Async/Await in JavaScript
Async/Await Syntax
With the advent of Async/Await, writing asynchronous code in JavaScript has been revolutionized to make it more natural and readable. In this section, we will delve into the syntax of Async/Await and how to use these constructs for effective handling of asynchronous operations.
The async
Keyword
To define an asynchronous function, the async
keyword is prefixed to the function definition. A function marked with async
always returns a promise.
javascript
This function returns a promise that resolves with the value 'Hello, world!'
.
Example: Asynchronous Function
javascript
The await
Keyword
The await
keyword is used to wait for a promise to resolve. It can only be used inside an asynchronous function. When await
is encountered, the execution of the function is paused until the promise resolves, then it returns the result.
javascript
Example: Using await
in an Asynchronous Function
javascript
Error Handling with try/catch
The try/catch
block is used to handle errors when working with Async/Await. This allows for more direct and clear error capturing.
javascript
Complete Example: Simulating a Process
Let's look at a complete example where we simulate obtaining a user, their orders, and processing a payment, using Async/Await:
javascript
Advantages and Considerations of Async/Await
- Readability and Maintenance: The more linear and sequential syntax makes the code easier to read and maintain.
- Simple Error Handling: Using
try/catch
makes error capturing and handling clearer. - Compatibility: It is essential to ensure that the runtime environment supports Async/Await, or use transpiling tools like Babel to ensure compatibility with older browsers.
Conclusion
Mastering the syntax of Async/Await is a crucial step for writing effective and readable asynchronous code in JavaScript. In the upcoming chapters, we will explore how to handle errors with Async/Await, perform asynchronous operations sequentially and in parallel, and much more.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
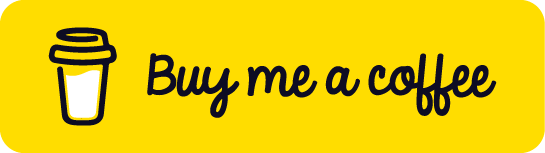
Chat with Chuck
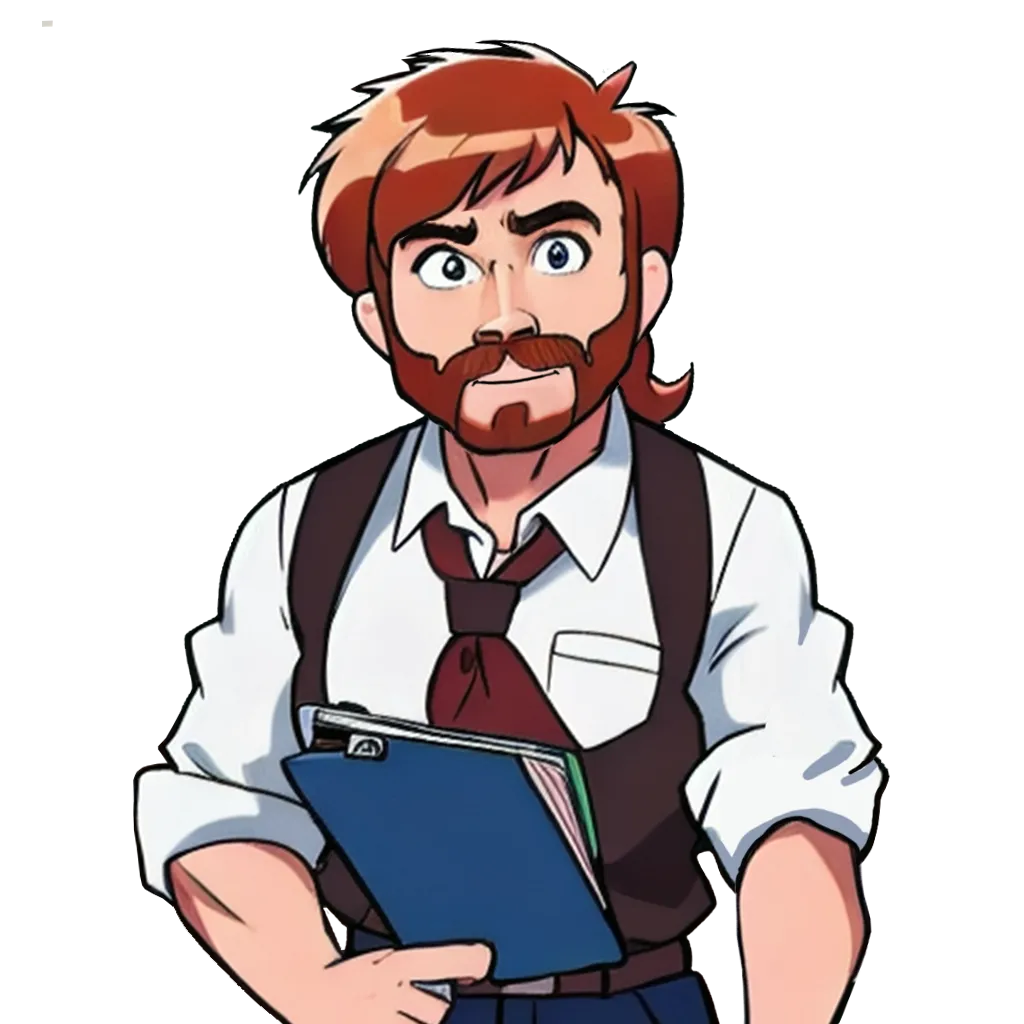
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps