React Context API
Fundamentals of State in React
To fully understand React's Context API, we must first have a solid foundation in the fundamentals of state in React. State is an essential feature in React as it allows components to maintain and manage changing data throughout the application's lifecycle.
What is State?
State is an object that contains data or properties that a component can handle and render dynamically. Unlike props, which are immutable and passed down from a parent component, state is mutable and managed internally by the component.
Initializing State
In functional components, state is managed using the useState
Hook. In class components, state is initialized in the constructor.
Example in Functional Component
jsx
In this example, useState
is used to declare a state variable called count
and a function setCount
to update that state.
Example in Class Component
jsx
Here, the state is initialized in the constructor and updated using this.setState
within the increment
method.
Updating State
Updating state is a crucial part of React as it triggers a re-render of the component to reflect the changes. It's important to note that state updates in React are asynchronous.
Considerations When Updating State
- Do Not Modify State Directly: Always use the update function (
setState
oruseState
) to change the state. Directly modifying it will not trigger a re-render.
jsx
- Combine State Updates: When the new state depends on the previous state, use the state update function that receives the previous state as an argument.
jsx
State vs. Props
- State: Managed internally by the component, mutable, and used to store information that can change during the component's lifecycle.
- Props: Passed down from the parent component, immutable, and used to configure the component.
Combined Example
Below is an example that demonstrates how a component can handle both state and props.
jsx
In this example, the Greeting
component receives a name
prop and manages a greeting
state.
Conclusion
Understanding how state works in React is fundamental for effectively working with the Context API. State allows components to manage data that can change over time, and it's essential for building interactive and dynamic applications. In the next chapter, we will delve into creating a context in React.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
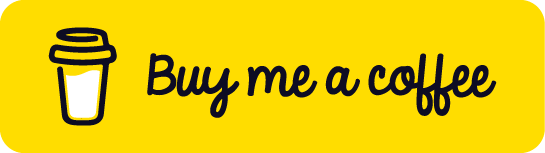
Chat with Chuck
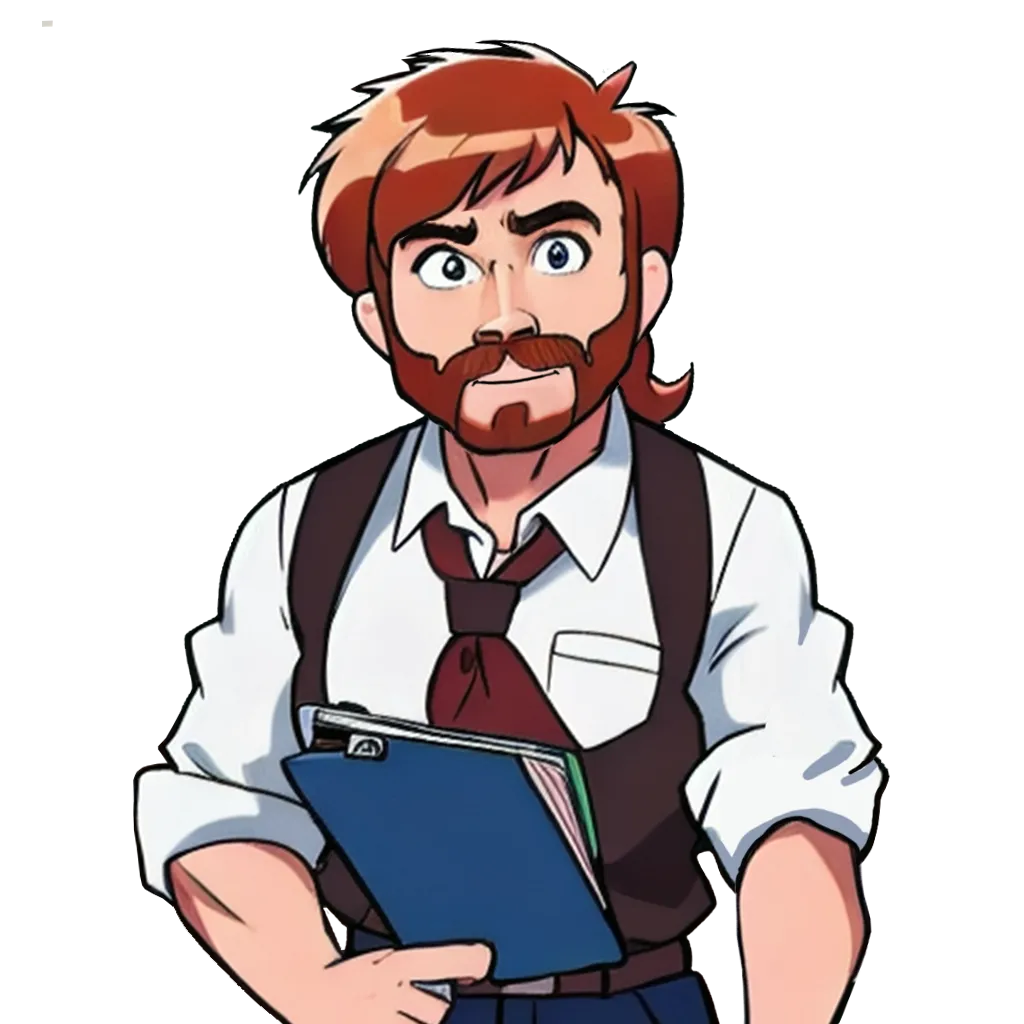
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices