Express JS
Deployment of Express Applications
The deployment of applications is a critical part of software development, as it involves taking our application from the development environment to a production environment accessible to end users. In this chapter, we will explore different options for deploying Express applications, including popular platforms like Heroku, AWS, and DigitalOcean. We will also learn how to use Docker to facilitate the deployment process.
Preparation for Deployment
Before deploying an Express application, we must ensure that it is properly configured for a production environment. This includes:
- Environment Variables: It's important not to expose sensitive information, such as API keys or database credentials, in the code. We will use environment variables to store this information.
- Error Handling: Make sure that error handling is configured not to expose internal details of the application to end users.
- Compression and Caching: It's recommended to use middleware like
compression
to reduce the size of HTTP responses and improve performance.
Configuring Environment Variables
We can use the dotenv
package to manage environment variables securely. Install it with the following command:
bash
Then, create an .env
file to store environment variables:
In our app.js
file, we load the environment variables:
javascript
Deployment on Heroku
Heroku
Heroku is a popular cloud platform for deploying applications quickly without the need to manage servers. Below are the steps to deploy an Express application on Heroku.
Step 1: Create an Account on Heroku
First, sign up at Heroku if you don't have an account.
Step 2: Install Heroku CLI
Install the Heroku command line tool to manage your applications from the terminal:
bash
Step 3: Log in to Heroku
Log into your Heroku account from the terminal:
bash
Step 4: Create an Application on Heroku
Navigate to your project directory and create a new application on Heroku:
bash
Step 5: Deploy the Application on Heroku
Add a Procfile
in the root directory of your project. This file tells Heroku how to start your application.
Then, initialize Git if you haven't already and deploy:
bash
Step 6: Verify Deployment
Once the deployment is complete, you can open your application in the browser:
bash
Deployment on AWS (Amazon Web Services)
AWS
AWS is a robust cloud platform offering various options for deploying applications. One of the simplest options is using AWS Elastic Beanstalk, a service that automatically handles infrastructure provisioning and scaling.
Step 1: Configure AWS CLI
First, install and configure the AWS CLI:
bash
Step 2: Create an Application on Elastic Beanstalk
Navigate to your project directory and create a new application on Elastic Beanstalk:
bash
Step 3: Deploy the Application
Then, deploy your application with the following command:
bash
Once deployed, you can open the application in your browser:
bash
Deployment with Docker
Docker
Docker is a platform that allows packaging applications in containers, facilitating deployment in any environment. Below, we will see how to create a Docker container for an Express application.
Step 1: Create a Dockerfile
First, create a Dockerfile
in the root directory of your project:
This Dockerfile
defines the Node.js image, copies the necessary files, installs dependencies, and exposes port 3000.
Step 2: Build the Docker Image
Build the Docker image with the following command:
bash
Step 3: Run the Docker Container
Then, run the container:
bash
Best Practices for Deployment
- Use a Continuous Integration (CI/CD) Process: Automate the testing and deployment process using tools like Jenkins, GitHub Actions, or Travis CI.
- Monitor the Application in Production: Implement monitoring tools like New Relic, PM2, or Datadog to oversee performance and detect errors in real-time.
- Scalability: Ensure the infrastructure can scale vertically or horizontally to handle increasing traffic.
- Security in Deployment: Set up HTTPS to secure communications and protect API keys using services like AWS Secrets Manager or Heroku's environment variables.
Conclusion
In this chapter, we have seen how to deploy Express applications on popular platforms like Heroku, AWS, and how to use Docker for containers. Deployment is a fundamental part of the software development lifecycle, and it's important to follow best practices to ensure the application runs optimally in production.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
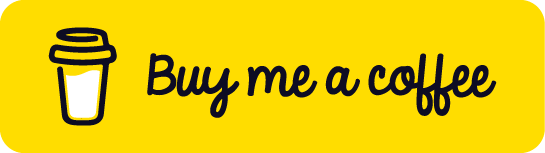
Chat with Chuck
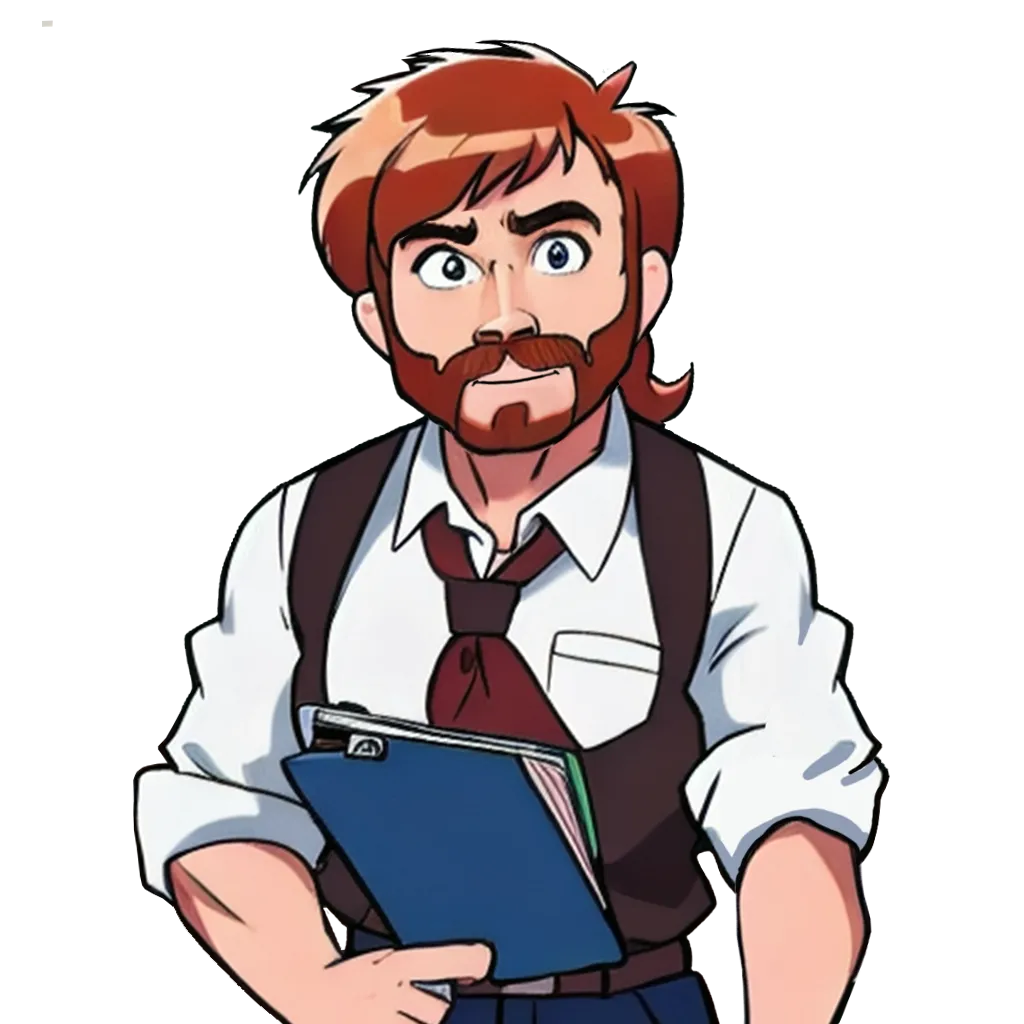
- Introduction to Express JS
- Express Fundamentals
- Request and Response Management
- Estructura de Proyectos en Express
- Authentication and Authorization in Express
- Connecting Express with Databases
- Error Handling and Logging in Express
- Sending Emails in Express
- Security in Express Applications
- Advanced Middleware in Express
- Creating REST APIs with Express
- WebSocket Implementation in Express
- Implementation of Webhooks in Express
- Testing Express Applications
- Deployment of Express Applications
- Performance Optimization in Express
- Monitoring and Maintenance of Express Applications
- Best Practices and Scalability in Express
- Course Conclusion: Express JS