Async/Await in JavaScript
Asynchronous Operations in Sequence
In many scenarios, it is necessary to execute asynchronous operations sequentially, that is, to wait for one operation to finish before starting the next one. Async/Await makes this type of control flow intuitive and easy to implement.
Sequential Execution with Async/Await
Using await
, we can write asynchronous code that executes in sequence in a way that resembles synchronous code.
Basic Example
Consider a scenario where we need to perform three operations sequentially:
- Get user data.
- Get that user's orders.
- Process a payment for one of those orders.
javascript
In this example, each await
waits for the previous promise to resolve before continuing with the next operation, ensuring that our asynchronous operations execute in the correct sequence.
Advantages of Sequential Execution with Async/Await
- Clarity: The syntax of Async/Await makes the code easier to read and understand, especially for those accustomed to synchronous programming.
- Natural Flow: The control flow is explicit and follows a logical order, which makes debugging and maintaining the code easier.
- Simplified Error Handling: As demonstrated in the previous chapter, using
try/catch
blocks in combination with Async/Await simplifies error handling in chains of asynchronous operations.
Considerations and Best Practices
- Ensure Correct Sequence: Proper use of
await
to ensure that each operation completes before starting the next. - Avoid Unnecessary Blocking: Despite the simplicity of sequential flow, it is crucial not to overuse
await
to avoid blocking operations that could run in parallel.
Handling Multiple Operations with Dependencies
Consider that each stage depends on the previous stage. If we need, for example, to get additional data at each step based on previous results:
javascript
Avoid Using await
in Loops
When using await
in a loop, you must be careful with performance and potential blocking of other operations that could run in parallel:
javascript
Instead, you could gather all the promises and then wait for all of them to resolve together using Promise.all
:
javascript
Conclusion
Using Async/Await to handle sequential asynchronous operations provides clear, linear syntax that simplifies writing and maintaining code. With these tools, you can ensure that operations that depend on each other execute in the correct sequence, keeping the code clean and efficient.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
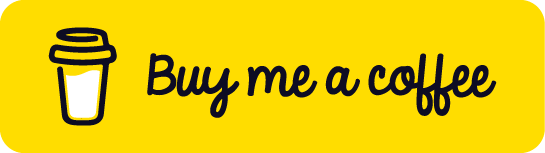
Chat with Chuck
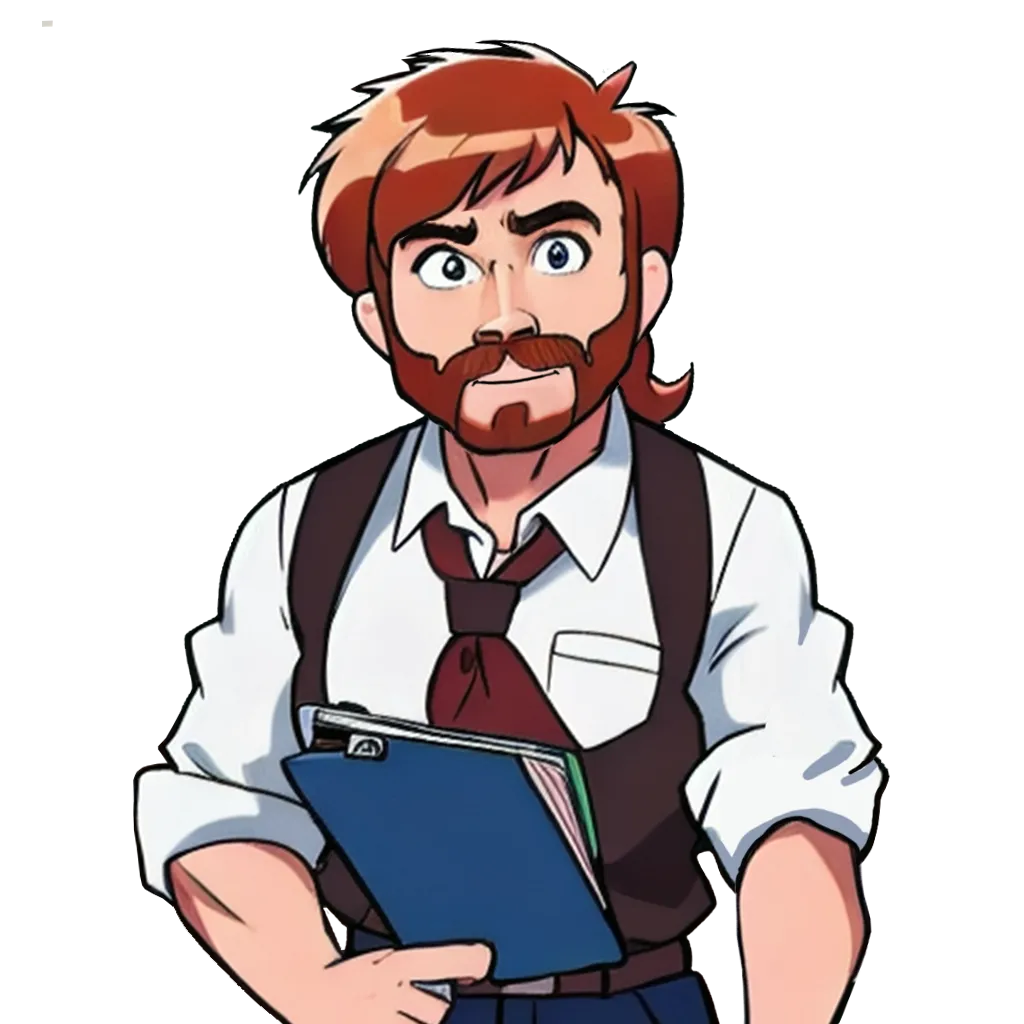
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps