Express JS
Implementation of Webhooks in Express
Webhooks are a powerful tool that allows an application to receive real-time event notifications from other services. They are very useful for integrating third-party applications and automating processes. In this chapter, we will learn how to implement webhooks in Express, how to manage incoming events, and how to secure requests to prevent abuse.
What is a Webhook?
A webhook is a mechanism that allows a service to send notifications or data to another system in response to specific events. Unlike traditional APIs, where the client makes a request, in webhooks, it is the server that sends an HTTP request to an endpoint in your application when an event occurs.
Common Examples of Webhooks
- GitHub: Sending notifications when a commit or pull request is made in a repository.
- Stripe: Notifications of successful or failed payments.
- Slack: Sending messages to a channel or user events.
Creating a Webhook in Express
To receive webhooks in our Express application, we need to create an endpoint that can process incoming HTTP requests. Webhooks generally send data in JSON format via POST requests.
Webhook Configuration Example
We create an endpoint in Express that will receive a sample webhook:
javascript
Webhook Validation
To ensure that the requests the webhook receives are authentic and come from reliable sources, it's important to validate the webhooks. Most services use signatures or tokens in requests to authenticate their origin.
Signature Validation with Header
Suppose the service sending us webhooks adds a signature in the x-webhook-signature
header. We can validate the signature by comparing it with one generated locally using a shared secret.
javascript
Webhook Event Management
When receiving a webhook, we usually need to process the event differently depending on the type of event. For example, a service like Stripe can send different types of events, such as a successful payment or a refund. We can handle these events individually.
Event Handling Example
javascript
Webhook Security
It is crucial to protect our webhooks to prevent malicious attacks. Below are some strategies to enhance the security of our webhooks:
- Signature Verification: As we saw earlier, validating the webhook signature is one of the best ways to ensure that the event is legitimate.
- HTTPS: Always use HTTPS to ensure that the webhook information is encrypted and cannot be intercepted.
- Tokens and Authentication: Some services allow webhooks to include an authentication token in the URL or request headers. Validate that token in each request.
Webhook Testing
To test webhooks locally during development, we can use tools like ngrok
, which creates a tunnel to our local server and allows external services to send webhooks to our development machine.
Using Ngrok for Webhook Testing
First, we install ngrok
and run it on the port of our local application:
bash
This generates a public URL, such as https://random-url.ngrok.io
, that we can use to test webhooks.
Common Use Cases for Webhooks
Some common use cases for webhooks include:
- Payments: Payment services like Stripe or PayPal send webhooks to notify about successful, failed transactions, or refunds.
- Task Automation: Webhooks can be used to automate processes in external systems when certain events occur, such as account creation or data updates.
- Notifications: Platforms like Slack use webhooks to receive real-time event notifications and execute them in different channels.
Best Practices for Webhooks
- Log Webhooks: It is crucial to log each received webhook, including its content and arrival time, to facilitate troubleshooting in case of errors.
- Retries: Some services will resend webhooks if they do not receive a successful response. Implement logic to handle duplicate requests.
- Respond Quickly: Webhooks should be processed quickly. If event processing takes a long time, respond with a 200 code immediately and handle the event asynchronously.
Conclusion
In this chapter, we have learned what webhooks are and how to implement them in Express, from creating an endpoint to validating and managing incoming events. Webhooks are a powerful tool for integration between systems, and with the right practices, we can ensure they work securely and efficiently in our applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
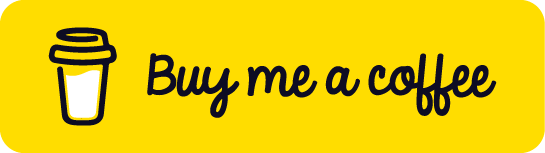
Chat with Chuck
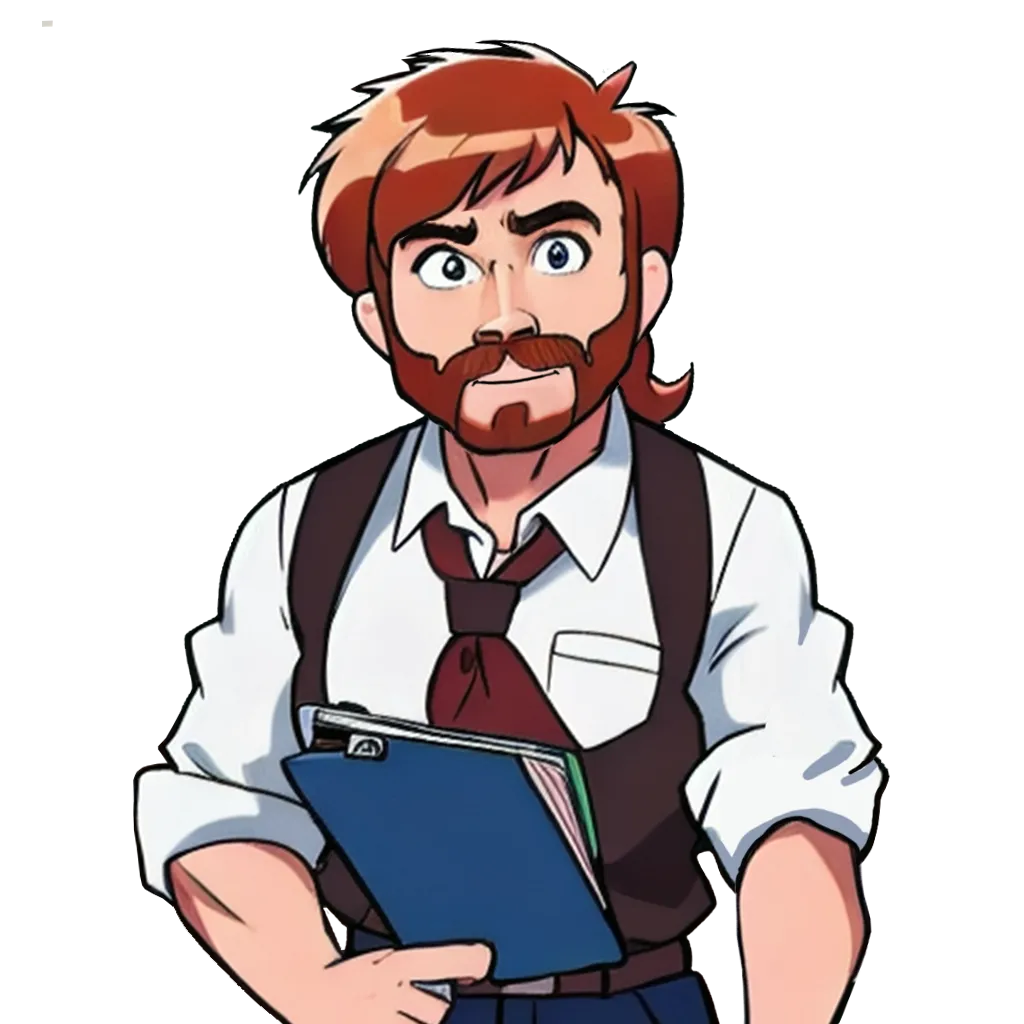
- Introduction to Express JS
- Express Fundamentals
- Request and Response Management
- Estructura de Proyectos en Express
- Authentication and Authorization in Express
- Connecting Express with Databases
- Error Handling and Logging in Express
- Sending Emails in Express
- Security in Express Applications
- Advanced Middleware in Express
- Creating REST APIs with Express
- WebSocket Implementation in Express
- Implementation of Webhooks in Express
- Testing Express Applications
- Deployment of Express Applications
- Performance Optimization in Express
- Monitoring and Maintenance of Express Applications
- Best Practices and Scalability in Express
- Course Conclusion: Express JS