Express JS
WebSocket Implementation in Express
WebSockets are a technology that enables real-time, bidirectional communication between the client and the server through a persistent connection. This technology is especially useful for applications that require real-time updates, such as chats, notifications, or multiplayer games. In this chapter, we will learn how to implement WebSockets in an Express application using the ws
library.
What is a WebSocket?
A WebSocket is a communication protocol that allows an open connection to be maintained between the server and the client, which means the server can send data to the client without the client having to make an explicit request. This contrasts with the traditional HTTP model, where the client always has to initiate the request.
Installing the ws
Library
To implement WebSockets in Express, we will use the ws
library, which is one of the most popular ones for working with this protocol in Node.js.
Installing ws
First, we install the ws
package:
bash
Configuring WebSockets in Express
Next, we configure a WebSocket server in our Express application. The ws
library allows us to attach a WebSocket server to the existing HTTP server of Express.
WebSocket Implementation Example
javascript
Testing the WebSocket Connection
To test the WebSocket connection, we can use tools like Postman or write a simple WebSocket client in JavaScript. Below is an example of how to connect a WebSocket client from the browser.
javascript
Bidirectional Communication
WebSockets are bidirectional, meaning both the client and the server can initiate communication. Let's see an example where the server sends periodic notifications to all connected clients.
Sending Messages to All Clients
javascript
Authentication in WebSockets
Although WebSockets do not follow the traditional HTTP model, we can still implement authentication to protect our connections. A common way is to pass authentication tokens as parameters in the URL or in the headers during the connection establishment.
Authentication with WebSockets
Here's how to add authentication using a token in the URL.
javascript
Common WebSocket Applications
WebSockets are useful in applications that require real-time updates. Some common examples include:
- Real-time chats: Chat applications allow real-time communication between users.
- Multiplayer games: Online games can use WebSockets to synchronize game state between players.
- Live notifications: Applications can send real-time notifications to users, such as status updates or messages.
- Live price updates: Trading or stock applications can use WebSockets to send real-time price updates to users.
Best Practices with WebSockets
- Close inactive connections: It is important to close connections that remain inactive for a long time to avoid unnecessary resource consumption.
- Limit message rate: To avoid overloading the server or client, it is advisable to implement a rate limit for messages sent through WebSockets.
- Handle errors correctly: Ensure to handle errors like unexpected client disconnections or message send failures.
- Use HTTPS and WSS: To secure WebSocket connections, use
wss://
(WebSocket Secure) instead ofws://
and ensure all communications are encrypted.
Conclusion
In this chapter, we have learned how to implement WebSockets in an Express application using the ws
library. We have also explored how to manage bidirectional communication, authenticate clients, and apply best practices for working with WebSockets. This technology is essential for creating real-time applications, offering an interactive and dynamic experience for users.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
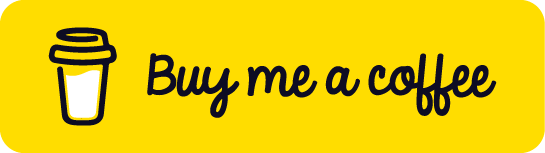
Chat with Chuck
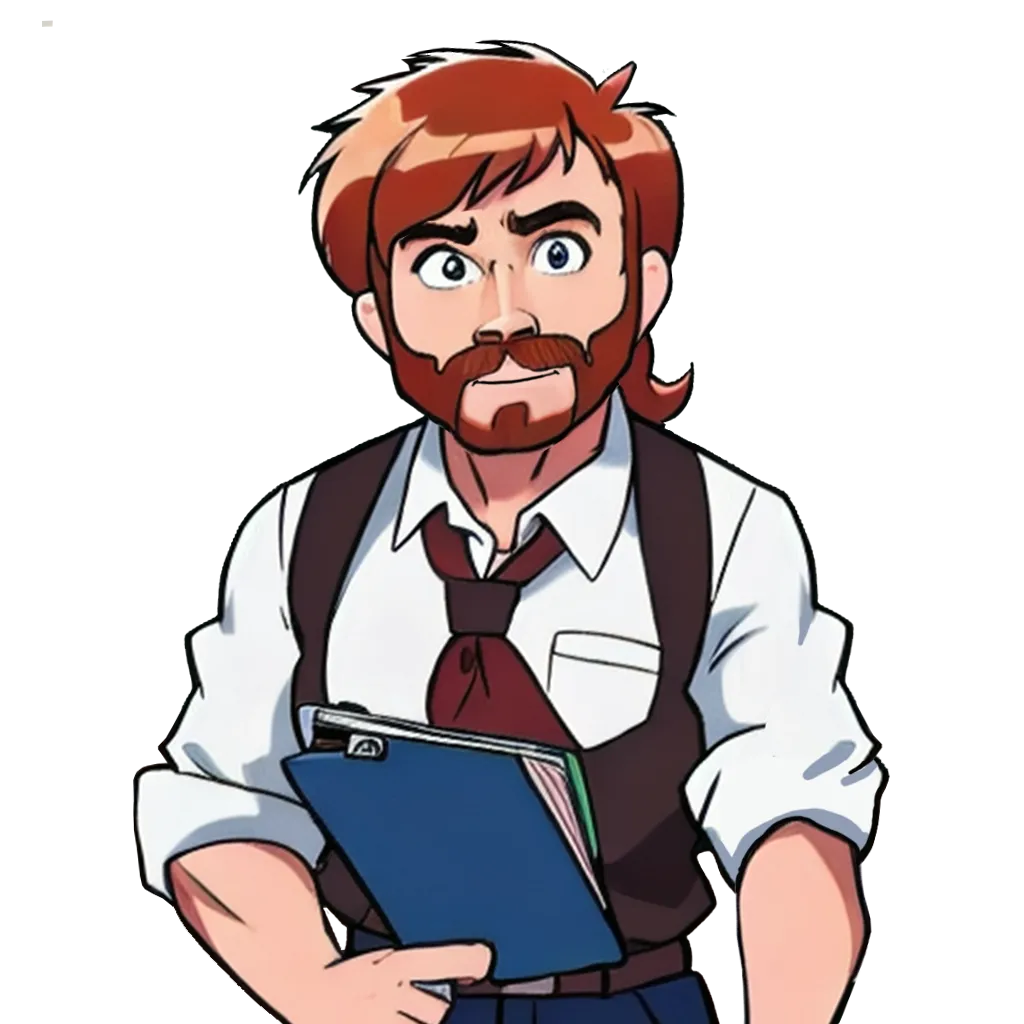
- Introduction to Express JS
- Express Fundamentals
- Request and Response Management
- Estructura de Proyectos en Express
- Authentication and Authorization in Express
- Connecting Express with Databases
- Error Handling and Logging in Express
- Sending Emails in Express
- Security in Express Applications
- Advanced Middleware in Express
- Creating REST APIs with Express
- WebSocket Implementation in Express
- Implementation of Webhooks in Express
- Testing Express Applications
- Deployment of Express Applications
- Performance Optimization in Express
- Monitoring and Maintenance of Express Applications
- Best Practices and Scalability in Express
- Course Conclusion: Express JS