the need to write aConsumer
component and use render props, making the code more straightforward. - Readability: The code is easier to read and understand, which is especially useful in large applications.
- Less Nesting: With
useContext
, the nesting that occurs when using multipleConsumers
is reduced.
Comparison between useContext
and Context Consumer
Using Context Consumer
jsx
Using useContext
jsx
As you can see, useContext
makes the code clearer and less verbose.
[Placeholder: Comparative image between Context Consumer
and useContext
, showing how useContext
simplifies the code]
Memoization and useContext
To optimize performance, especially when the context value is an object or a function, it is recommended to memoize these values using useMemo
or useCallback
.
Example with useMemo
jsx
In this example, useMemo
ensures that the context value only updates when user
changes, avoiding unnecessary re-renders.
Handling Multiple Contexts
When your application requires multiple contexts, you can use several useContext
hooks within the same component.
Example with Multiple Contexts
jsx
In this example, UserProfile
consumes values from both UserContext
and ThemeContext
.
Conclusion
The useContext
Hook is a powerful and simple tool for consuming contexts in React functional components. It facilitates access to context data, improves code readability, and reduces unnecessary nesting. In the next chapter, we will compare the Context API with the prop drilling pattern and discuss the advantages and disadvantages of each approach.
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices
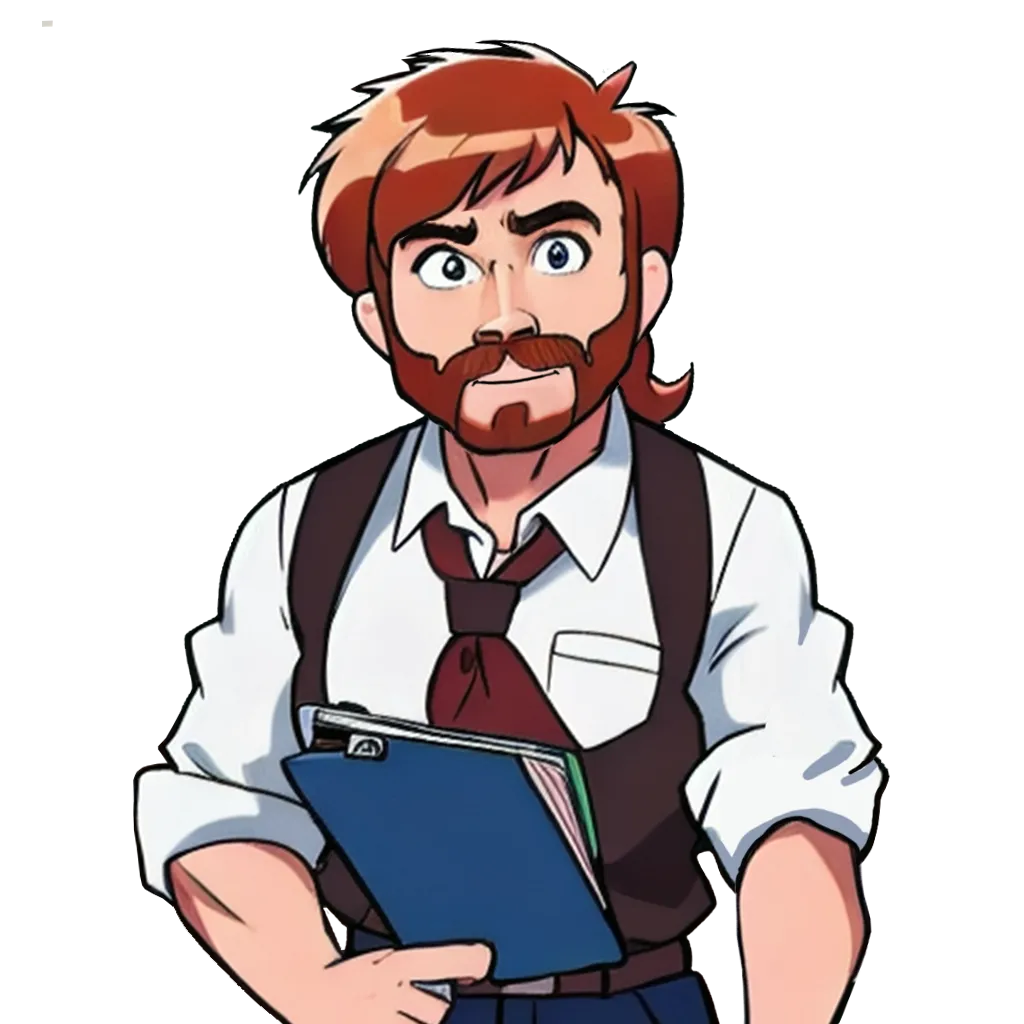