Express JS
Testing Express Applications
Testing is an essential part of software development, as it helps us ensure that our application works correctly and identify bugs before they reach production. In this chapter, we will learn how to test Express applications using tools like Mocha, Chai, and Supertest. We will see how to create unit tests, integration tests, and how to automate the testing process.
Installation of Testing Tools
To perform tests on our Express application, we will use three main tools:
- Mocha: A testing framework for Node.js that allows us to define and execute our tests.
- Chai: An assertion library that allows us to define expectations about our application's behavior.
- Supertest: A tool for making HTTP requests to our Express application, allowing us to test our routes and APIs.
Installing Mocha, Chai, and Supertest
First, we install the necessary tools:
bash
Test Structure
It is recommended to organize our tests in a separate folder, such as test/
, to keep the code clean and easy to maintain. Within this folder, we can create specific test files for different parts of our application.
Writing Unit Tests
Unit tests focus on testing individual units of code, such as functions or methods, without worrying about how they interact with other parts of the application. In an Express application, we might write unit tests for helper functions or internal logic.
Example of a Unit Test
Suppose we have a helper function in our application that calculates the total of a purchase:
javascript
We can write a unit test for this function as follows:
javascript
Integration Tests with Supertest
Integration tests allow us to test how different parts of our application interact. In the case of Express, we can use Supertest to test our routes and ensure that our APIs respond correctly to HTTP requests.
Example of an Integration Test
Suppose we have an API that returns a list of users:
javascript
We can write an integration test for this route using Supertest:
javascript
Automating Tests with Mocha
Mocha allows us to run all tests in our application automatically. We can set up a script in our package.json
file to run tests using the following command:
json
Now, we can run our tests simply using the command:
bash
Testing Protected Routes
It is important to test routes that require authentication or special permissions. We can simulate sending authentication tokens or authorization headers using Supertest.
Example of a Protected Route Test
Suppose we have a route /admin
that only allows access to users with an admin token:
javascript
We can write an integration test for this route as follows:
javascript
Good Practices in Testing
- Ensure tests run quickly: Tests should run quickly so as not to slow down the development workflow.
- Keep tests isolated: Each test should be independent, so that changes in one do not affect the results of others.
- Test both positive and negative cases: Make sure to test not only success cases but also cases where things should fail.
- Automate the process: Use tools like Mocha and npm scripts to automatically run tests on each commit or deployment.
Conclusion
In this chapter, we have learned how to test Express applications using Mocha, Chai, and Supertest. Testing is crucial to ensuring the quality and stability of our applications, and we have covered how to write both unit tests and integration tests.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
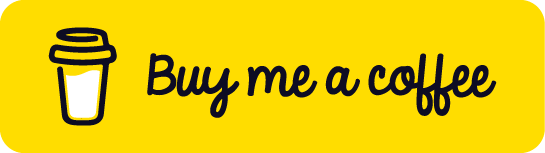
Chat with Chuck
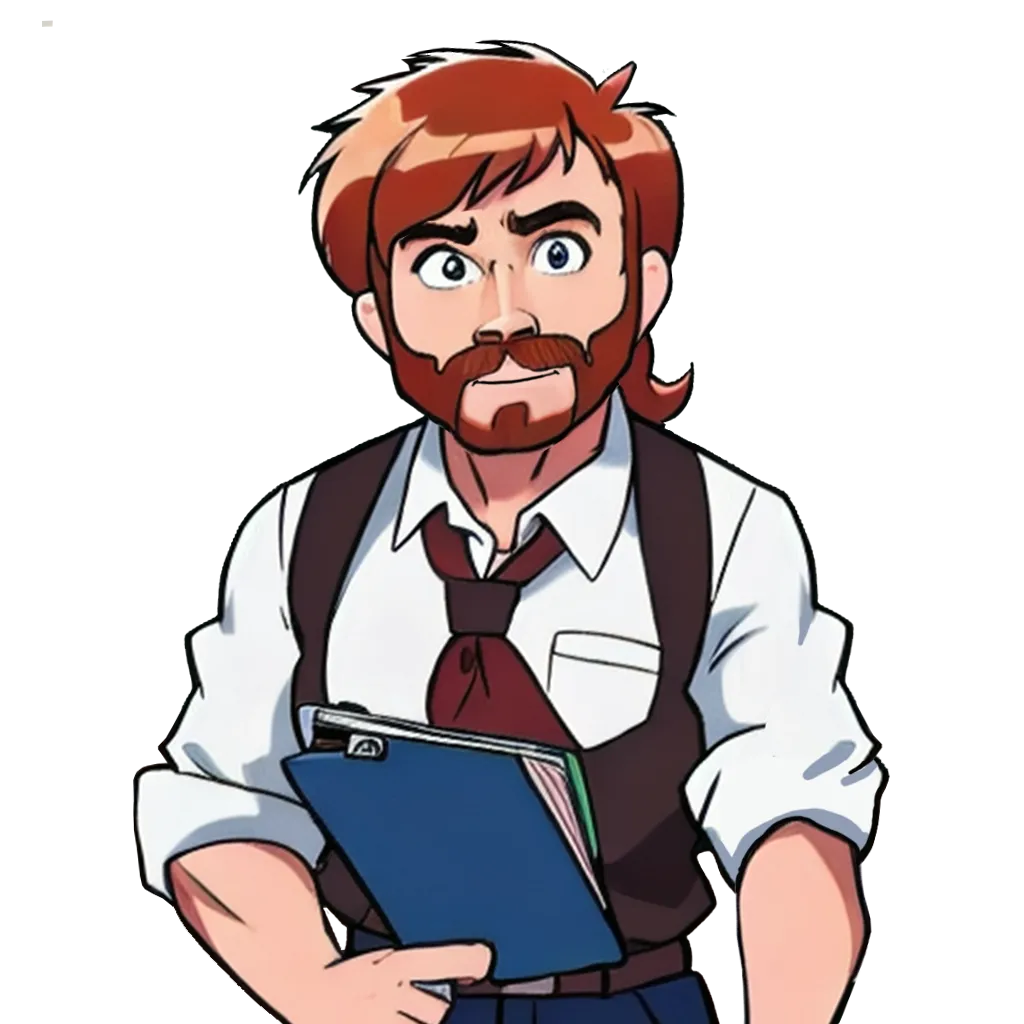
- Introduction to Express JS
- Express Fundamentals
- Request and Response Management
- Estructura de Proyectos en Express
- Authentication and Authorization in Express
- Connecting Express with Databases
- Error Handling and Logging in Express
- Sending Emails in Express
- Security in Express Applications
- Advanced Middleware in Express
- Creating REST APIs with Express
- WebSocket Implementation in Express
- Implementation of Webhooks in Express
- Testing Express Applications
- Deployment of Express Applications
- Performance Optimization in Express
- Monitoring and Maintenance of Express Applications
- Best Practices and Scalability in Express
- Course Conclusion: Express JS