Async/Await in JavaScript
Error Handling with Try/Catch
Handling errors is a crucial part of developing robust software. In the context of Async/Await, error handling becomes simpler and more straightforward thanks to the use of try/catch
blocks.
Errors in Asynchronous Code
Traditionally, handling errors in asynchronous code can be challenging, especially when using callbacks or even promises. However, Async/Await offers a clearer and more understandable syntax for handling errors.
Using try/catch
The try/catch
block allows you to encapsulate code that might throw exceptions within the try
block, and handle any exceptions thrown within the catch
block.
Basic Example with try/catch
javascript
In this example, if the fetch
call fails or the response is not successful (respuesta.ok
is false
), an error is thrown and caught in the catch
block.
Error Handling in Nested Functions
You might need to handle errors at multiple levels of functions, especially if you have several asynchronous operations depending on each other.
Example of Error Handling in Nested Functions
Consider the following flow of asynchronous operations:
javascript
This flow handles errors at each level of the chain of asynchronous operations. If any function throws an error, it will be caught in the catch
block of procesarFlujoDeDatos
.
Best Practice Recommendations
- Precise Try/Catch Blocks: Place
try/catch
blocks only around code that might throw exceptions, not around the entire function, to avoid catching unwanted errors. - Intentionally Throw Errors: If a specific condition is not met, intentionally throw an error to be handled.
- Custom Errors: Create custom errors to provide more context and details about what went wrong.
javascript
Conclusion
Error handling with try/catch
in the context of Async/Await brings clarity and simplicity to asynchronous code. It allows you to capture and handle errors more structurally, ensuring that problems are identified and managed appropriately, thereby improving the robustness and maintainability of your code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
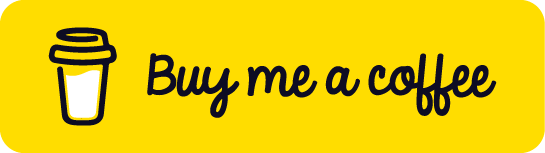
Chat with Chuck
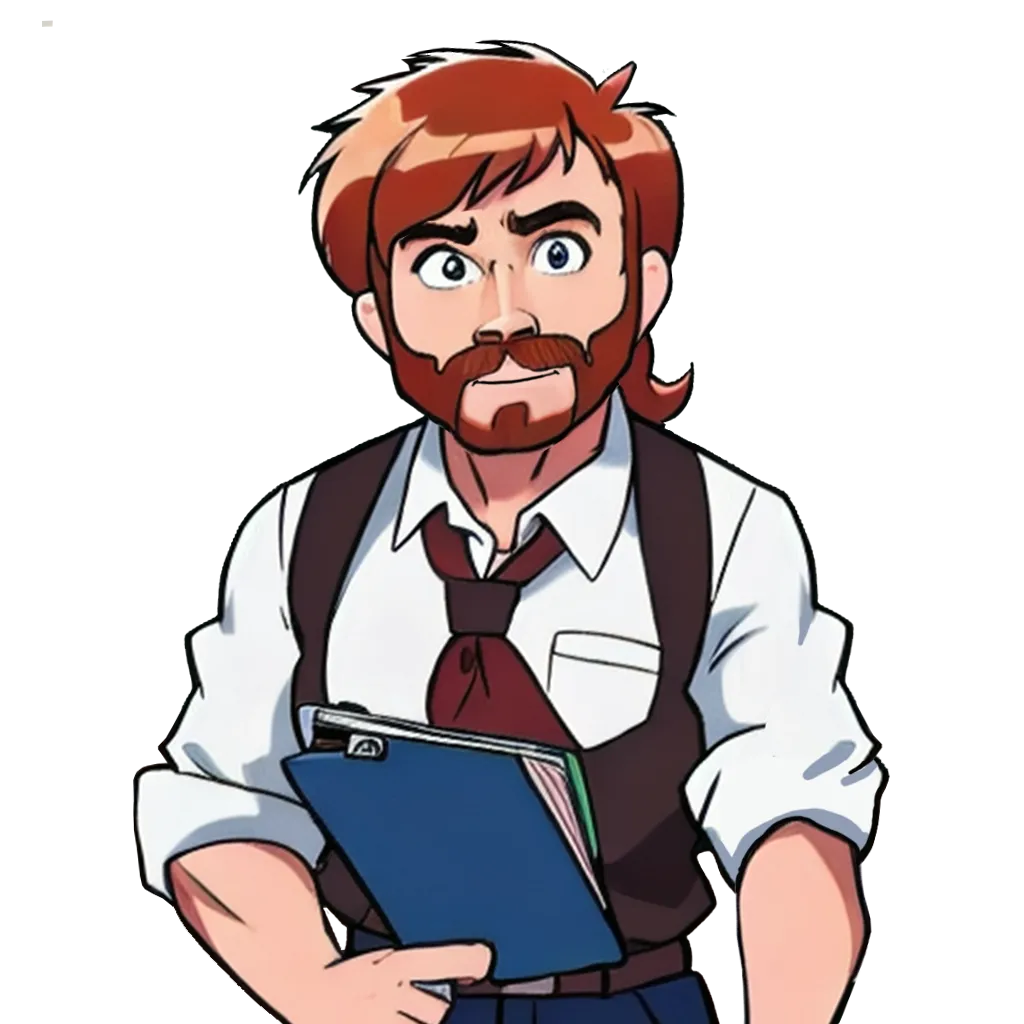
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps