Express JS
Request and Response Management
Request and response management is one of the fundamental concepts in any web application. In Express, the interaction between the client and server is based on receiving HTTP requests and sending responses. In this chapter, we will learn how to handle these requests and responses in Express, from accessing request parameters, bodies, and headers to sending responses with different status codes and formats.
Understanding the Request
Every time a client makes a request to a server, Express creates a req
(request) object that contains all the information related to that request, such as parameters, body, cookies, and headers.
Accessing Route Parameters
When a route is defined in Express, we can access the dynamic parameters that are part of the URL using req.params
.
javascript
Accessing Query Parameters
Query parameters are those passed in the URL after the question mark ?
. Express makes them available through req.query
.
javascript
Accessing the Request Body
To access the body of a request (usually in POST or PUT requests), we need to use the middleware express.json()
or express.urlencoded()
if the data is in JSON format or form format.
javascript
Accessing Request Headers
HTTP headers provide additional information about the request. We can access headers using req.headers
.
javascript
Sending Responses
In Express, the res
(response) object is used to send responses back to the client. We can send responses in various formats like text, JSON, and redirections.
Sending Text Responses
We can send a simple text response using res.send()
.
javascript
Sending JSON Responses
To send responses in JSON format, we use res.json()
.
javascript
Setting HTTP Status Codes
HTTP status codes indicate the result of a request. We can set a status code using res.status()
.
javascript
Redirecting Requests
We can redirect clients to a new URL using res.redirect()
.
javascript
Sending Files
Express also allows sending files as a response using res.sendFile()
.
javascript
Middleware for Handling Requests and Responses
Middleware in Express is a function that can intercept requests and responses in the application's lifecycle. Middlewares are commonly used to authenticate users, validate data, or log events.
Request Logging Middleware
We can create middleware to log all requests received by the application.
javascript
Error Handling Middleware
To manage errors in a centralized manner, we can use error-handling middleware.
javascript
Best Practices for Handling Requests and Responses
- Input Validation: Always validate request data before processing it to prevent errors and security vulnerabilities.
- Header Security: Use tools like
helmet
to secure HTTP headers and prevent common attacks such as clickjacking or cross-site scripting (XSS). - Efficient Response Sending: Try to minimize the size of responses by using compression or sending only necessary data to improve performance.
- Error Handling: Implement a global error handling system to capture any problems and prevent responses with sensitive information from being sent to clients.
Conclusion
In this chapter, we have learned how Express handles HTTP requests and responses, from accessing request parameters and bodies to sending responses in different formats and setting status codes. This knowledge is essential for building robust and efficient web applications.
- Introduction to Express JS
- Express Fundamentals
- Request and Response Management
- Estructura de Proyectos en Express
- Authentication and Authorization in Express
- Connecting Express with Databases
- Error Handling and Logging in Express
- Sending Emails in Express
- Security in Express Applications
- Advanced Middleware in Express
- Creating REST APIs with Express
- WebSocket Implementation in Express
- Implementation of Webhooks in Express
- Testing Express Applications
- Deployment of Express Applications
- Performance Optimization in Express
- Monitoring and Maintenance of Express Applications
- Best Practices and Scalability in Express
- Course Conclusion: Express JS
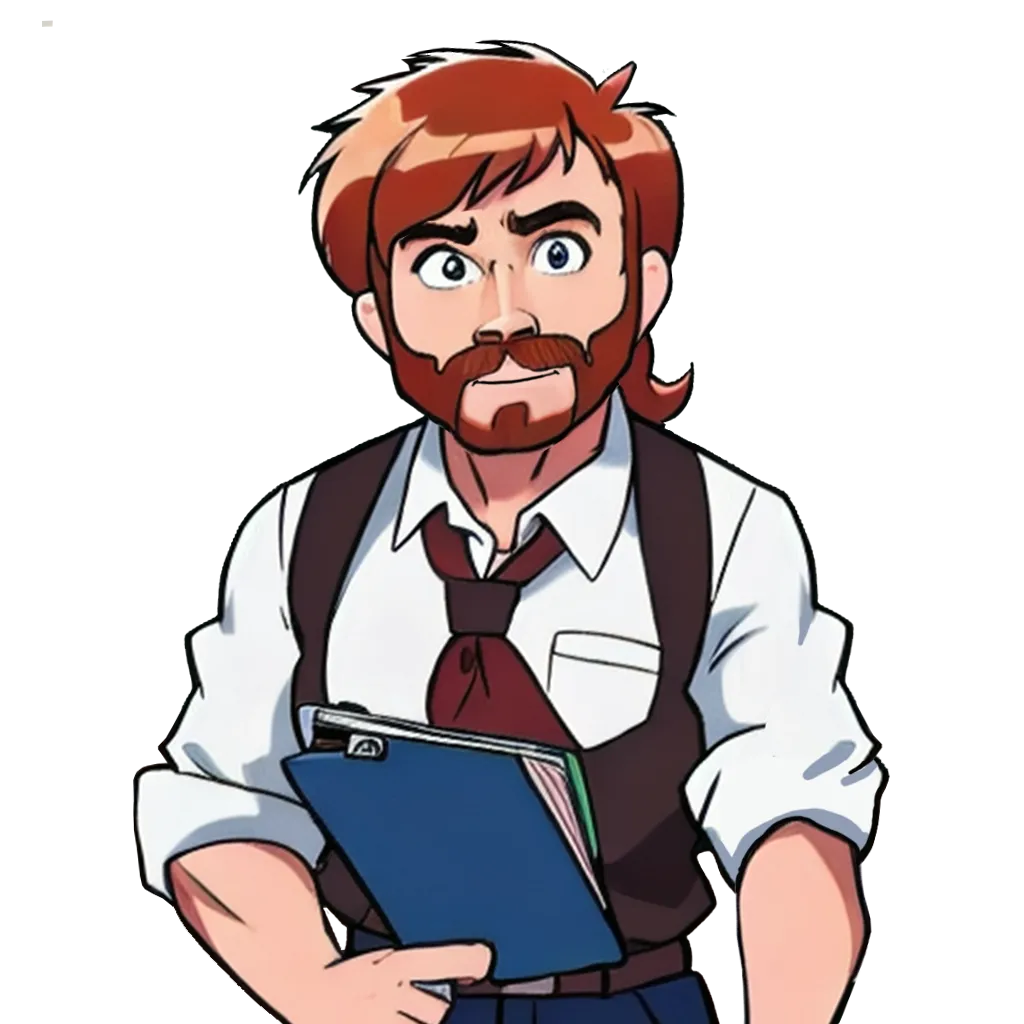