React Context API
Context API and Functional Components
React's Context API integrates very efficiently with functional components, especially with the help of hooks like useContext
. Functional components are more concise and readable, and when combined with Context API, they can result in cleaner and more maintainable code. In this chapter, we will explore how to use Context API effectively in functional components.
Advantages of Using Functional Components with Context API
- Clarity and Conciseness: Functional components are shorter and clearer, making them easier to understand and maintain.
- Hooks: Hooks like
useContext
provide direct and simple access to contexts, eliminating the need for more complex patterns like render props. - Logic Reuse: With hooks, it's easy to reuse state and effect logic in different components.
Using useContext
in Functional Components
The useContext
hook allows you to access context data directly within a functional component. This eliminates the need to use the Consumer
component and simplifies the code.
Basic Example with useContext
jsx
In this example, useContext(UserContext)
is used inside the functional component UserProfile
to access and update context data.
Using useReducer
with Context API
useReducer
is useful for handling complex states in functional components, and it can be combined with Context API to provide more robust state management.
Example with useReducer
jsx
In this example, useReducer
is combined with Context API to handle a more complex user state.
Memoization with useMemo
and useCallback
To prevent unnecessary re-renders and improve performance, you can use useMemo
and useCallback
to memoize values and functions.
Memoization Example
jsx
In this example, useMemo
and useCallback
are used to memoize the context value and the update function, respectively, avoiding unnecessary re-renders.
Custom Hooks for Context
You can create custom hooks to encapsulate context logic, making your code more modular and reusable.
Custom Hook Example
jsx
In this example, the custom hook useUser
encapsulates the logic for accessing the user context, and the UserProvider
provides the context to child components.
[Placeholder: Image showing a diagram of functional components using useContext
, custom hooks, and useReducer
, highlighting modularity and simplicity]
Conclusion
Using Context API with functional components and hooks in React allows you to write cleaner, more modular, and efficient code. From the basic use of useContext
to more advanced patterns like useReducer
and custom hooks, these techniques will help you handle global states more effectively in your React applications. In the next chapter, we will compare using Context API in functional components versus class components.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
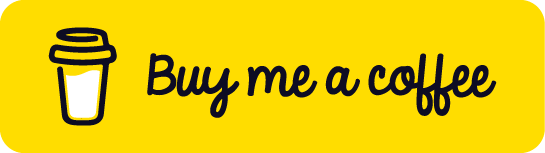
Chat with Chuck
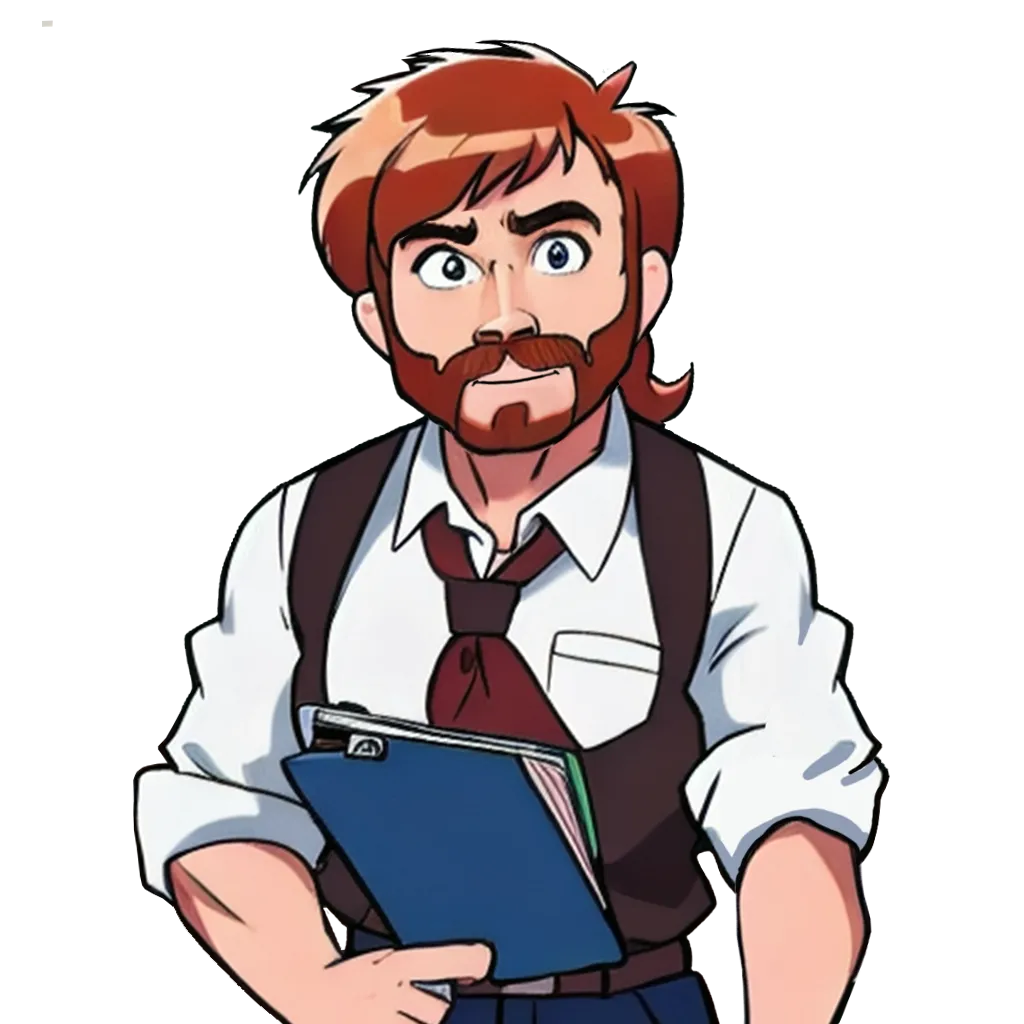
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices