React Context API
Context API and Class Components
Although functional components combined with hooks have gained popularity in the React community, class components remain relevant and are used in many applications. In this chapter, we will explore how to use the Context API with class components, including best practices and common patterns.
Using Context API in Class Components
Unlike functional components that use the useContext
hook, class components access context through a Consumer
component or the static contextType
context.
Basic Example with Context.Consumer
jsx
In this example, UserProfile
accesses the context using UserContext.Consumer
.
Using contextType
Another way to consume context in class components is by using the static contextType
property. This allows the component to access the context through this.context
.
Example with contextType
jsx
In this example, UserProfile
accesses the context directly through this.context
using the static contextType
property.
Comparison between Context.Consumer
and contextType
Using Context.Consumer
Advantages:
- Applicable to more than one context.
- Does not require the use of a static property, which can be more flexible in some cases.
Disadvantages:
- Can result in less clean code due to render props functions.
Using contextType
Advantages:
- Cleaner and more concise, especially when working with a single context.
- Direct access to
this.context
simplifies the code.
Disadvantages:
- Limited to a single context per component.
Updating Context Content in Class Components
To update the context content from class components, an update function is passed within the context value.
Update Example
jsx
In this example, UserProfile
updates the context by calling the setUser
function provided in the context.
[Placeholder: Image comparing the use of Context.Consumer
and contextType
in class components, highlighting the differences in context access and updating]
Performance Considerations
As with functional components, it is important to consider how changes in context can trigger re-renders in class components. Ensure to optimize context usage to avoid unnecessary renders.
Conclusion
The Context API can be effectively used in both functional and class components. Understanding how to apply these techniques in class components can be crucial for maintaining and optimizing legacy applications or those still using this style. In the next chapter, we will explore how to test applications that use the Context API to ensure their correct and reliable operation.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
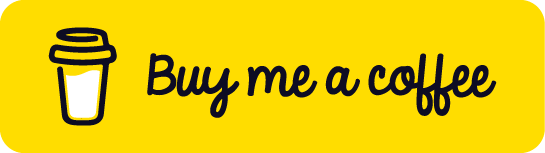
Chat with Chuck
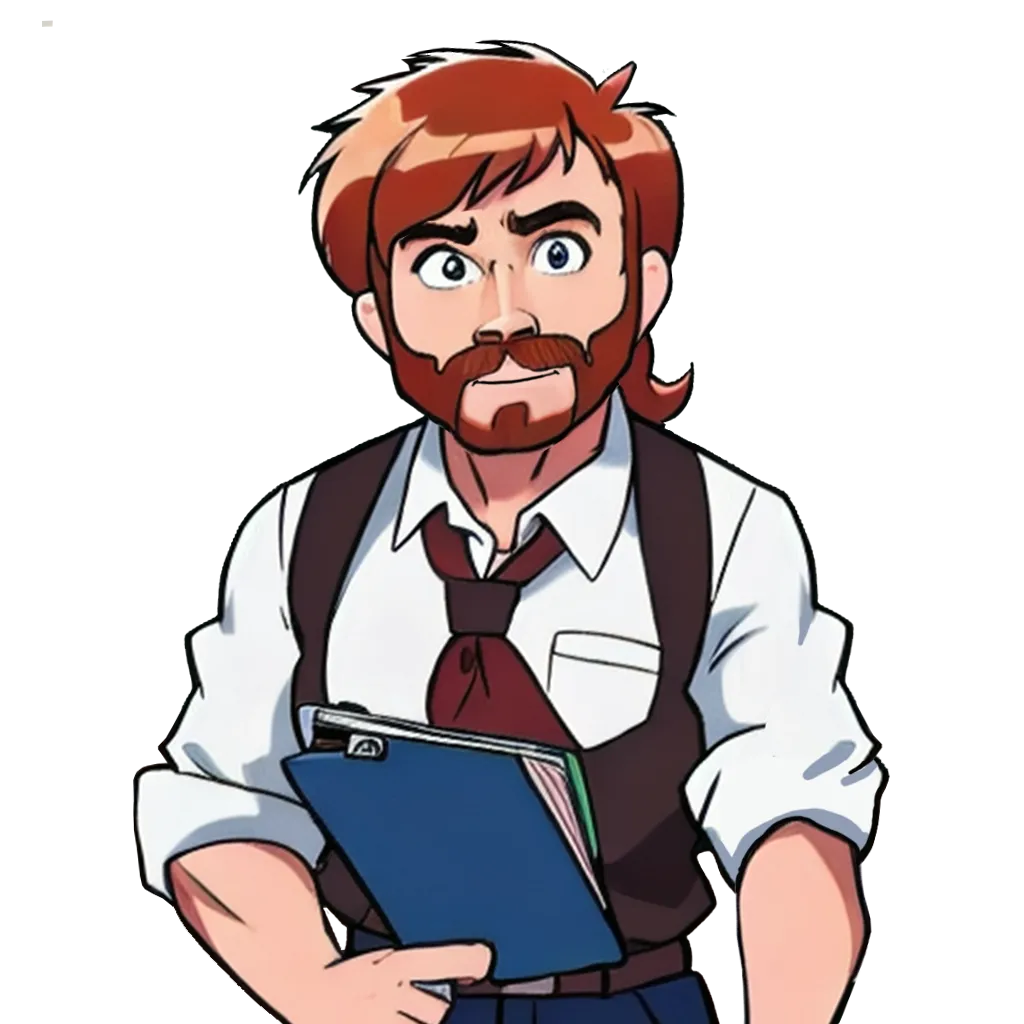
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices