React Context API
Creating a Context in React
Creating a context in React is the first step to using the Context API. A context is created using the React.createContext
function and is essential for efficiently sharing data in components that are not directly related.
Steps to Create a Context
- Import React and createContext
- Create a Context
- Provide the Context
- Consume the Context
1. Import React and createContext
First, we need to import React
and the createContext
function from the React library.
jsx
2. Create a Context
We use createContext
to create a new context. This function returns an object with two components: Provider
and Consumer
.
jsx
In this example, MyContext
is now a context that can be used in our application.
3. Provide the Context
To make the context available in the component tree, we need to wrap the components where we want the context to be accessible with the context's Provider
component.
jsx
Here we are providing the user
value to the MyContext
context, which makes any component within MyContext.Provider
able to access this value.
4. Consume the Context
To access the context's data, we use the Consumer
component provided by the context.
jsx
In this example, UserProfile
consumes the context value and uses it to render user information.
Complete Example
Combining all the steps, here is a complete example of creating and using a context in React.
jsx
Using useContext
Hook
Starting with React 16.8, you can also use the useContext
Hook to consume the context in a simpler way.
jsx
The useContext
Hook simplifies the code required to consume the context, making it cleaner and easier to read.
Conclusion
Creating a context in React is a simple process that can significantly improve how we share data between components. By understanding the process of creating and utilizing a context, we can build more efficient and easy-to-maintain applications. In the next chapter, we will dive deeper into the context Provider
component.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
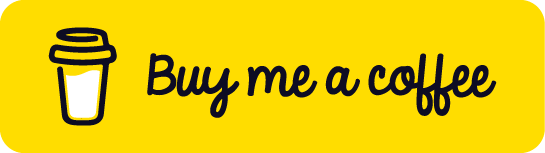
Chat with Chuck
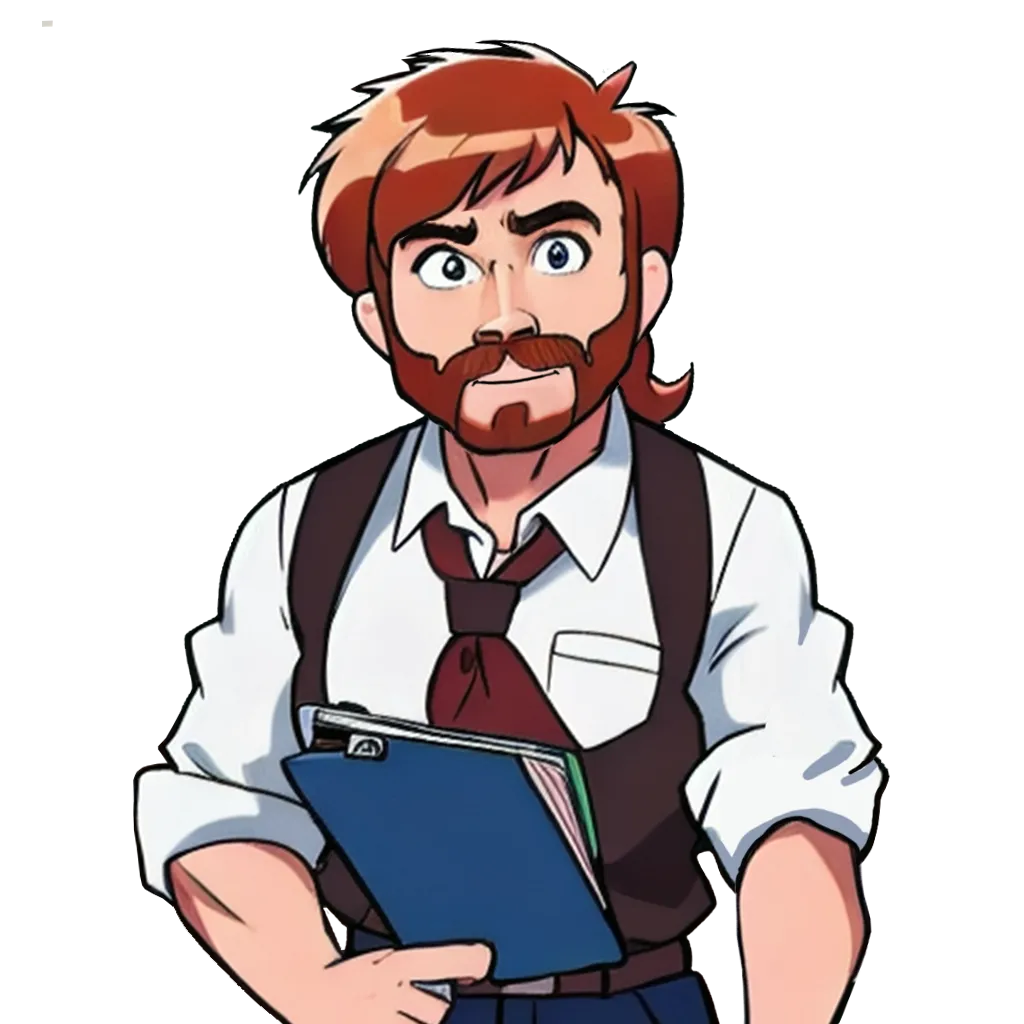
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices