Async/Await in JavaScript
The Callback Hell Problem
One of the most notable challenges in asynchronous programming with JavaScript is the problem known as "Callback Hell." This problem arises when callbacks are deeply nested due to the need to perform sequential and asynchronous operations.
What is Callback Hell?
"Callback Hell" refers to a situation where multiple nested callbacks make the code difficult to read and maintain. This pattern is common in applications that require multiple sequential asynchronous operations.
Example of Callback Hell
Let's consider a series of asynchronous operations that depend on one another:
javascript
In this example, each operation must wait for the previous one to complete before it can proceed. This style of programming creates a pyramid structure, leading to code that is hard to read and maintain.
Disadvantages of Callback Hell
- Readability: Deep nesting of callbacks makes the code hard to follow.
- Maintainability: Modifying, debugging, and updating the code becomes a challenge due to its complexity.
- Errors: It is easier to introduce and overlook errors because of the complexity and lack of clarity.
Strategies to Handle Callback Hell
There are several strategies and patterns to mitigate the issues associated with Callback Hell:
- Code Modularization: Breaking up large functions into smaller, more manageable functions.
- Use of Promises: Promises help avoid deep nesting and manage sequential operations in a clearer way.
Using Promises to Avoid Callback Hell
The previous example can be rewritten using promises:
javascript
Conclusion
Callback Hell is a common problem in asynchronous programming, but it can be managed using appropriate strategies such as code modularization and the use of promises. In the following chapters, we will see how Async/Await builds on promises to further simplify asynchronous code handling, almost entirely eliminating issues associated with Callback Hell.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
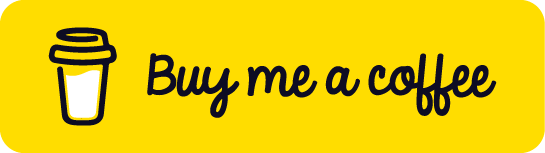
Chat with Chuck
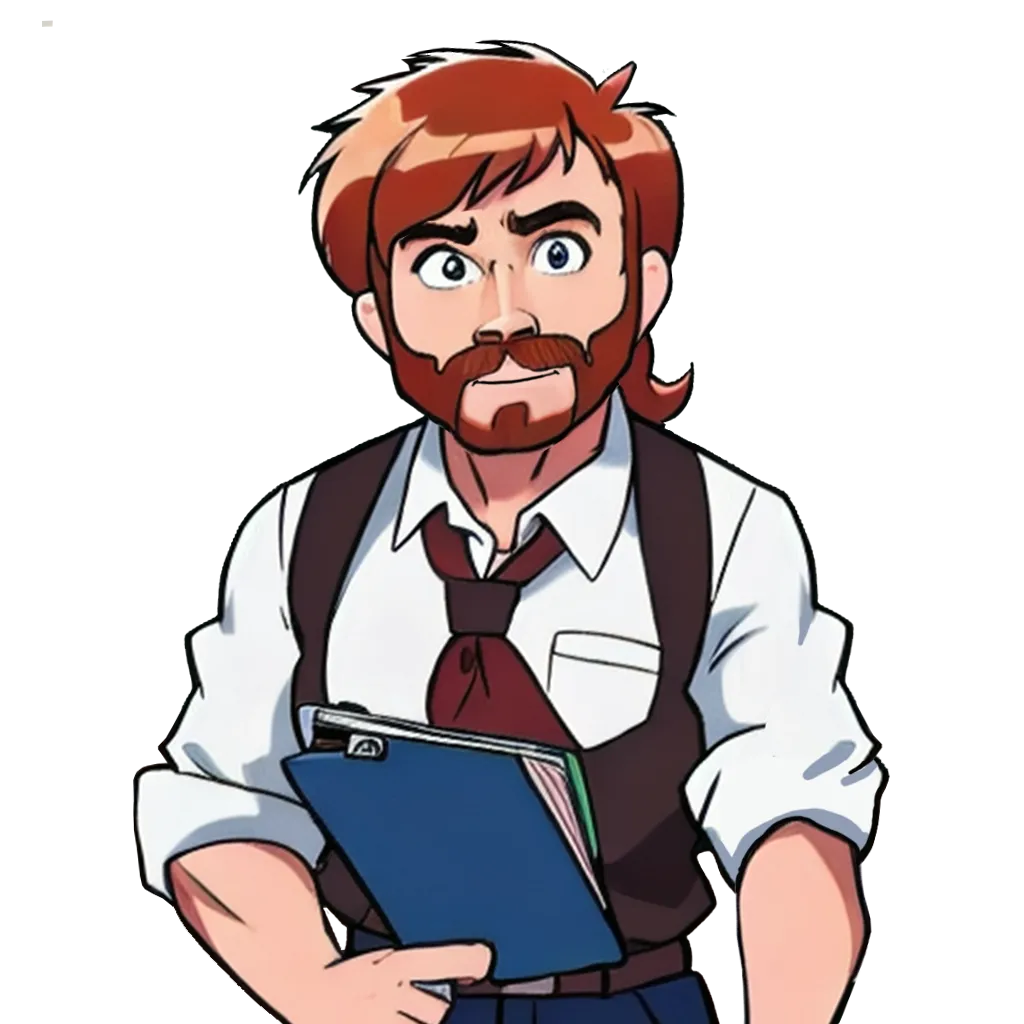
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps