Express JS
Performance Optimization in Express
Optimizing the performance of an Express application is crucial for ensuring fast response times and a smooth user experience, especially as the number of users grows. In this chapter, we will explore various techniques and strategies to improve the performance of Express applications, including caching, compression, database optimization, and more.
Using Middleware for Compression
Compressing HTTP responses is a simple and effective way to reduce the size of the data sent to the client and therefore improve load times. The compression
middleware can automatically compress responses in gzip
format.
Installing and Using compression
First, we install the compression
middleware:
bash
Then, we configure it in our application:
javascript
Implementing Cache
Implementing caching is another essential technique to improve performance, as it allows storing previously calculated responses and avoiding repetitive operations. We can use Redis to store and quickly access cached data.
Installing Redis
First, we install redis
and the Node.js redis
client:
bash
Configuring Redis
We can implement a cache for HTTP requests as follows:
javascript
Database Optimization
A poorly optimized database can significantly slow down an application. To improve performance, it is important to follow best practices such as:
- Using indexes: Ensure that database queries are fast by using indexes on columns that are frequently queried.
- Efficient queries: Avoid queries that return large amounts of unnecessary data.
- Using ORM: Tools like Sequelize or Mongoose can help optimize queries and improve database performance.
Example of Indexes in MongoDB
If we use MongoDB with Mongoose, we can define indexes in our models to improve query performance.
javascript
Using Clustering to Leverage Multiple Cores
By default, Node.js uses a single CPU core. However, we can use cluster
to create multiple instances of our Express application and leverage the different cores available on the server.
Configuring Clustering
javascript
Request Rate Limiting
To prevent denial-of-service attacks (DoS) and abuse, we can implement a request rate limiter that controls the number of requests allowed per user in a time interval.
Installing and Configuring express-rate-limit
First, we install the express-rate-limit
middleware:
bash
Then, we configure it in our application:
javascript
Performance Monitoring
Implementing monitoring tools is essential to detect and solve performance issues in production. PM2 and New Relic are two popular tools for monitoring Node.js applications.
Monitoring with PM2
PM2 is a process manager for Node.js that allows running applications in cluster mode, automatically restarting on errors, and monitoring performance.
We install PM2 with the following command:
bash
We start the application using PM2:
bash
Best Optimization Practices
- Minimize middleware usage: Loading too many unnecessary middlewares can impact performance. Use only the necessary ones and avoid excessive processing in middlewares.
- Handle errors properly: Prevent unhandled errors from impacting performance. Use middlewares to efficiently handle errors.
- Optimize responses: Use techniques like compression, caching, and database query optimization to improve response performance.
- Constant monitoring: Implement monitoring tools to detect performance issues and fix them before they affect users.
Conclusion
In this chapter, we have learned various techniques to optimize the performance of an Express application, from caching and compression to the implementation of clustering and rate limiting. Performance optimization is essential to ensure that our applications can efficiently handle user load.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
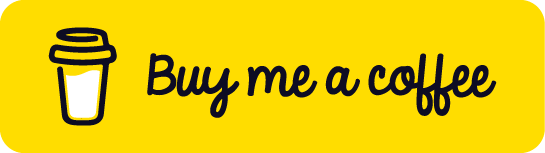
Chat with Chuck
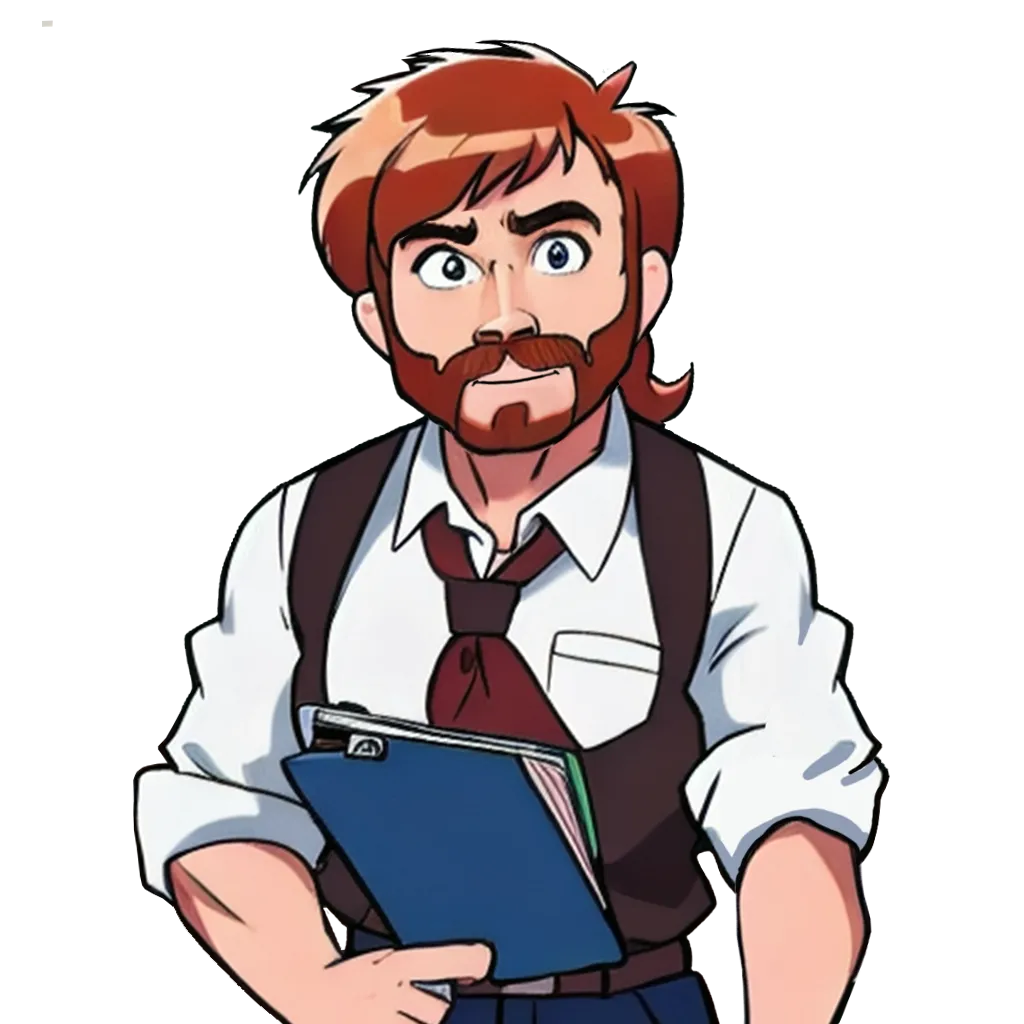
- Introduction to Express JS
- Express Fundamentals
- Request and Response Management
- Estructura de Proyectos en Express
- Authentication and Authorization in Express
- Connecting Express with Databases
- Error Handling and Logging in Express
- Sending Emails in Express
- Security in Express Applications
- Advanced Middleware in Express
- Creating REST APIs with Express
- WebSocket Implementation in Express
- Implementation of Webhooks in Express
- Testing Express Applications
- Deployment of Express Applications
- Performance Optimization in Express
- Monitoring and Maintenance of Express Applications
- Best Practices and Scalability in Express
- Course Conclusion: Express JS