Async/Await in JavaScript
Conclusions and Next Steps
Throughout this course, we have explored Async/Await in JavaScript in depth, highlighting its relevance and applicability in asynchronous programming. From the introduction to the comparison with other techniques, we have seen how Async/Await can transform the way we handle asynchronous operations, making our code more readable, maintainable, and efficient.
Summary of Key Points
- Introduction to Async/Await: Async/Await offers a cleaner and more understandable syntax for handling asynchrony compared to callbacks and promises.
- Fundamentals of Asynchronous Programming: Understanding the JavaScript Event Loop and how asynchronous operations are handled is crucial to leveraging Async/Await to its fullest potential.
- Promises in JavaScript: Promises are the foundation upon which Async/Await is built, providing better handling and chaining of asynchronous operations.
- The Callback Hell Problem: Async/Await is an effective solution to avoid excessive nesting and complexity of callbacks.
- Transitioning from Promises to Async/Await: We demonstrated how to convert promise-based code to Async/Await to improve code clarity and structure.
- Async/Await Syntax: We analyzed the basic syntax and how to handle asynchronous operations sequentially and in parallel.
- Error Handling with Try/Catch: We learned how to handle errors effectively using
try/catch
blocks in asynchronous functions. - Sequential and Parallel Asynchronous Operations: We saw how to organize asynchronous operations sequentially and in parallel to optimize performance.
- Using Async/Await with Fetch API: We explored how to use Async/Await with the Fetch API to efficiently make HTTP requests.
- Integration with Asynchronous Libraries: We discovered how to integrate Async/Await with popular asynchronous libraries like Axios and Mongoose.
- Debugging Asynchronous Code: Techniques and tools for efficiently debugging asynchronous code.
Next Steps
With the knowledge acquired, you can start applying Async/Await in your projects. Here are some suggestions on how to continue your learning and practice:
1. Practical Project
Implement Async/Await in a practical project. This could be a personal project or an application you are currently working on. Replace callbacks and promises with Async/Await and observe how it improves code readability and maintainability.
2. Experiment with Complex APIs
Search for public APIs and build small projects to consume and handle data using Fetch and Async/Await. Practice complex operations, error handling, and data processing.
3. Contribute to Open Source Projects
Explore open source projects on GitHub and find opportunities to refactor existing code using Async/Await. This not only improves your skills but also contributes to the community.
4. Perfect Error Handling
In larger and more complex projects, practice error handling and ensure that your code is robust. Learn to use monitoring and logging tools to track and resolve issues in production.
5. Deepen Knowledge of Libraries and Frameworks
Explore how Async/Await integrates with different libraries and frameworks such as React, Vue.js, and Next.js. Practice complex implementations and make sure to understand how to handle state and side effects in asynchronous environments.
Additional Resources
-
Official JavaScript Documentation:
-
Articles and Blogs:
- Technical articles on Medium, Dev.to, and other developer blogs.
-
Courses and Tutorials:
- Platforms like Udemy, Coursera, and FreeCodeCamp offer advanced JavaScript courses covering Async/Await.
-
Communities and Forums:
- Participate in communities on Stack Overflow, Reddit, and developer forums to share knowledge and solve doubts.
Conclusion
Async/Await has transformed the way we handle asynchronous operations in JavaScript, bringing clarity and simplicity. With the proper understanding and practice, you can write more efficient and maintainable code, significantly improving your projects and skills as a developer. Now it's your turn to apply and experiment with everything you have learned!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
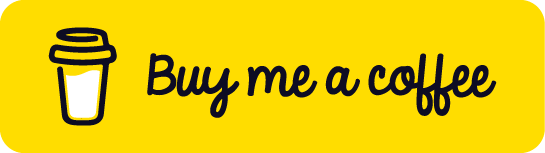
Chat with Chuck
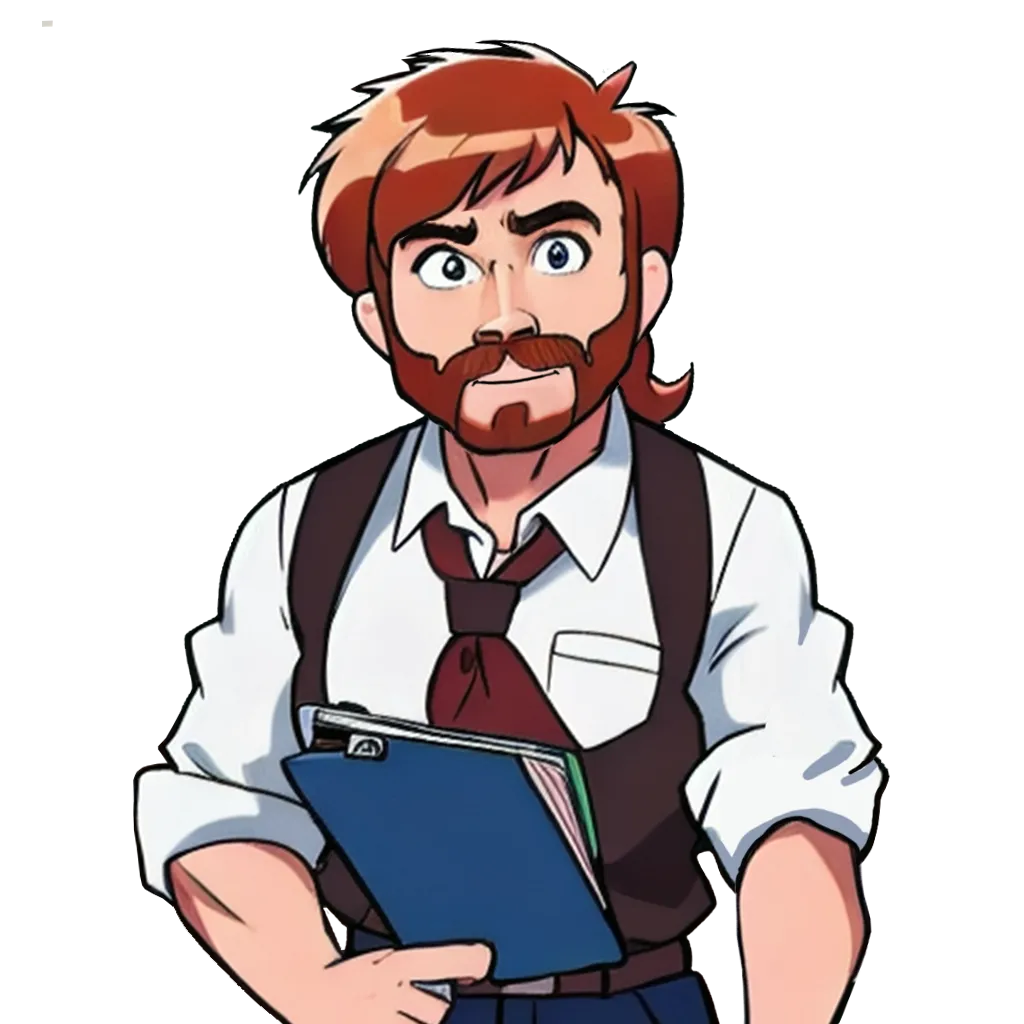
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps