Async/Await in JavaScript
Debugging in Asynchronous Code
Debugging asynchronous code can be challenging due to the non-linear nature of this type of execution. However, with some tools and strategies, it is possible to make debugging asynchronous code with Async/Await more manageable and efficient.
Debugging Tools and Techniques
1. Using console.log
Using console.log
is a basic but effective technique to follow the execution flow of your asynchronous code. You can add messages before and after asynchronous operations.
javascript
2. Using the Browser Debugger
Modern browsers like Chrome and Firefox have built-in developer tools that allow pausing at specific points and examining the state of the application.
- Breakpoints: You can set breakpoints in your code by clicking on the line number in the Sources panel.
- Conditional breakpoints: These allow you to pause execution when a specific condition is met.
3. Debugging in Node.js
In Node.js, you can use --inspect
to run your application in debug mode and connect a debugging client like Chrome DevTools.
sh
Error Handling with try/catch
Ensure to wrap your asynchronous operations in try/catch
blocks to catch errors. This not only helps in proper error handling but also facilitates debugging by providing clear error messages.
javascript
Using async
and await
in Strict Mode
Ensure your code is in strict mode to avoid silent errors. You can enable strict mode at the start of your script or function:
javascript
Analyzing Stack Traces
When an exception is thrown, the stack trace provides detailed information about where and why the exception occurred. Examine the stack trace in the console to identify the root cause of the error.
Complete Example: Step-by-Step Debugging
javascript
In this example, console.log
statements are strategically placed to provide a clear trace of the execution flow and to help identify where errors might arise.
Third-Party Tools
There are third-party tools that can enhance the debugging experience:
- VS Code Debugger: Offers an integrated debugging experience directly in Visual Studio Code.
- LogRocket: A logging tool that allows you to record user sessions and replay them for better understanding of real-time errors.
Conclusion
Debugging asynchronous code can be complex, but using the right tools and techniques, it is possible to identify and resolve errors effectively. Take advantage of breakpoints, strategic use of console.log
, and available development tools to keep your code clean and error-free.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
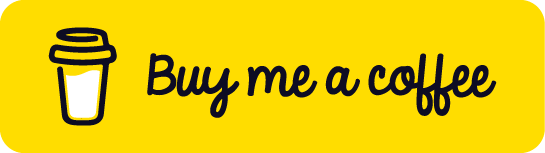
Chat with Chuck
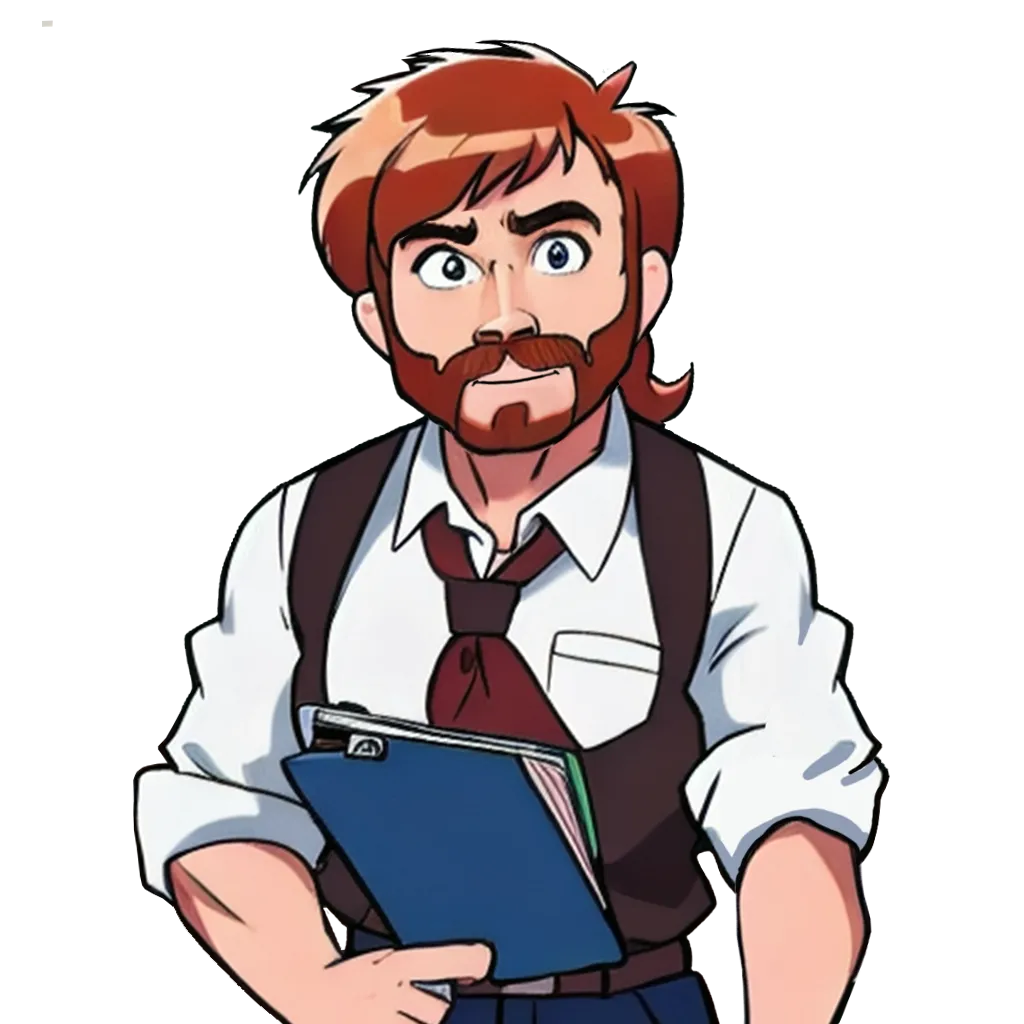
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps