React Context API
Context Consumer (Context Consumer)
The Context Consumer
is an integral part of React's Context API. It is used to access data provided by the context Provider
. This chapter will cover how to use the Context Consumer
effectively and best practices for its use.
What is a Context Consumer?
The Context Consumer
is a component provided by createContext()
that allows components to access context values. The most common way to use it is through the render props pattern, where a function is passed as a child of the Consumer
, and this function receives the context value as an argument.
Basic Example
Let's see how to use the Context Consumer
in a simple example:
jsx
In this example, UserContext.Consumer
wraps the UserProfile
component and uses a function to access the context value.
Render Props Pattern
The Context Consumer
uses the render props pattern, which means it expects a function as a child. This function receives the context value as an argument and returns a React Node.
jsx
Using the useContext
Hook
Starting from React 16.8, the useContext
Hook simplifies the way of consuming contexts. Instead of using the Consumer
component, we can use the useContext
Hook to access the context directly within functional components.
Example with useContext
jsx
In this example, useContext
is used to access the context within the UserProfile
functional component.
Comparison between Consumer
and useContext
Context Consumer
: Uses a render props pattern, which can be less intuitive and more verbose, especially in large or nested components.useContext
: Cleaner and more concise, improving readability and reducing the amount of code.
Special Considerations
- Re-renders: Every time the context value changes, all consumers re-render. This can affect performance if not handled properly.
- Nested Contexts: When working with nested contexts, make sure each
Provider
andConsumer
corresponds to the correct context.
Example of Re-renders
jsx
In this example, CounterDisplay
re-renders every time the counter is incremented since the context value changes.
[Placeholder: Image showing how the Consumer
accesses values from the Provider
at different levels of the component tree]
Best Practices
- Using
useContext
: PreferuseContext
in functional components for cleaner and more concise code. - Memoization: Memoize context values that are objects or functions to avoid unnecessary re-renders.
- Single Responsibility: Keep components responsible for consuming the context small and focused on a single task.
Conclusion
The Context Consumer
and the useContext
Hook are essential tools for accessing context values in React. Understanding how to use them correctly can improve your application's efficiency and code readability. In the next chapter, we will explore the use of context with the useContext
Hook in greater detail.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
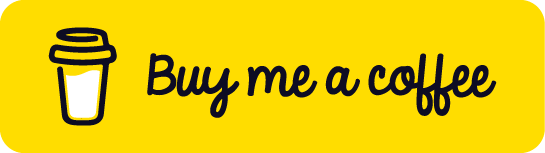
Chat with Chuck
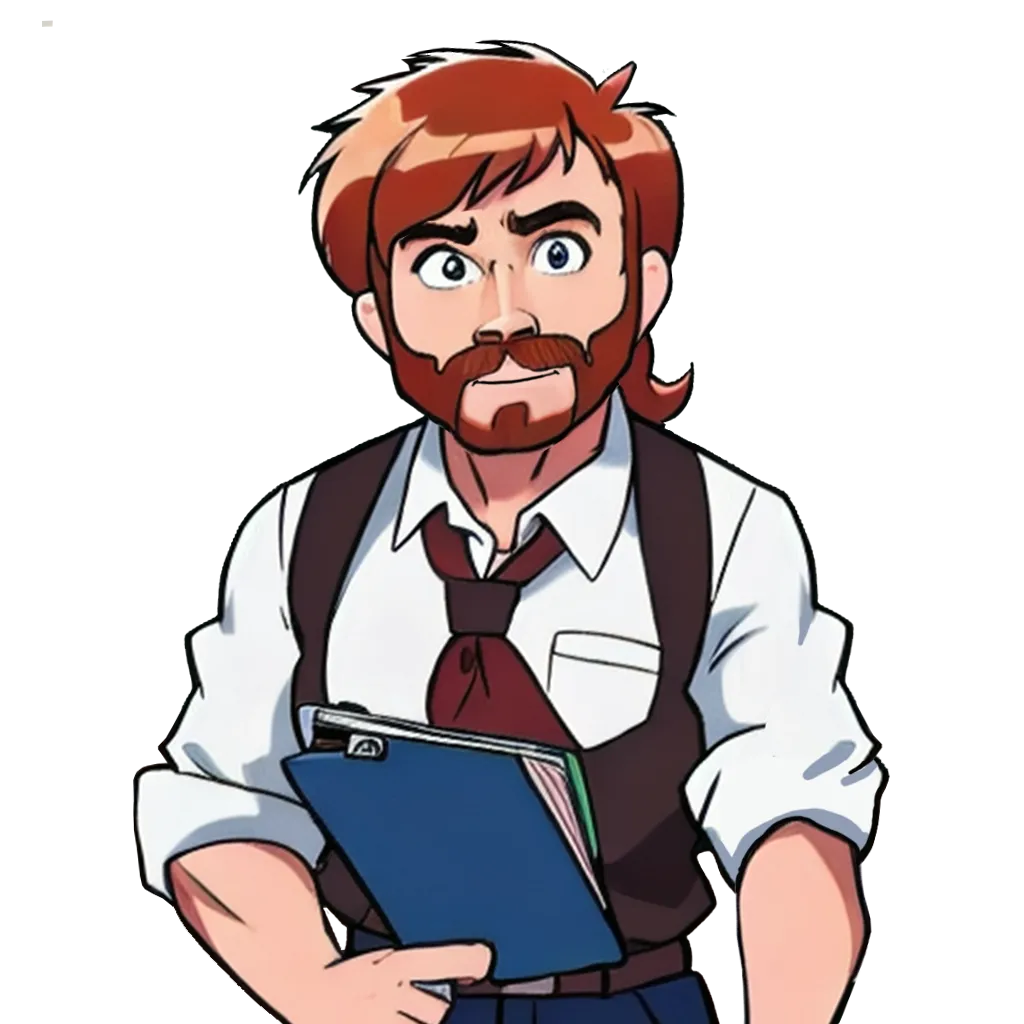
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices