Express JS
Security in Express Applications
Security is a critical aspect of any web application, especially in backend development. In this chapter, we will explore various techniques to protect our Express applications from common threats like injection attacks, session hijacking, and other vulnerabilities. We will see how to implement HTTPS, protect against XSS, CSRF attacks, and other best practices to keep our application secure.
Using HTTPS
Using HTTPS instead of HTTP is essential to protect the communication between the server and the client. HTTPS encrypts the data sent and received, which helps prevent man-in-the-middle attacks. In a production environment, it is important that our Express application is configured to run under HTTPS.
Configuring HTTPS
To configure HTTPS in Express, we need a valid SSL certificate. If we are in a development environment, we can generate a self-signed one, but for production, it is recommended to obtain a certificate from a trusted authority.
javascript
Protection against SQL Injection Attacks
SQL injections are one of the most common vulnerabilities. They occur when an attacker can insert or manipulate SQL queries through application inputs. To prevent such attacks, we should always use parameterized queries instead of directly concatenating strings in SQL queries.
Example of a Secure Query
If we are using Sequelize or another ORM, it is easier to avoid SQL injections as they handle input sanitization automatically. Here is an example of preventing SQL injections:
javascript
Protection against XSS (Cross-Site Scripting) Attacks
XSS attacks occur when an attacker inserts malicious code into a web page, which is then executed in the user's browser. To prevent these attacks, it's essential that all user inputs are sanitized before being rendered in the browser.
Helmet Middleware to Enhance Security
Helmet is a middleware that helps secure Express applications by setting various security-related HTTP headers. It protects against common attacks like XSS, clickjacking, and others.
Installing Helmet
First, install the Helmet package:
bash
Using Helmet in Express
To use Helmet, simply include it as a global middleware in our application.
javascript
Protection against CSRF (Cross-Site Request Forgery) Attacks
A CSRF attack occurs when an authenticated user is tricked into executing unwanted actions on an application where they are authenticated. To protect ourselves from such attacks, we can use the csurf
package, which generates unique tokens for each data modification request (POST, PUT, DELETE).
Installing csurf
Install the csurf
package:
bash
Configuring csurf
Then, configure csurf
as middleware in our application.
javascript
Encrypting Sensitive Data
When handling sensitive data like passwords, it is crucial to use secure hashing algorithms to encrypt them before storing them in the database. The bcrypt
package is an excellent choice for this task.
Installing bcrypt
First, install the bcrypt
package:
bash
Using bcrypt
to Encrypt Passwords
Below, we see how to encrypt a password before storing it in the database and how to verify it during login.
javascript
Best Security Practices in Express
- Keep all dependencies up to date: External libraries and packages can contain vulnerabilities that are fixed in later versions. Always keep your dependencies updated.
- Limit request rate: Implementing a request rate limiter prevents brute force and denial of service (DoS) attacks. The
express-rate-limit
package can help set a request limit per user. - Use Content Security Policy (CSP): Configuring a content security policy helps mitigate XSS attacks. This can be easily done with Helmet.
- Protect sessions: Ensure session cookies are secure by marking the
secure
andhttpOnly
options on cookies to prevent session hijacking attacks.
Conclusion
In this chapter, we have explored various ways to protect an Express application against common attacks, such as SQL injections, XSS, CSRF, and more. Implementing good security practices is essential to ensure that our web applications are secure and reliable.
- Introduction to Express JS
- Express Fundamentals
- Request and Response Management
- Estructura de Proyectos en Express
- Authentication and Authorization in Express
- Connecting Express with Databases
- Error Handling and Logging in Express
- Sending Emails in Express
- Security in Express Applications
- Advanced Middleware in Express
- Creating REST APIs with Express
- WebSocket Implementation in Express
- Implementation of Webhooks in Express
- Testing Express Applications
- Deployment of Express Applications
- Performance Optimization in Express
- Monitoring and Maintenance of Express Applications
- Best Practices and Scalability in Express
- Course Conclusion: Express JS
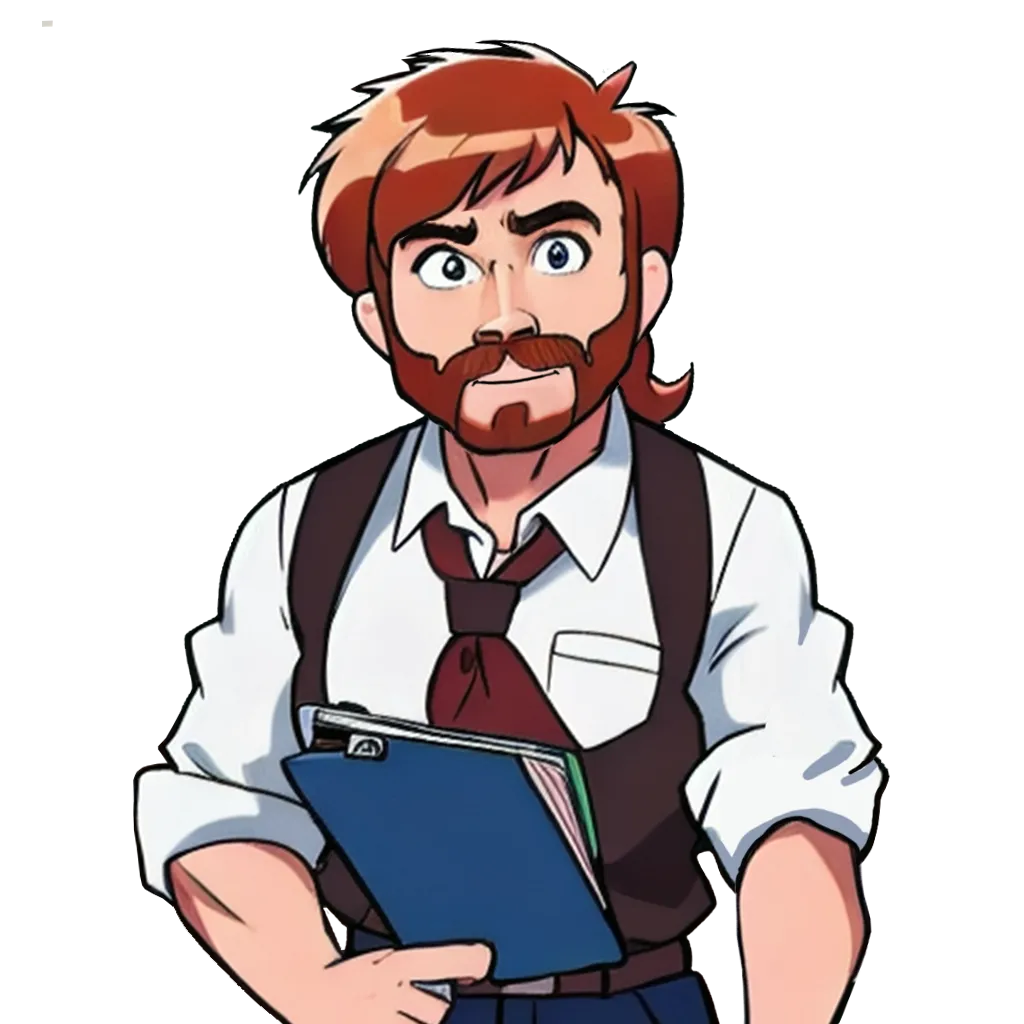