Async/Await in JavaScript
Using Async/Await with the Fetch API
Fetch is a modern API that allows making HTTP requests in JavaScript in a simple and efficient way. Combining Fetch with Async/Await simplifies even further the execution of asynchronous operations like fetching data from a server.
Introduction to Fetch
Fetch is a function that returns a promise and is used to make HTTP requests. You can use it to fetch resources from a server and handle the responses effectively.
Basic Fetch Example
javascript
While this code uses promises, we can make it even more readable with Async/Await.
Using Async/Await with Fetch
Using Async/Await with Fetch allows us to write HTTP request code that looks synchronous, improving readability.
Basic Example with Async/Await
javascript
In this example, await
is used to wait for the fetch
request promise to resolve, and then to wait for the promise returned by response.json()
, allowing the request to be handled sequentially and clearly.
Error Handling
Error handling is simple with try/catch
. If the request fails or the response is unsuccessful, an error is thrown that can be caught and handled.
Error Handling Example
javascript
This example shows how to properly handle errors by checking the response status before attempting to parse it.
Sending Data with Fetch and Async/Await
Fetch can also be used to send data to the server using methods like POST
, PUT
, DELETE
, etc.
Sending Data Example
javascript
In this example, we use fetch
to send data to the server with the POST
method, handling the response and any errors that may occur.
Important Considerations
- CORS: When making cross-origin requests, make sure the server allows CORS (Cross-Origin Resource Sharing).
- Response Status Handling: Always check the response status to appropriately handle errors and unexpected responses.
- Security: Do not include sensitive information directly in the URLs of
fetch
. Use headers and the request body appropriately.
Complete Example: CRUD with Fetch and Async/Await
javascript
Conclusion
Using Fetch with Async/Await provides a powerful and simple way to make HTTP requests and handle responses asynchronously in JavaScript. By mastering these techniques, you can build robust and efficient web applications that interact with web services in a clear and maintainable way.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
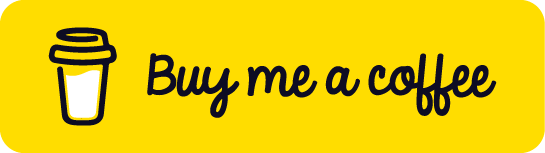
Chat with Chuck
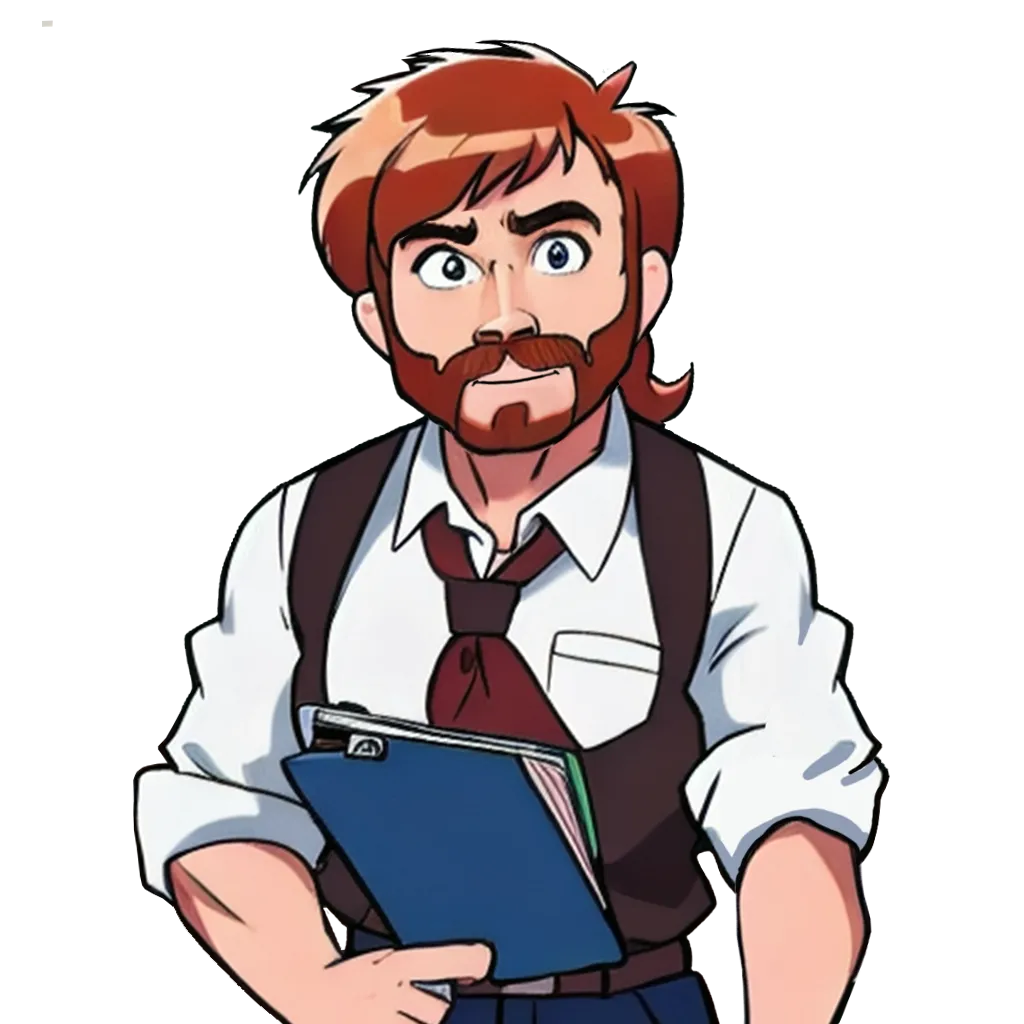
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps