React Context API
Context Update
The ability to update context values in a React application is crucial for managing global states that may change over time, such as user data or application settings. In this chapter, we will explore different strategies and best practices for efficiently updating context.
Using an Update Function
A common way to allow components to update context is to pass an update function along with the initial context value. This is achieved by providing an object that contains both the state and the update function to the Provider
.
Basic Example
We will use the useState
Hook to manage state and provide the update function in the context.
jsx
In this example, the UserContext
context provides both the user data and the setUser
function to update those data.
Reducing Unnecessary Renders
Whenever the context value changes, all context consumers re-render. To minimize unnecessary renders, it can be helpful to memoize the context object using useMemo
.
Example with useMemo
jsx
In this example, useMemo
is used to create a memoized version of the context value and only update it when user
changes, avoiding unnecessary re-renders.
Using Separate Contexts
In large applications, it can be useful to split the global state into multiple separate contexts, each responsible for a different part of the state. This can help optimize performance and maintain code clarity.
Example with Separate Contexts
jsx
In this example, UserContext
and ThemeContext
are handled separately, improving the organization and performance of the application.
Performance Considerations
- Minimize Updates: Memoize objects and functions in context to minimize unnecessary re-renders.
- Split State: Use separate contexts for different parts of the global state. This can improve application clarity and performance.
- Update Functions: Provide specific functions to update context and avoid passing large objects that frequently change.
Conclusion
Efficiently updating context is key to maintaining a performant and easy-to-maintain React application. Using update functions, memoizing values, and splitting global state into multiple contexts are effective strategies to achieve this goal. In the next chapter, we will explore the intersection of nested contexts and how to handle them effectively.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
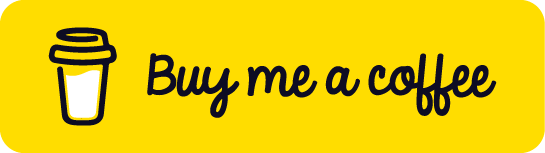
Chat with Chuck
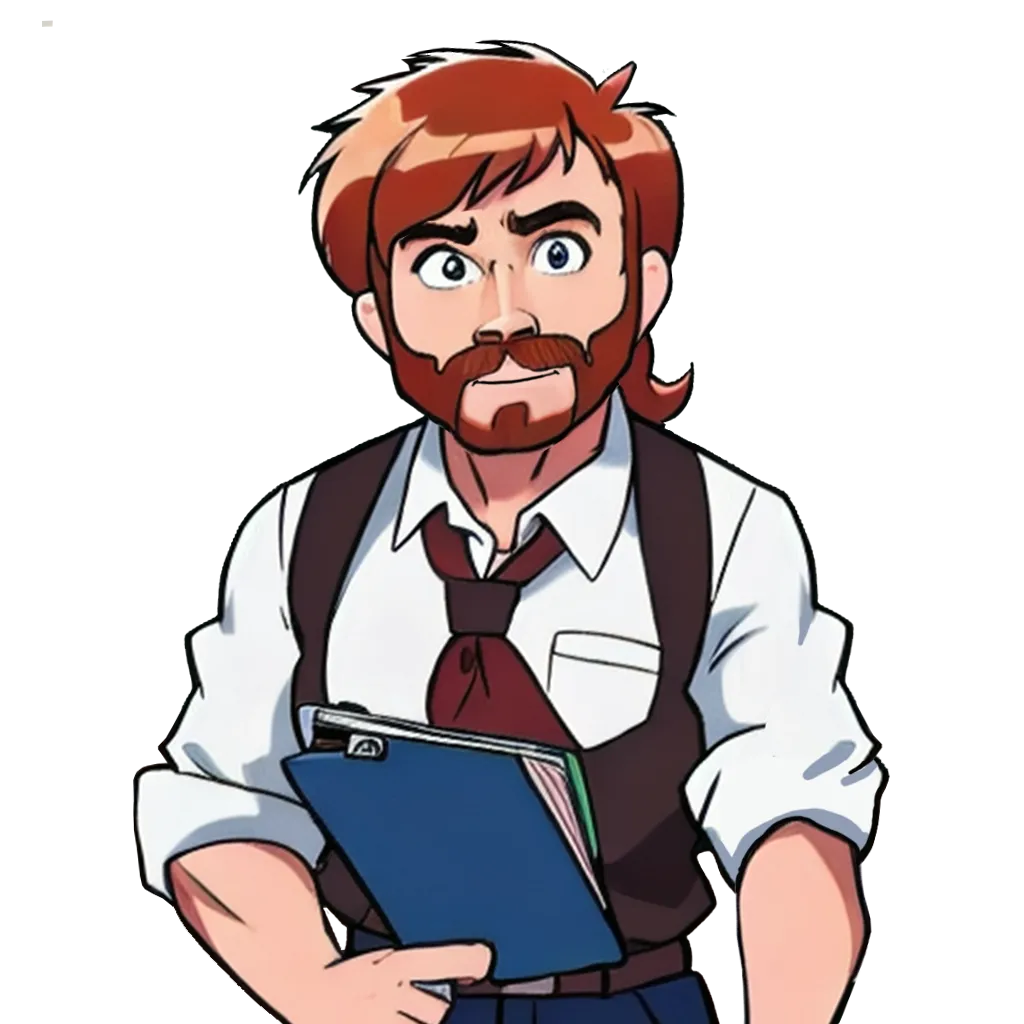
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices