Async/Await in JavaScript
Introduction to Async/Await in JavaScript
Welcome to the course "Async/Await in JavaScript." In this section, we will begin our exploration of Async/Await, fundamentally important tools for asynchronous programming in JavaScript.
What is Asynchronous Programming?
Asynchronous programming allows your code to perform other tasks while waiting for responses from long-duration operations, such as API calls, file reading, or database interactions. Instead of blocking the main thread until the operation finishes, the program continues execution, and the asynchronous operation notifies the code when it is done.
Problems in Traditional Asynchronous Programming
Before the introduction of Async/Await, JavaScript developers used callbacks
and promises
to handle asynchronous programming. Although effective, these techniques often result in complicated and hard-to-follow code.
What are Async and Await?
Async/Await
are constructs in JavaScript that simplify the writing of asynchronous code. Async
is a keyword placed before a function definition to indicate that the function is asynchronous and may contain the await
keyword. Await
is used within an asynchronous function to wait for a promise to resolve.
Advantages of Async/Await
- Readability: Code written with
Async/Await
resembles synchronous code, making it easier to read and write. - Maintainability: Asynchronous code becomes a linear sequence of instructions, improving maintainability.
- Error Handling: Using
try/catch
, handling errors in asynchronous functions becomes simpler and clearer.
Basic Example of Async/Await
Below is an example showing how async
and await
can make asynchronous code more manageable. In this example, we simulate a function that waits for an "API" response.
javascript
In this code, fetch
is an asynchronous function that returns a promise. We use await
to wait for the promise to resolve and then process the response. If an error occurs, we handle it with a try/catch
block.
Conclusion
The introduction of Async/Await has transformed how asynchronous programming is handled in JavaScript, providing a clearer and more effective way to write and maintain asynchronous code. In the upcoming chapters, we will delve deeper into the fundamental concepts of asynchronous programming and the evolution of techniques in JavaScript, preparing to use Async/Await to its fullest potential.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
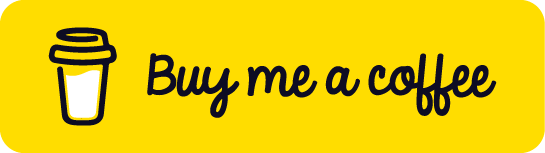
Chat with Chuck
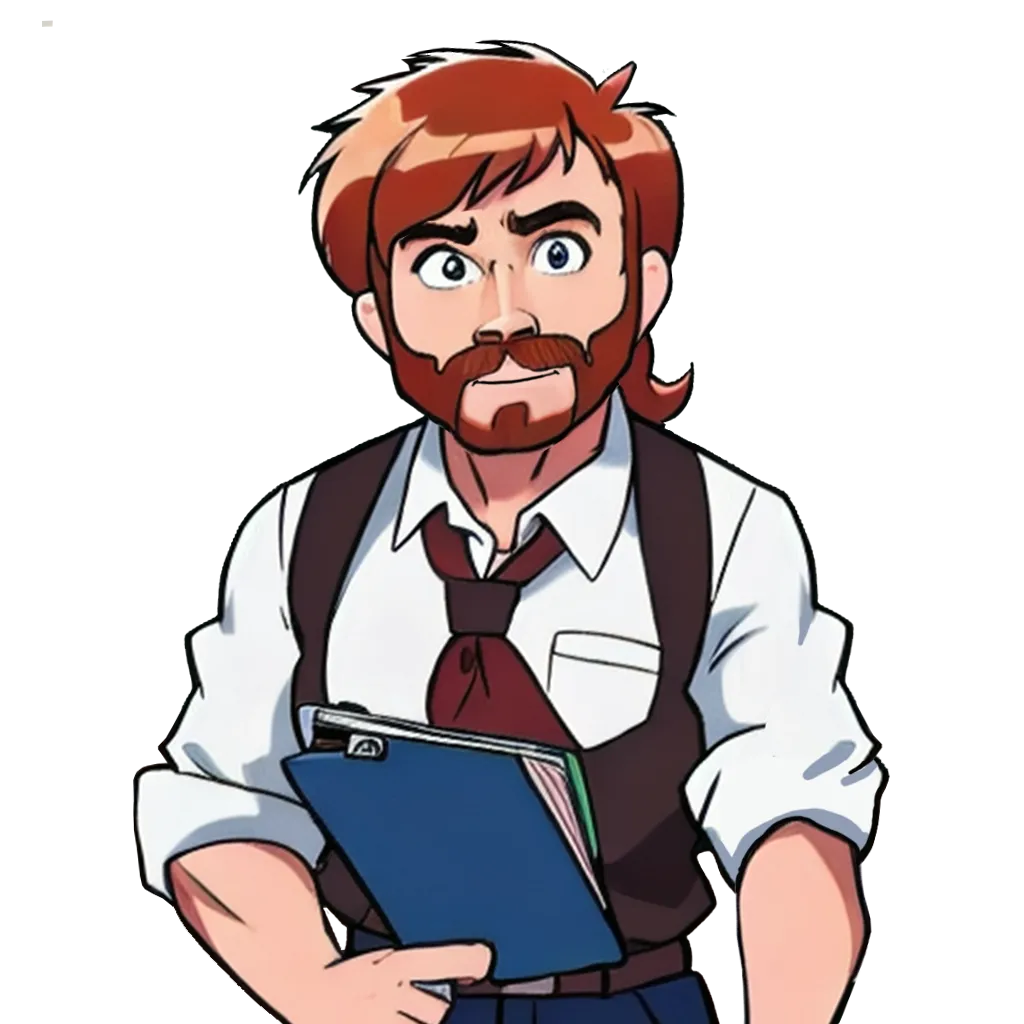
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps