Async/Await in JavaScript
Common Use Cases and Best Practices
Async/Await is a powerful tool in JavaScript, and understanding its use in common cases and following best practices, you can write more robust and maintainable code. In this section, we will explore some common use cases of Async/Await and the best practices to follow.
Common Use Cases
1. Sequential and Parallel API Calls
A common task is to make multiple API calls, either in sequence or in parallel.
Sequential Calls:
javascript
Parallel Calls:
javascript
2. Database Access
Accessing and manipulating data in a database asynchronously is another common use.
javascript
3. File Processing
Reading and writing files asynchronously is crucial for I/O operations in Node.js.
javascript
Best Practices
1. Code Structure and Readability
Keep the code clean and readable by following naming conventions and organizing the code in well-defined functions.
Example of Readable Code:
javascript
2. Proper Error Handling
Incorporate error handling using try/catch
and provide clear and detailed messages.
javascript
3. Avoid Excessive Nesting
While Async/Await makes asynchronous handling easier, avoid nesting operations when possible. Use helper functions to keep the program flow clean.
javascript
4. Reasonable Use of Promise.all
When appropriate, use Promise.all
to execute operations in parallel, but be mindful of system limitations and error handling.
javascript
5. Avoid Using await
in Loops
await
inside loops can lead to inefficiencies. Use Promise.all
to handle promises in a loop.
javascript
Conclusion
Understanding and applying common use cases along with best practices for handling Async/Await in JavaScript will allow you to write more robust, readable, and maintainable code. By following these guidelines, you can maximize efficiency and minimize potential errors in your asynchronous applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
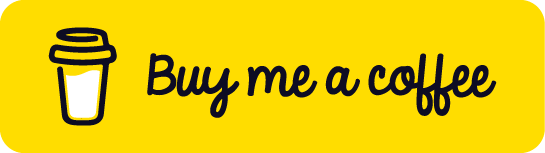
Chat with Chuck
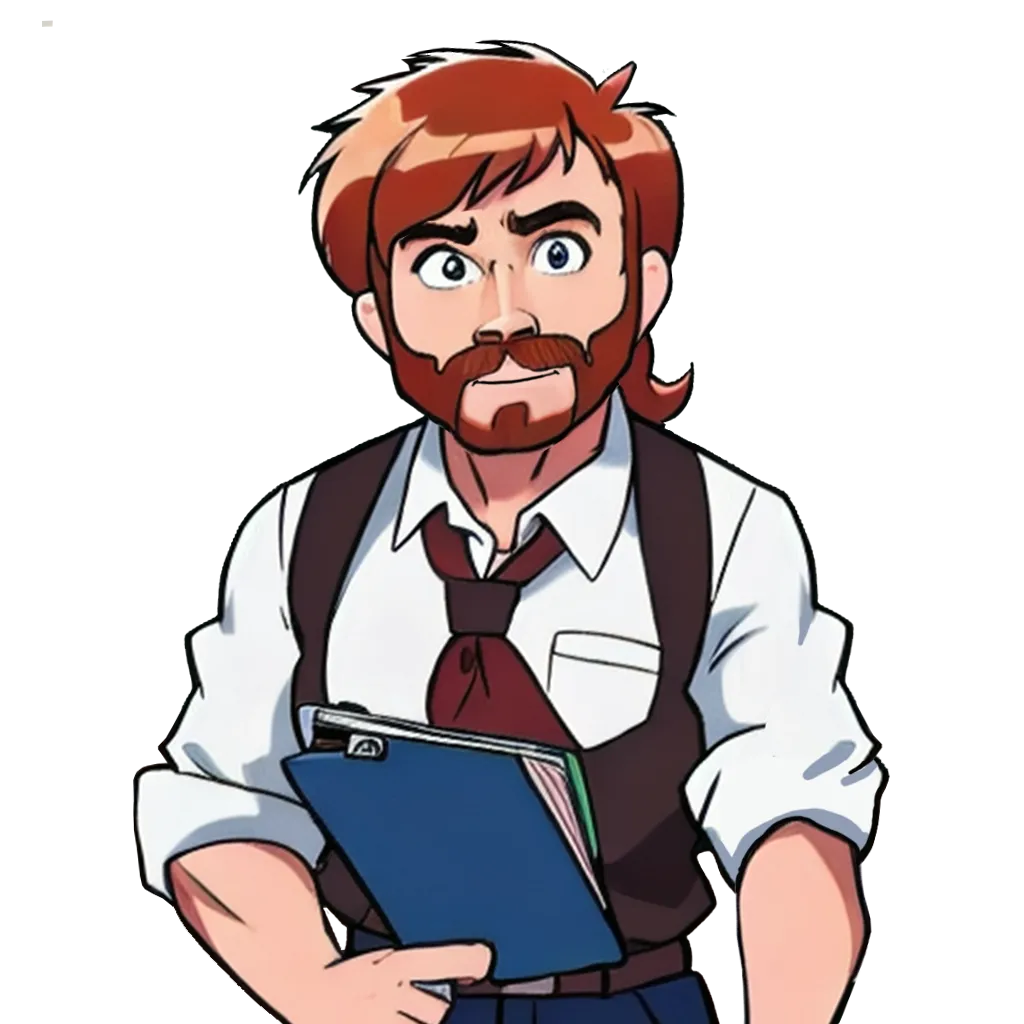
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps