Express JS
Creating REST APIs with Express
REST APIs (Representational State Transfer) are a widely used standard for building web services that allow interaction between systems. In this chapter, we will learn how to create a REST API in Express while following best practices and adhering to RESTful principles. We will see how to handle HTTP requests, structure routes, and manage responses in JSON format.
What is a REST API?
A RESTful API is an interface that follows REST principles, which means it is:
- Stateless: Each client request to the server must contain all the necessary information, without relying on any saved state on the server.
- Resource-based: Resources (data) are identified by URLs, and operations on those resources use HTTP methods (GET, POST, PUT, DELETE).
- Scalable: RESTful APIs are designed to be scalable and easy to maintain.
Creating a Basic REST API in Express
To create a REST API in Express, we need to define routes that correspond to CRUD operations (Create, Read, Update, Delete). These operations correspond to the HTTP methods:
- GET: Retrieve data (read)
- POST: Send data (create)
- PUT: Update data
- DELETE: Delete data
Next, we will build a REST API to manage a simple resource like "users".
Defining REST Routes
Let's start by creating the basic routes to handle CRUD operations on our "users" resource.
javascript
Error Handling in the API
It is important to properly handle errors in a REST API to ensure the client receives clear and precise responses when something fails. For example, if we try to get a user that doesn't exist, we should return a 404 Not Found
status code.
We have already implemented some basic error responses in the previous routes, like 404
for users not found. However, we can improve this by creating a more robust error-handling middleware:
javascript
API Versioning
As APIs evolve, it may be necessary to maintain multiple versions of the same API. API versioning allows us to make changes without breaking existing integrations. A common way to implement versioning is through the URL:
javascript
Best Practices for Designing REST APIs
- Use HTTP status codes correctly: Make sure to return the correct status codes, such as
200 OK
for successful requests,201 Created
for created resources,400 Bad Request
for malformed requests, and404 Not Found
for non-existent resources. - Use JSON for responses: JSON is the most commonly used data format in REST APIs. Make sure all your API responses are in JSON format.
- Make URLs readable: The API URLs should be clear and represent the resource they are manipulating. For example,
/users/123
is clearer than/getUser?id=123
. - Keep the API stateless: Do not store the request state on the server. Each request should contain all the necessary information to process it, such as authentication tokens and request data.
API Documentation
Documenting the API is essential for other developers to understand and use it correctly. Tools like Swagger or Postman are excellent options for generating and maintaining API documentation.
Using Swagger for Documentation
We can integrate Swagger into our Express application to automatically document the routes.
bash
Then, configure Swagger:
javascript
Conclusion
In this chapter, we have seen how to create a REST API in Express by following RESTful principles and handling CRUD operations for a basic resource. We also covered how to handle errors, version, and document our APIs.
- Introduction to Express JS
- Express Fundamentals
- Request and Response Management
- Estructura de Proyectos en Express
- Authentication and Authorization in Express
- Connecting Express with Databases
- Error Handling and Logging in Express
- Sending Emails in Express
- Security in Express Applications
- Advanced Middleware in Express
- Creating REST APIs with Express
- WebSocket Implementation in Express
- Implementation of Webhooks in Express
- Testing Express Applications
- Deployment of Express Applications
- Performance Optimization in Express
- Monitoring and Maintenance of Express Applications
- Best Practices and Scalability in Express
- Course Conclusion: Express JS
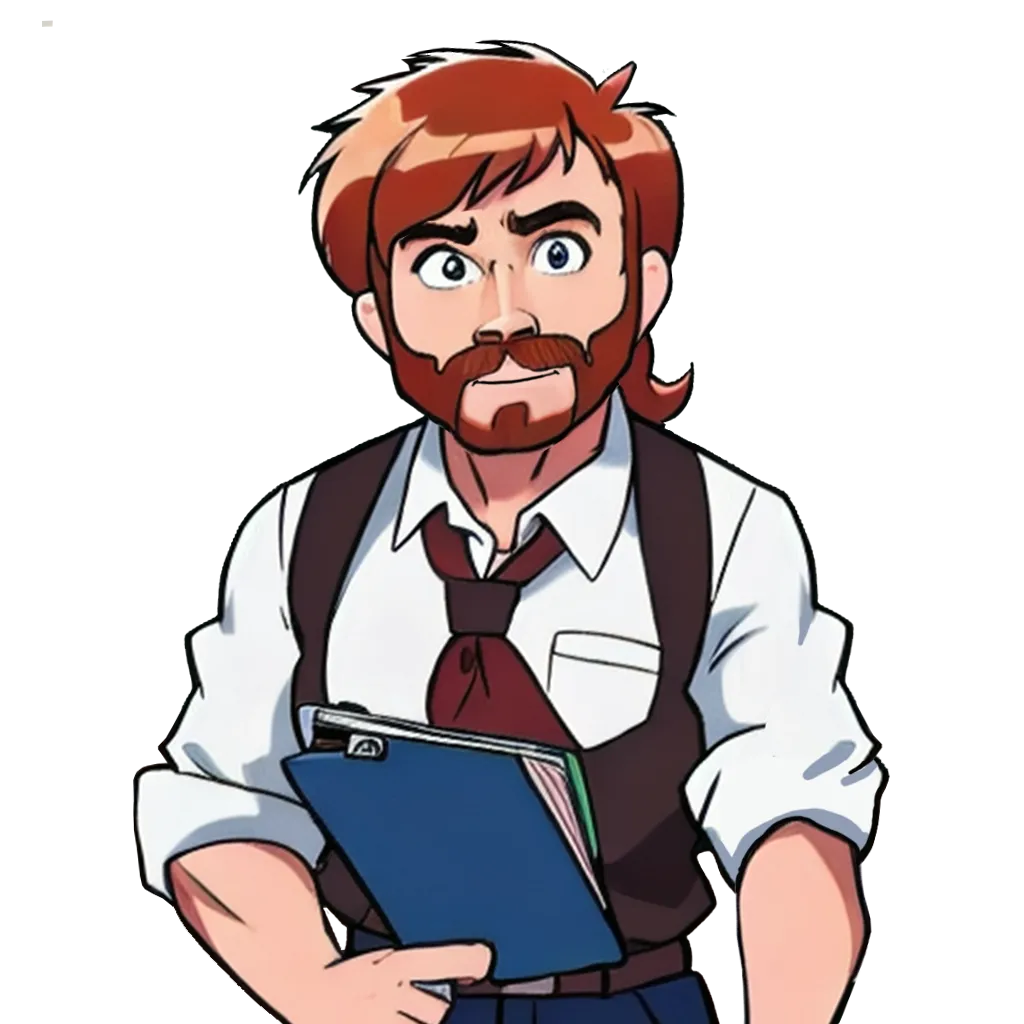