Async/Await in JavaScript
Integration with Asynchronous Libraries
In modern JavaScript development, it is common to work with various libraries that offer asynchronous capabilities. Integrating Async/Await with these libraries can make handling asynchronous operations more efficient and maintainable. This chapter will explore how to use Async/Await in combination with some of the most popular asynchronous libraries.
Integration with Axios
Axios is a popular library for making HTTP requests. Although it is based on promises, using Async/Await with Axios improves code readability.
Example with Axios
javascript
Integration with Mongoose
Mongoose is a library for working with MongoDB in Node.js. It offers asynchronous methods based on promises that can be easily managed with Async/Await.
Example with Mongoose
javascript
Integration with Sequelize
Sequelize is an ORM for Node.js that supports multiple SQL databases. Handling asynchronous models and queries with Async/Await can improve the readability of database operations.
Example with Sequelize
javascript
Integration with Firebase
Firebase is a platform for web and mobile application development that provides backend services such as real-time databases and authentication. Here is how to use Async/Await with Firebase.
Example with Firebase
javascript
Considerations for Integrating Asynchronous Libraries
- Documentation: Review the library documentation to understand how it handles asynchronous operations and promises.
- Error Handling: Make sure to handle errors properly. Use
try/catch
blocks to capture errors and provide informative messages. - Performance: Some libraries may perform better with parallel operations. Evaluate when to use
Promise.all
to optimize execution time.
Conclusion
Integrating Async/Await with asynchronous libraries can enhance the readability and maintainability of your JavaScript code, simplifying the handling of asynchronous operations in popular libraries like Axios, Mongoose, Sequelize, and Firebase. Use these techniques to write clearer and more efficient code in your applications.
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps
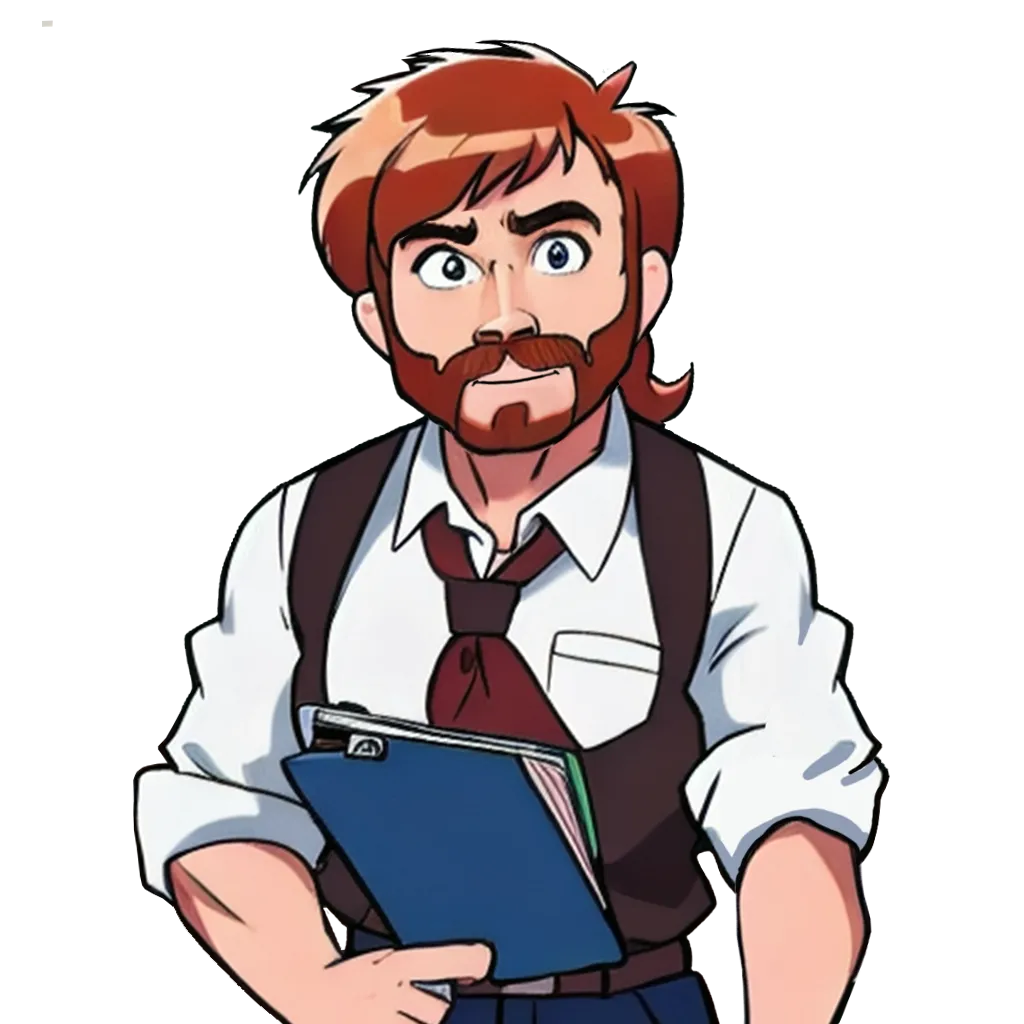