Async/Await in JavaScript
Transition from Promises to Async/Await
The introduction of Async/Await in JavaScript has greatly simplified the handling of asynchronous operations, eliminating the problem of Callback Hell and making the code more readable and easier to maintain. In this section, we will explore how to transition from promises to Async/Await and the additional advantages this technique offers.
From Promises to Async/Await
The key to understanding Async/Await is to realize that both are based on promises. Async/Await is simply a more modern and readable syntax for working with promises.
Review of a Promise Example
Let's consider the promise example from the previous chapter:
javascript
This code is readable, but it could be even clearer by using Async/Await. Let's see how to do it.
Async/Await Syntax
Asynchronous Functions
To use await
, we first need to define an asynchronous function using the async
keyword.
javascript
Inside an async
function, we can use the await
keyword to wait for a promise.
Transforming the Example to Async/Await
Let's see how to transform the previous promise example to Async/Await:
javascript
Advantages of Async/Await
- Improved Readability: The syntax resembles synchronous code, making it easier to read and understand.
- Error Handling: Using
try/catch
to handle errors instead ofcatch
provides a more direct and clear way of capturing exceptions. - Simplified Maintenance: Linear code is easier to maintain and modify.
Important Considerations
- Browser Compatibility: While most modern browsers support Async/Await, it's important to consider compatibility with older browsers or use tools like Babel to transpile the code.
- Silent Errors: Make sure to properly handle errors with
try/catch
to avoid silent errors that can be difficult to debug.
Conclusion
The transition from promises to Async/Await in JavaScript brings clarity and simplicity to managing asynchronous operations. As we progress in this course, we will see how to effectively use Async/Await and how to leverage its advantages to write cleaner and more maintainable code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
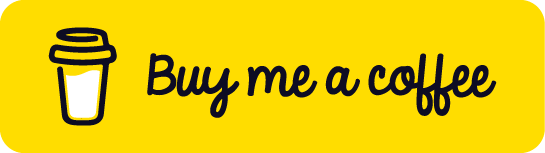
Chat with Chuck
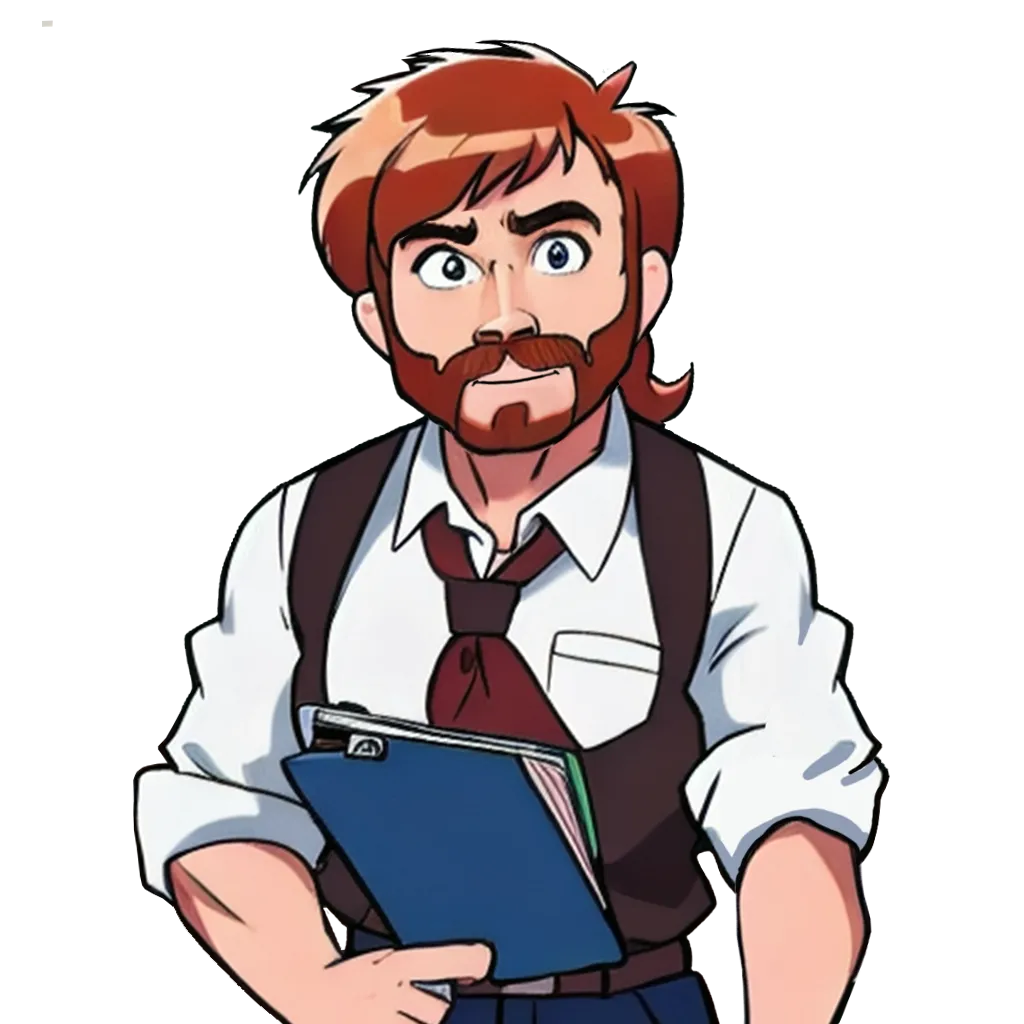
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps