Async/Await in JavaScript
Fundamentals of Asynchronous Programming
Before diving into Async/Await, it is crucial to understand the basics of asynchronous programming in JavaScript. Asynchronous programming is a technique that allows a unit of work to operate independently from the main flow of the program, enabling other operations to continue executing.
Concept of Concurrency and Parallelism
- Concurrency: Concurrency refers to the ability to handle multiple operations at the same time progressing.
- Parallelism: Parallelism involves executing multiple operations at the same time.
The Event Loop in JavaScript
The event loop is a fundamental architecture on which JavaScript relies to handle asynchronous operations. It works as follows:
- Call Stack: Where synchronous code is executed.
- Queue: Where asynchronous operations put their callbacks once they are ready.
- Event Loop: Monitors the Call Stack and the Queue. If the Call Stack is empty, it pushes elements from the Queue to the Call Stack for execution.
Callbacks
Callbacks are functions that are passed as arguments to other functions and are executed after an asynchronous operation has completed. Although callbacks are powerful, they can lead to the well-known problem of "Callback Hell," making the code difficult to read and maintain.
Callback Example
javascript
Promises
Promises were introduced to make the management of asynchronous programming more manageable. A promise is an object that represents the eventual state of an asynchronous operation: pending, fulfilled, or rejected.
Promises Example
javascript
Advantages of Promises over Callbacks
- Chaining: Promises allow chaining multiple asynchronous operations more manageably.
- Error Handling: It is easier to handle errors using
catch
. - Readability: Code with promises can be read more sequentially than nesting callbacks.
Conclusion
Understanding the basics of asynchronous programming is essential to mastering Async/Await. In this chapter, we have reviewed basic concepts such as the event loop, the use of callbacks, and the introduction of promises. In the following chapters, we will see how promises and callbacks evolved into Async/Await to offer a simpler and more efficient way of handling asynchrony in JavaScript.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
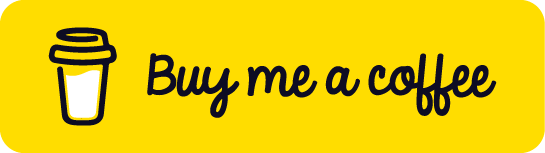
Chat with Chuck
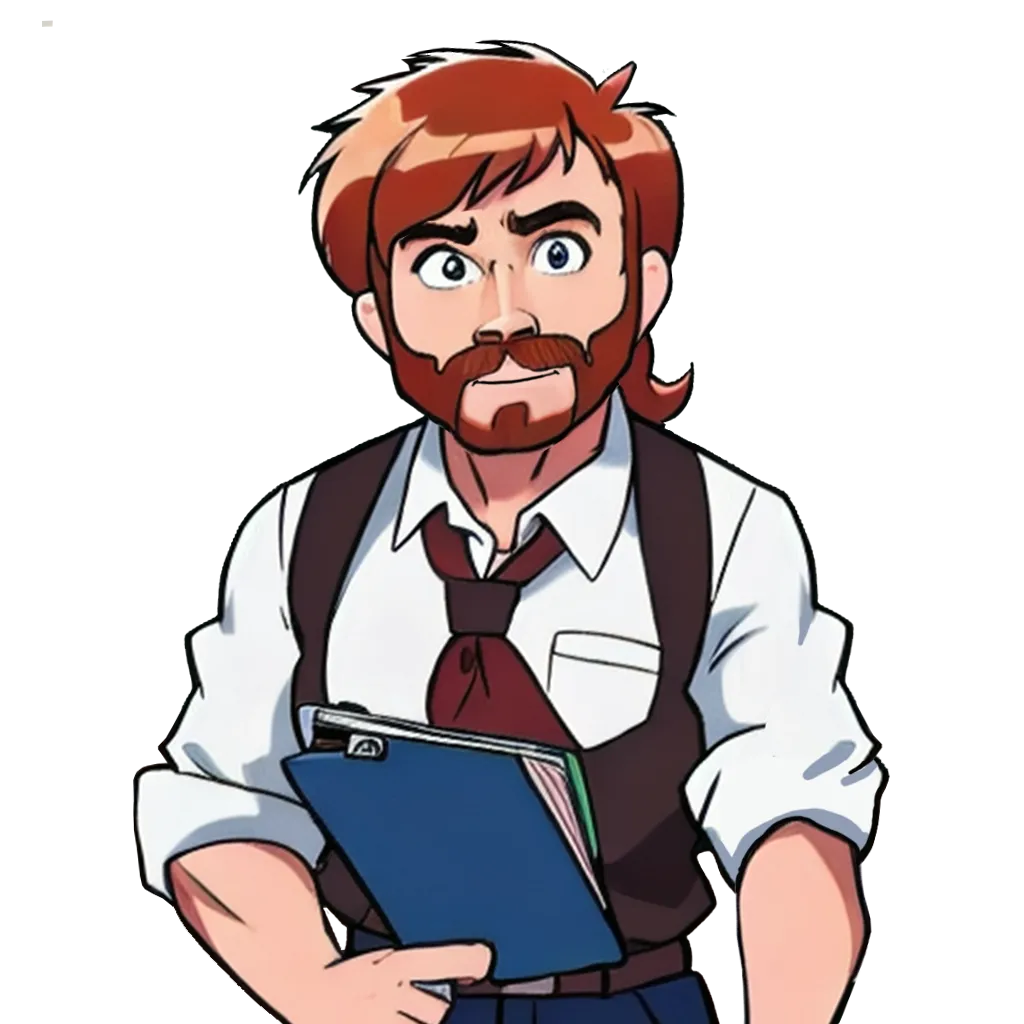
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps