Express JS
Sending Emails in Express
Sending emails is a common feature in web applications, whether for account confirmations, password resets, or notifications. In this chapter, we'll learn how to send emails in an Express application using the nodemailer service.
Installing Nodemailer
Nodemailer is a Node.js library that allows sending emails easily. The first thing we need to do is install nodemailer in our project.
bash
Configuring Nodemailer
To send emails, we need to set up an email service. In this example, we'll use Gmail as the email provider. Below is how to configure nodemailer to send emails using Gmail.
javascript
Sending Emails
Once we have the mail transporter configured, we can create a route that sends an email when accessed. Let's see an example.
javascript
Using HTML Templates in Emails
Often, we want our emails to have a more advanced format than plain text. For this, we can send emails in HTML format using nodemailer.
javascript
Using Third-Party Services to Send Emails
If we don't want to rely on services like Gmail, we can use third-party services specialized in mass email sending, such as SendGrid or Mailgun. These services usually offer APIs that are easy to integrate with Express and are ideal for sending emails at scale.
Example with SendGrid
To use SendGrid, first, we need to create an account on SendGrid and obtain an API key. Then, we install the official SendGrid package.
bash
Then, we configure SendGrid with our API key and send an email:
javascript
Best Practices for Sending Emails
- Do not send sensitive data via email: Email is not a secure channel for sending sensitive information, such as passwords or bank details.
- Use multi-factor authentication: If you use third-party services like Gmail or SendGrid, enable multi-factor authentication on the associated account to enhance security.
- Handle errors properly: Always handle errors that may occur during the email sending process. If the sending fails, it is important to notify the user in a friendly manner.
- Avoid being marked as spam: If you send mass emails, make sure to comply with anti-spam regulations, such as including an unsubscribe link or using appropriate headers.
Conclusion
Sending emails is an essential feature in many web applications. With Express and nodemailer, it's easy to implement this feature. In this chapter, we've seen how to configure nodemailer to send emails with plain text and HTML, as well as how to use third-party services like SendGrid.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
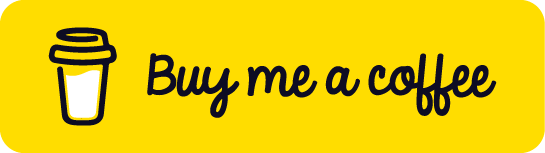
Chat with Chuck
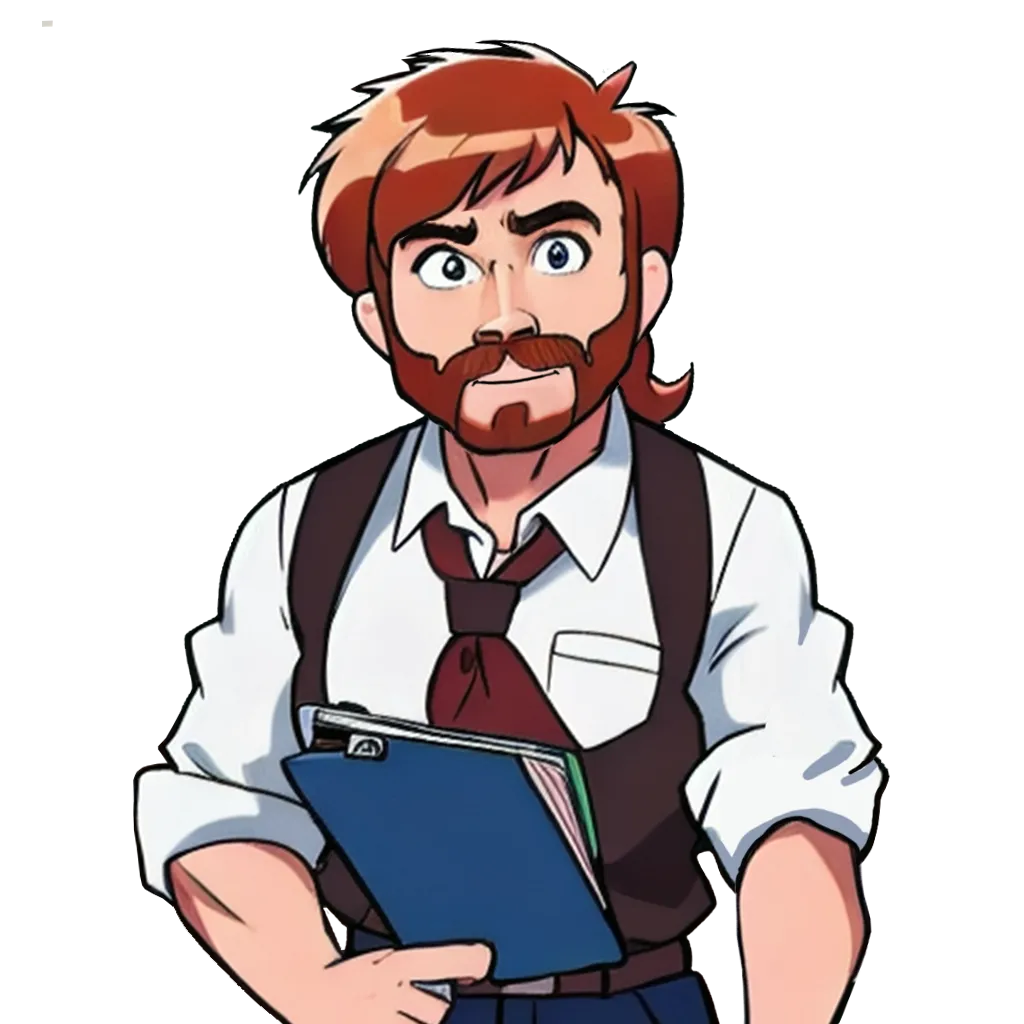
- Introduction to Express JS
- Express Fundamentals
- Request and Response Management
- Estructura de Proyectos en Express
- Authentication and Authorization in Express
- Connecting Express with Databases
- Error Handling and Logging in Express
- Sending Emails in Express
- Security in Express Applications
- Advanced Middleware in Express
- Creating REST APIs with Express
- WebSocket Implementation in Express
- Implementation of Webhooks in Express
- Testing Express Applications
- Deployment of Express Applications
- Performance Optimization in Express
- Monitoring and Maintenance of Express Applications
- Best Practices and Scalability in Express
- Course Conclusion: Express JS