Docker
Writing and Optimizing Images
The Dockerfile is an essential tool in Docker that allows for building customized images for applications. In this chapter, we will learn to write efficient Dockerfiles and optimize their structure to achieve lightweight and fast images.
What is a Dockerfile?
A Dockerfile is a text file that contains a series of instructions that Docker follows to build an image. Each instruction in the Dockerfile becomes a layer in the final image, so a good structure is essential to optimize its size and performance.
Basic Instructions in Dockerfile
Basic rules for writing dockerfiles
A basic Dockerfile contains instructions that specify the base image, the working directory, the files to be copied, and the command that runs the application. Below are some fundamental instructions explained:
FROM
Defines the base image from which the new image will be built. This image can be an official Docker Hub image or a custom image.
dockerfile
WORKDIR
Sets the working directory inside the container, where the following instructions will be executed.
dockerfile
COPY
Copies files from the host system to the container. This is useful for adding the application source code and other necessary files.
dockerfile
RUN
Executes commands in the container during the image building, like installing dependencies.
dockerfile
CMD
Defines the command that will run when the container starts. It is ideal for specifying the main application execution.
dockerfile
Dockerfile Optimization for Lightweight Images
To create more efficient images, it's important to follow best optimization practices in Dockerfiles.
1. Use Lightweight Base Images
Opting for lightweight images like alpine
can significantly reduce the final image size. Usage example:
dockerfile
2. Minimize RUN Usage
Every time RUN
is used, a new layer is created in the image. To reduce the number of layers, combine multiple commands in a single RUN
instruction.
dockerfile
3. Copy Only Necessary Files
Avoid copying unnecessary files to the container. For this, you can use a .dockerignore
file to exclude files.
Example of .dockerignore
:
Optimized Dockerfile Example
Below is an optimized Dockerfile for a Node.js application:
dockerfile
Building the Image with a Dockerfile
To build the image from a Dockerfile, use the following command:
bash
Conclusion
Writing efficient and optimized Dockerfiles is essential for creating lightweight and fast images in Docker. With careful structuring and proper use of instructions, containers can be created that deploy and scale quickly. In the next chapter, we will explore volumes and how to manage data storage in Docker for applications that require persistence.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
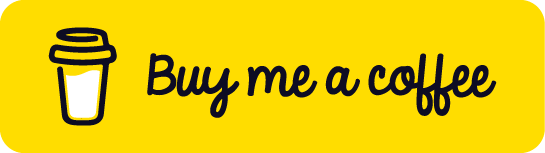
Chat with Chuck
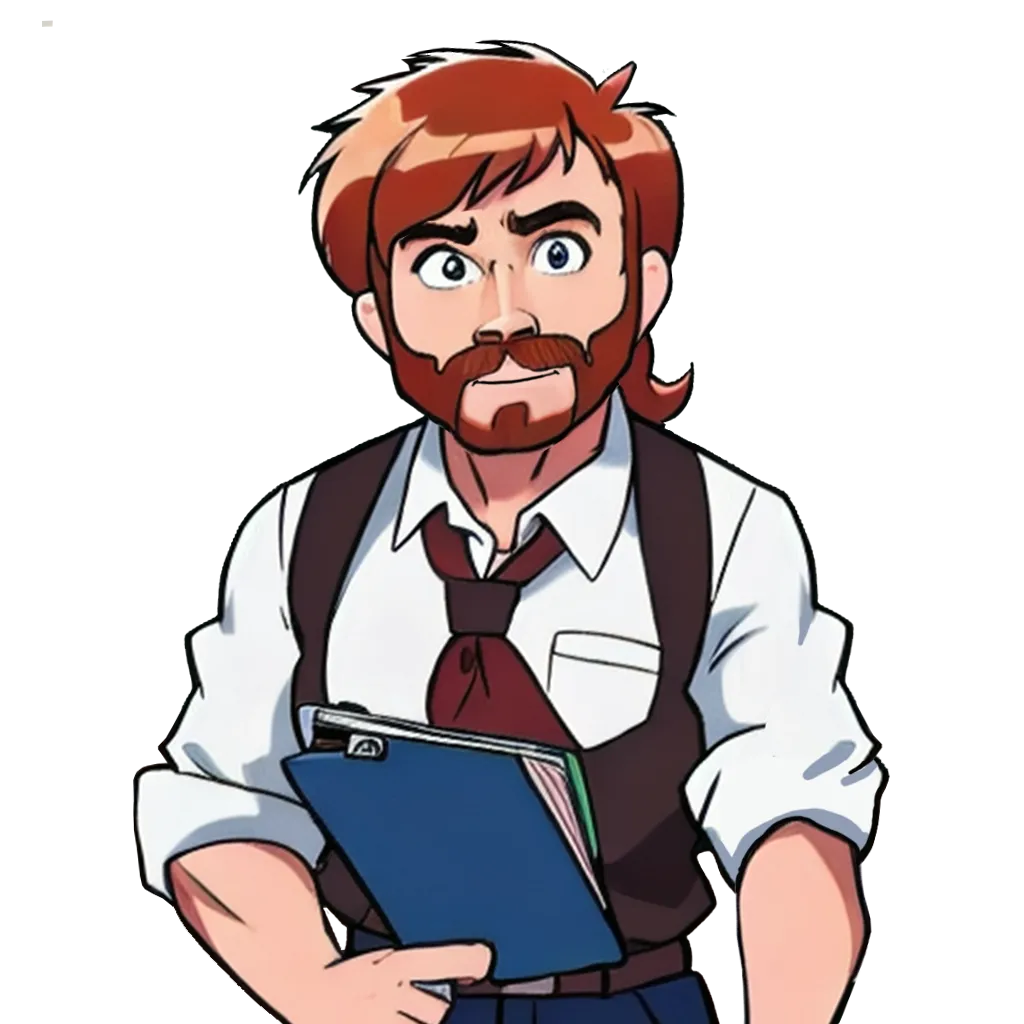
- Introduction to Docker and Containerization
- Installation and Configuration of Docker
- Principles of Containers and Virtualization
- Images in Docker: Creation and Management
- Writing and Optimizing Images
- Volumes and Persistent Storage in Docker
- Networking in Docker: Container Connectivity
- Docker Compose: Multi-Container Application Management
- Best Practices in Docker for Application Deployment
- Resource Management and Optimization in Docker
- Security in Docker and Best Containerization Practices
- Docker Swarm: Basic Container Orchestration
- Kubernetes vs Docker Swarm: Introduction to Kubernetes
- Deployment and Scalability with Kubernetes
- Continuous Integration and Continuous Delivery (CI/CD) with Docker
- Docker Image Registry: Docker Hub and Alternatives
- Monitoring and Logging of Containers in Docker
- Problem Solving and Debugging in Docker
- Migrating Applications to Docker Containers
- Practical Examples: Deploying Web Applications and APIs
- Conclusions and Best Practices in Using Docker