Async/Await in JavaScript
Comparison with Other Asynchronous Techniques
Since the birth of JavaScript, there have been various techniques to handle asynchronous operations. In this chapter, we will compare Async/Await with other common asynchronous techniques: Callbacks and Promises, highlighting their advantages and disadvantages.
Callbacks
Callbacks were one of the first ways to handle asynchronous operations in JavaScript. They involve passing a function as an argument to another function and executing it once the asynchronous operation is completed.
Example with Callbacks
javascript
Advantages of Callbacks
- Initial Simplicity: Initially, callbacks may seem easy to understand and implement.
Disadvantages of Callbacks
- Callback Hell: When callbacks are deeply nested, the code becomes difficult to read and maintain.
- Complex Error Handling: Handling errors can be more complicated and error-prone.
Promises
Promises were a significant improvement over callbacks, providing a clearer and more manageable way to handle asynchrony.
Example with Promises
javascript
Advantages of Promises
- Chaining: Allows chaining multiple asynchronous operations in a readable manner.
- Improved Error Handling: Errors can propagate and be handled more easily with
.catch
.
Disadvantages of Promises
- Readability: Although better than callbacks, chained promises can make reading difficult in very long sequences.
- Flat Promise Trees: Linear operations may result in flatter promise trees, but still less clear than synchronous code.
Async/Await
Async/Await builds on promises and provides a more modern and readable syntax for handling asynchronous operations.
Example with Async/Await
javascript
Advantages of Async/Await
- Readability and Maintainability: The syntax resembles synchronous code, making it easier to read and maintain.
- Intuitive Error Handling: Uses
try/catch
blocks to handle errors, providing a clear control flow. - Linear Control Flow: Writing asynchronous code in a linear manner makes it easier to follow the program's flow.
Disadvantages of Async/Await
- Browser Compatibility: Some older browsers do not support Async/Await. However, this can be mitigated with transpiling using Babel.
- Controlled Blocking: Improper use of
await
in loops can result in unnecessary blocking.
Summary Comparison
Readability
- Callbacks: Poor when there are multiple levels of nesting (Callback Hell).
- Promises: Better than callbacks, but can still be complex in long chained operations.
- Async/Await: Excellent, as it resembles synchronous code and is easy to follow.
Error Handling
- Callbacks: Complex, especially in multiple operations.
- Promises: Better, with the use of
.catch
. - Async/Await: Superior, using
try/catch
to handle errors.
Control Flow
- Callbacks: Difficult to follow in deep nestings.
- Promises: Better control with
.then
and.catch
, but still can be messy. - Async/Await: Clear and linear, like synchronous code.
Context and Complexity
- Callbacks: Suitable for simple tasks, but not recommended for complex flows.
- Promises: Acceptable for moderate tasks, but can be challenging to manage in extremely complex flows.
- Async/Await: Suitable for both simple and complex tasks due to its clarity and ease of handling.
Conclusion
While callbacks and promises have been fundamental for handling asynchronous operations in JavaScript, Async/Await has brought a revolution in terms of readability and simplicity. By understanding when and how to use each of these techniques, you can write clearer, more efficient, and easier-to-maintain code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
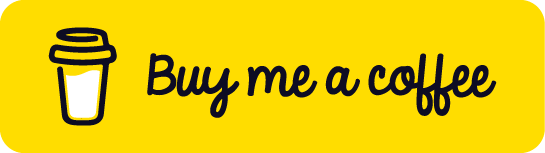
Chat with Chuck
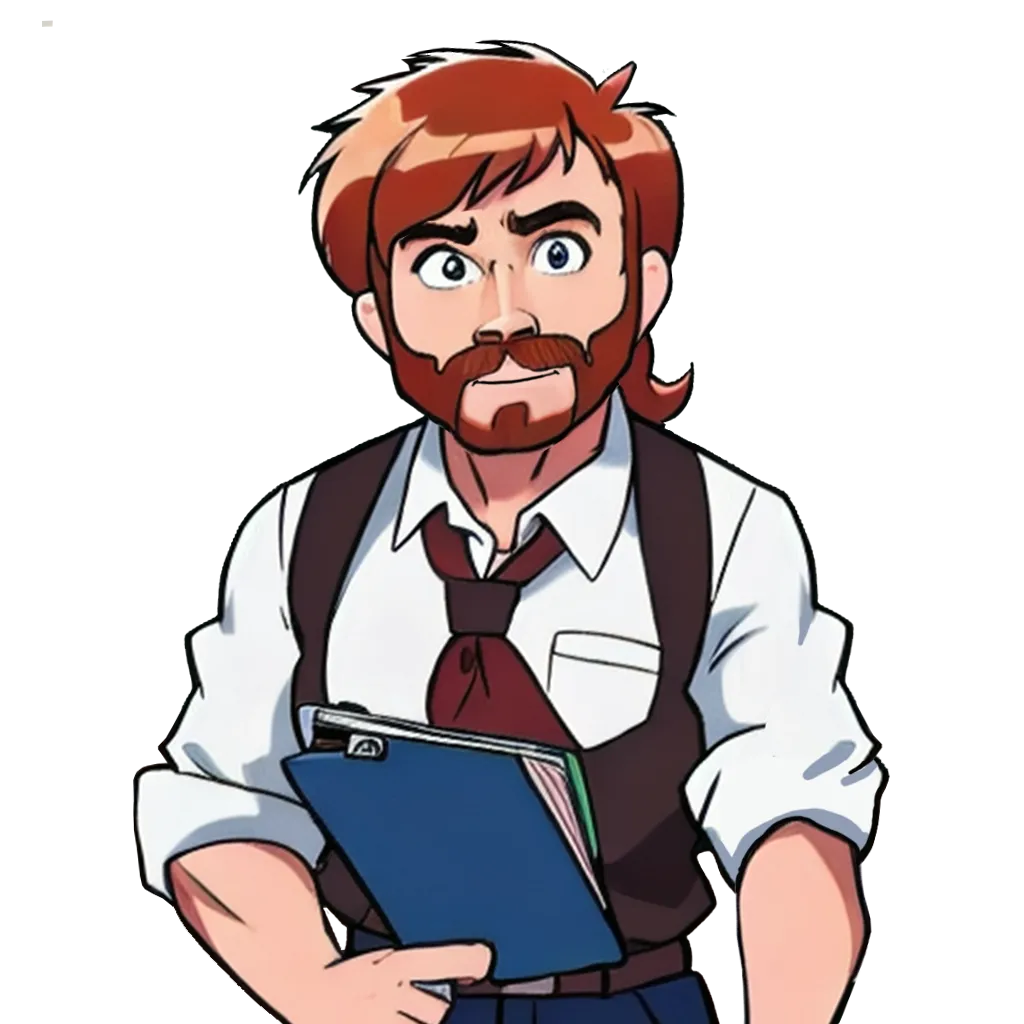
- Introduction to Async/Await in JavaScript
- Fundamentals of Asynchronous Programming
- Promises in JavaScript
- The Callback Hell Problem
- Transition from Promises to Async/Await
- Async/Await Syntax
- Error Handling with Try/Catch
- Asynchronous Operations in Sequence
- Parallel Asynchronous Operations
- Using Async/Await with the Fetch API
- Integration with Asynchronous Libraries
- Debugging in Asynchronous Code
- Common Use Cases and Best Practices
- Comparison with Other Asynchronous Techniques
- Conclusions and Next Steps