Async/Await in JavaScript
Promises in JavaScript
In this chapter, we will dive deep into promises, an essential feature of JavaScript that has revolutionized the way asynchronous programming is handled.
What is a Promise?
A promise is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. A promise can be in one of three states:
- Pending: The operation has not yet completed.
- Fulfilled: The operation was completed successfully.
- Rejected: The operation failed.
Creating a Promise
To create a promise, you use the Promise
constructor, which takes a function with two parameters: resolve
and reject
. This function is executed immediately, with resolve
and reject
controlling the promise's state.
Example of Creating a Promise
javascript
Working with Promises
The then
method is used to handle a fulfilled promise, while catch
is used to handle a rejected promise.
Example of Using Then and Catch
javascript
Chaining Promises
One of the powerful features of promises is their ability to chain them. This allows for sequential operations to be performed in a more readable manner.
Example of Chaining Promises
javascript
Useful Promise Methods
- Promise.all: Runs multiple promises in parallel and resolves when all promises are resolved, or rejects if any promise is rejected.
javascript
- Promise.race: Resolves or rejects as soon as one of the given promises resolves or rejects.
javascript
Conclusion
Promises have significantly improved the way we write asynchronous code in JavaScript, making it easier to manage the asynchronous workflow. By understanding how to create, handle, and chain promises, we are laying the groundwork for understanding how Async/Await builds on these structures to offer even more simplicity and clarity to asynchronous code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
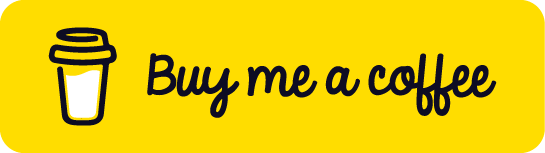
Chat with Chuck
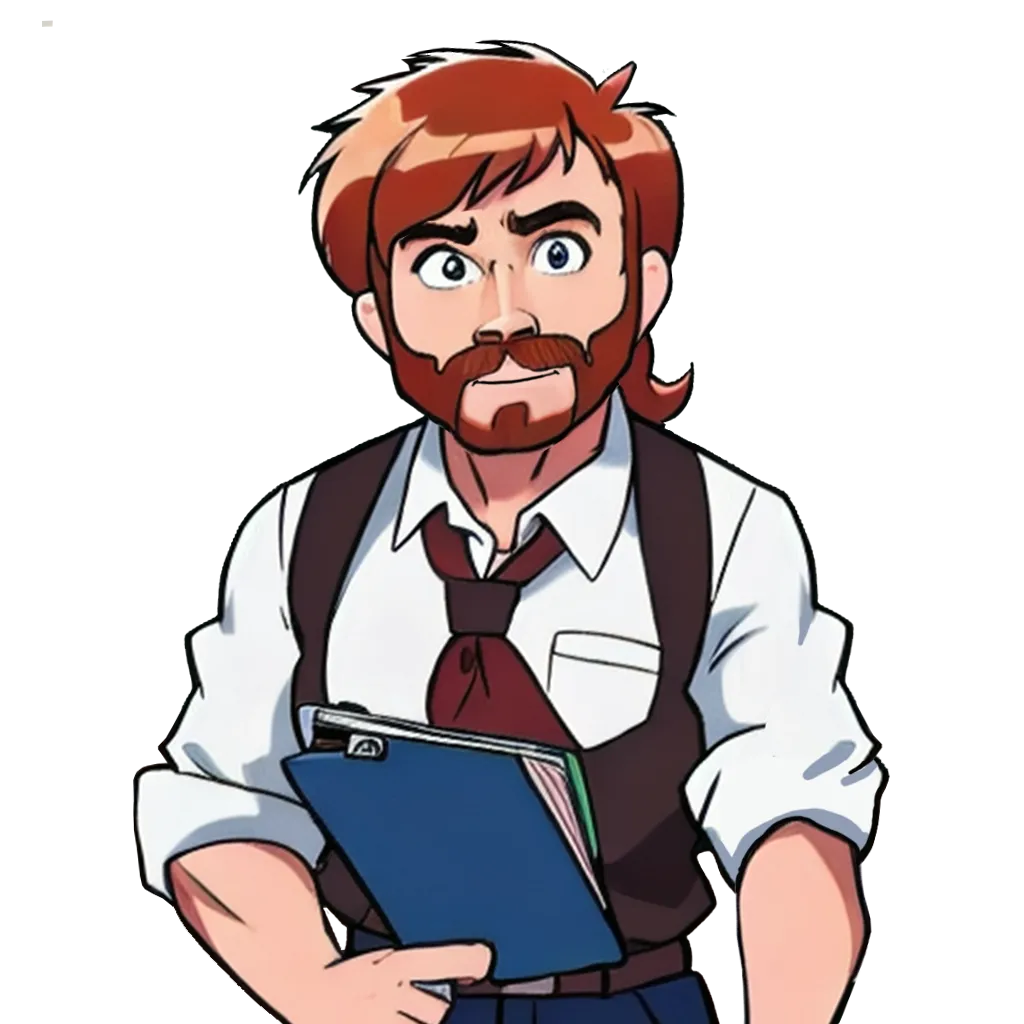
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps