Async/Await in JavaScript
Parallel Asynchronous Operations
In many scenarios, it is beneficial to execute multiple asynchronous operations in parallel to improve efficiency and reduce the total execution time. JavaScript provides various techniques to manage asynchronous operations in parallel using Async/Await and other promise utilities like Promise.all
.
Parallel Execution with Promise.all
Promise.all
allows you to execute multiple promises in parallel and wait until all of them have resolved or one has been rejected. This is useful when operations are independent and do not rely on each other.
Basic Example with Promise.all
Suppose we need to fetch data from several services at once:
javascript
In this example, Promise.all
executes fetchData1
, fetchData2
, and fetchData3
in parallel. The code will wait for all promises to resolve before continuing.
Advantages of Parallel Execution
- Efficiency: Reduces total wait time by executing independent operations simultaneously.
- Simplicity:
Promise.all
simplifies managing parallel operations, making the code cleaner and easier to follow.
Use of Promise.race
Promise.race
is another utility that allows you to wait until one of the promises in an array resolves or is rejected. This can be useful for operations where the first response is important or to implement timeouts.
Example of Promise.race
javascript
In this example, Promise.race
is used to implement a time limit for fetching data. If the data is not fetched within 2000 ms, a "Timeout exceeded" error is thrown.
Considerations for Parallel Execution
- Independence: Ensure that the operations you execute in parallel are truly independent of each other. If an operation depends on the result of another, it should be executed sequentially.
- Error Handling: When using
Promise.all
, if any promise is rejected, all other promises are automatically cancelled. Make sure to handle errors appropriately. - System Resources: Running many operations in parallel can consume a lot of system resources. Control the number of promises to avoid overloading the system.
Complete Example: Fetching and Processing Data in Parallel
Let's see a complete example where we fetch data from multiple sources and process the results in parallel:
javascript
Conclusion
Handling asynchronous operations in parallel using Async/Await and Promise.all
improves efficiency and code clarity. Make sure to understand when and how to use these techniques to maximize performance without introducing concurrency issues or overloading the system.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
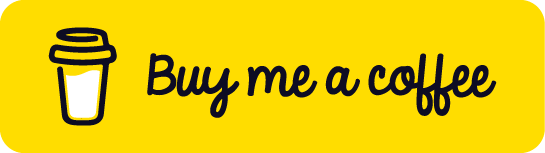
Chat with Chuck
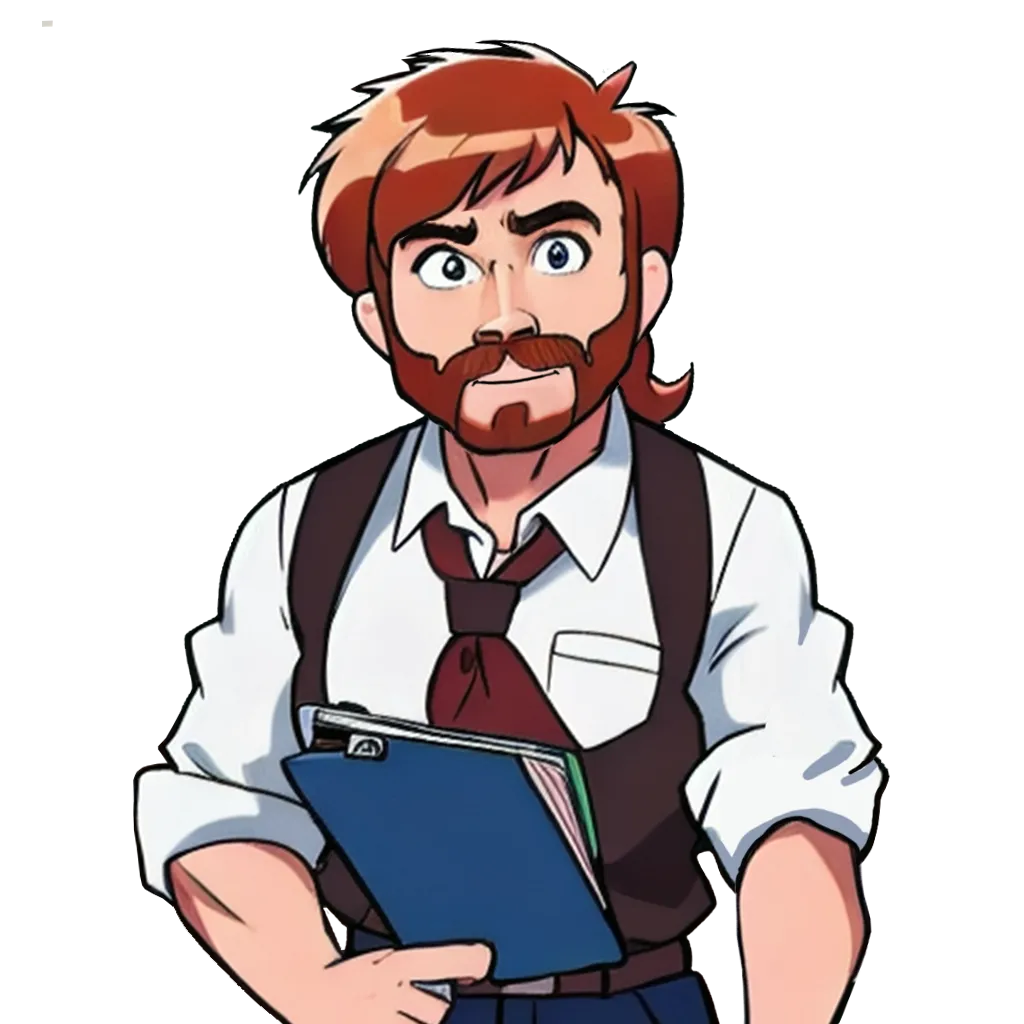
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps