React Context API
Introduction to the React Context API
The React Context API is a powerful tool that allows for passing data through the React component tree without the need to manually pass props at every level. It was introduced in React 16.3 and has been widely adopted to handle cases where multiple components need to share the same dataset.
Why use {Context API}?
In React applications, sharing data among components can become complicated, especially if that data needs to be accessed by multiple nested component levels. Traditionally, a pattern called "prop drilling" is used, which means manually passing props from the parent component to the child components, and to the children's children, until reaching the component that actually needs the data. This approach can make the code difficult to maintain and scale.
Key Concepts
- Context: An object created by
React.createContext()
that contains two components:Provider
andConsumer
. - Provider: A component that wraps the part of the component tree that needs access to the context, allowing these data to be available to all child components.
- Consumer: A component that can access and use the context data.
When should you use Context API?
Using the Context API is ideal in the following cases:
- User Authentication: Maintaining authentication state and user data throughout the application.
- Theming: Passing appearance themes (colors, fonts, etc.) across the application.
- Application Settings: Global values such as the interface language or application configurations.
Basic Example
Here is a simple example to illustrate how the React Context API works.
jsx
In this example, UserContext.Provider
wraps the part of the component tree that needs access to the user data, and UserContext.Consumer
is used in the UserProfile
component to consume those data.
Conclusion
The React Context API is a flexible and efficient tool for managing global data and avoiding prop drilling in our applications. In the following chapters, we will delve into how to create and use contexts, as well as some advanced patterns we can employ to optimize our code and improve performance.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
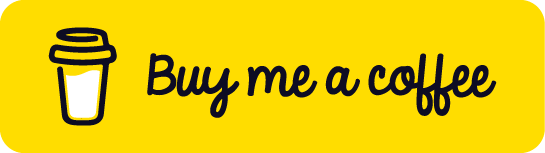
Chat with Chuck
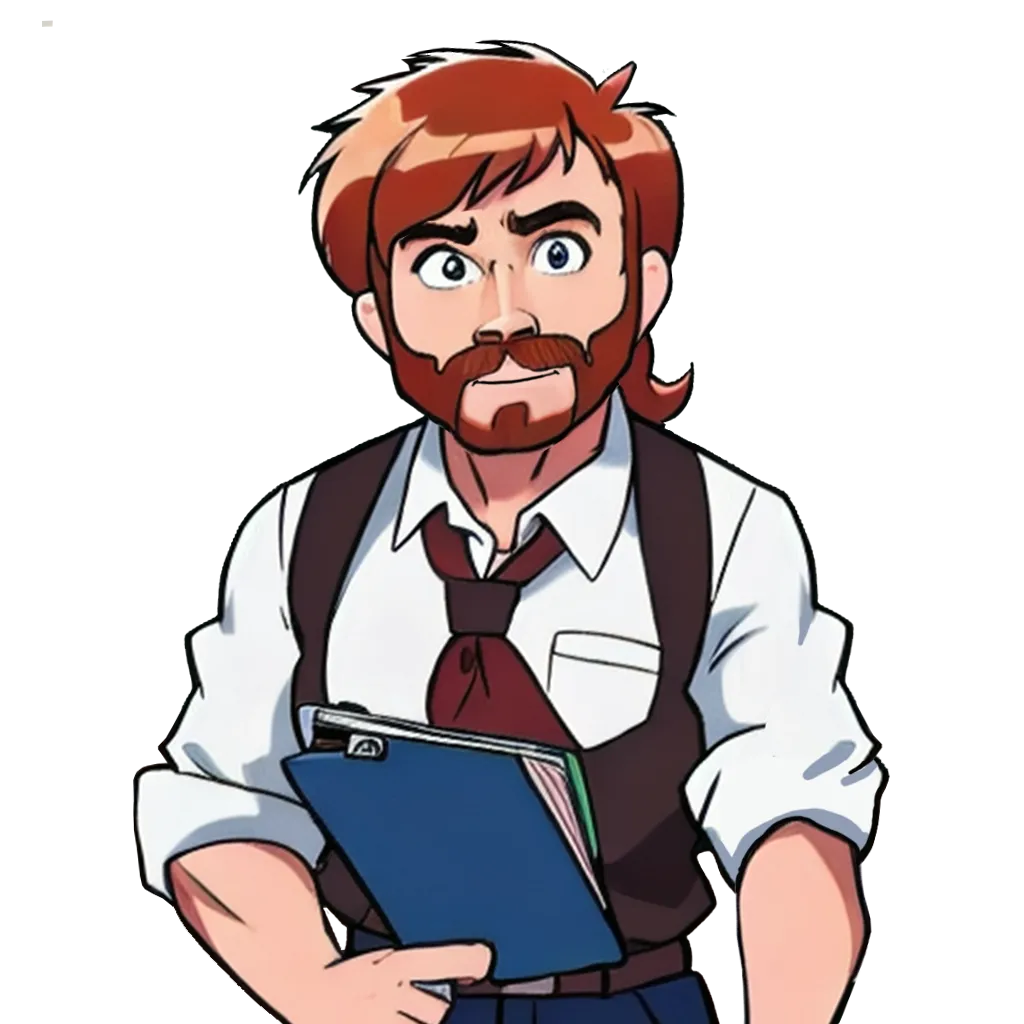
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices