Express JS
Best Practices and Scalability in Express
As our applications grow in size and complexity, it is important to follow best practices to keep the code clean, efficient, and scalable. In this chapter, we will explore some of the best practices for developing with Express and how to prepare our applications to easily scale as demand increases.
Code Organization
Proper code organization is key for the maintenance and scalability of any Express application. Below are some recommendations on how to efficiently structure your code.
Separation of Routes, Controllers, and Models
It is recommended to separate routes, controllers, and models into different files and folders. This helps keep the code modular and easy to understand.
Example of Project Structure
Using Middlewares
Using middlewares is an excellent way to add reusable functionalities to your application. However, it's important not to overload the application with unnecessary middlewares. Keep the middlewares focused on specific tasks and use them only where they are really needed.
Example of Middleware for Authentication
javascript
Best Practices for Error Handling
Proper error handling not only improves user experience but also facilitates debugging and maintaining the application. Here are some key best practices:
- Use Error Handling Middlewares: Implement a dedicated middleware to capture and handle errors throughout the application.
javascript
- Asynchronous Error Handling: Use
try-catch
blocks in asynchronous functions and pass errors to the error handling middleware usingnext(err)
.
javascript
Optimization for Scalability
A scalable application can handle an increase in user load and requests without degrading performance. Here are some techniques to prepare our Express applications to scale efficiently.
Using Clustering
Node.js by default uses a single CPU core. However, we can use the cluster
module to run multiple instances of our application and leverage all server cores.
javascript
Horizontal Scalability
Horizontal scalability involves adding more application instances to distribute the workload. Cloud services like AWS, Google Cloud, and Heroku allow automatic scaling based on demand.
Using PM2 for Scalability
PM2 allows running applications in cluster mode, enabling you to leverage multiple CPU cores without manually managing processes.
bash
Load Balancing
Load balancing distributes incoming requests among several application instances, improving responsiveness and preventing a single instance from being overloaded. A load balancer like NGINX or a cloud-managed service can be used to handle this automatically.
Example of NGINX Configuration
nginx
Using Caching
Caching is essential to improving the performance of high-demand applications. Redis is one of the most popular options for caching temporary data.
Example of Using Redis for Caching
javascript
Database Query Optimization
Optimizing database queries is key to improving overall application performance, especially when working with large data volumes.
- Use Indexes: Indexes allow faster searches on database tables.
- Paginated Queries: Avoid returning large data sets at once by using pagination.
- ORM Optimization: If you are using an ORM like Sequelize or Mongoose, make sure to use only necessary queries and avoid the overhead of complex relationships.
Security Best Practices
In addition to scalability, it is crucial to follow security best practices, as an application that scales quickly can also become vulnerable to attacks if not well-protected.
- Robust Authentication and Authorization: Use JWT tokens, OAuth, or secure sessions to authenticate users.
- Protection Against Common Attacks: Implement middleware like
helmet
to protect the application from code injection, cross-site scripting (XSS), and clickjacking attacks. - Request Rate Limiting: Use middleware like
express-rate-limit
to prevent denial-of-service (DoS) attacks.
Conclusion
In this chapter, we've explored various techniques and best practices to improve the organization, scalability, and security of Express applications. By following these recommendations, you can develop more robust and scalable applications capable of handling high traffic volumes without compromising performance or security. With this, we complete our guide on best practices and scalability in Express.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
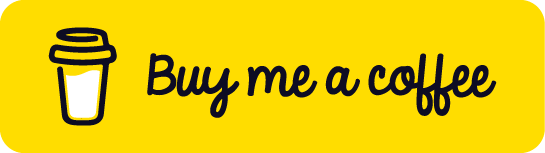
Chat with Chuck
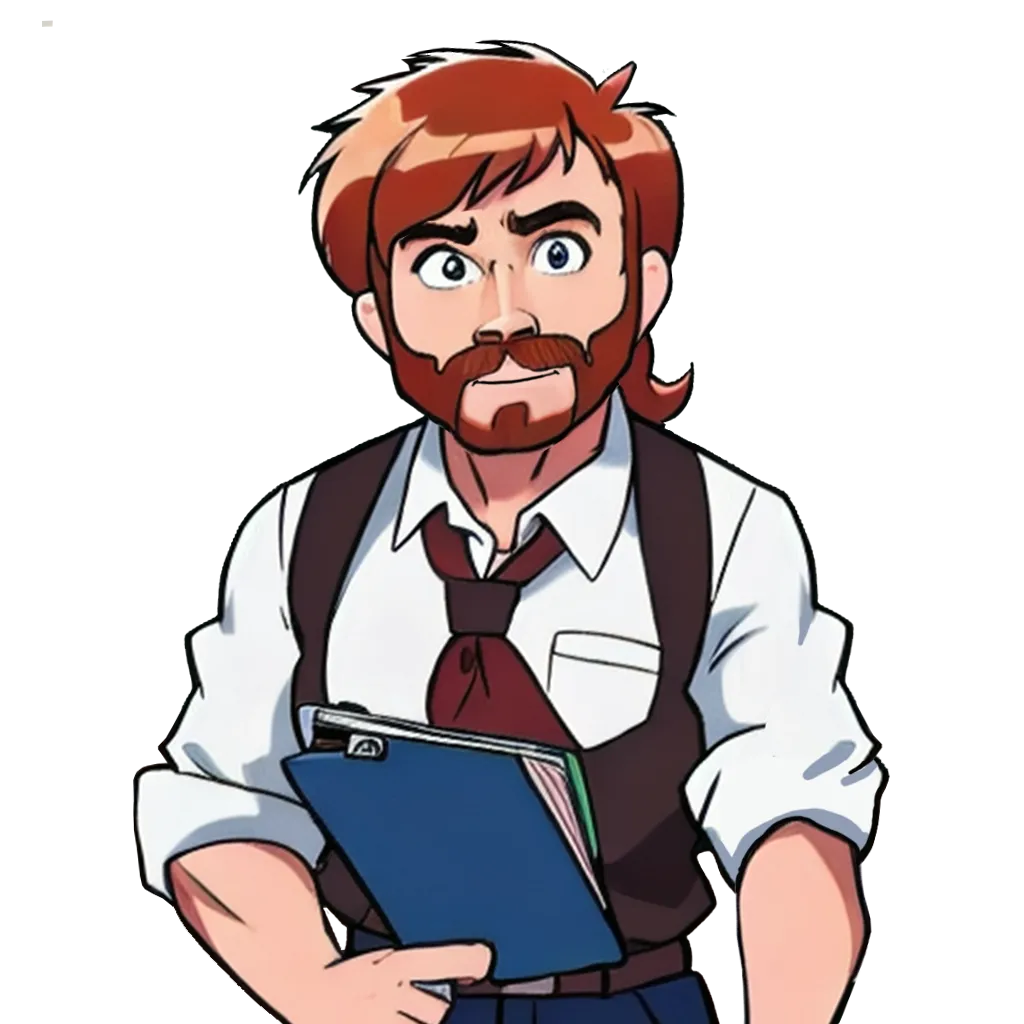
- Introduction to Express JS
- Express Fundamentals
- Request and Response Management
- Estructura de Proyectos en Express
- Authentication and Authorization in Express
- Connecting Express with Databases
- Error Handling and Logging in Express
- Sending Emails in Express
- Security in Express Applications
- Advanced Middleware in Express
- Creating REST APIs with Express
- WebSocket Implementation in Express
- Implementation of Webhooks in Express
- Testing Express Applications
- Deployment of Express Applications
- Performance Optimization in Express
- Monitoring and Maintenance of Express Applications
- Best Practices and Scalability in Express
- Course Conclusion: Express JS