Express JS
Connecting Express with Databases
Data management is a central aspect of any application. In this chapter, we will learn how to connect Express with databases, both SQL and NoSQL. We will focus on how to interact with databases to store, update, and retrieve data. Also, we will see how to use ORMs and ODMs to facilitate these tasks.
Connecting to SQL Databases (MySQL)
To connect to a MySQL database from Express, we can use the mysql2
package or sequelize
as an ORM (Object-Relational Mapping) to abstract the interaction with the database.
Installing MySQL and Sequelize
First, let's install the necessary packages:
bash
Sequelize Configuration
Next, we configure Sequelize to connect to our MySQL database:
javascript
Defining Models with Sequelize
Once connected, we can define models to represent our tables in the database. For example, if we have a users table, we can create a User
model:
javascript
CRUD Operations with Sequelize
Now, let's see how to perform CRUD (Create, Read, Update, Delete) operations using Sequelize.
- Create a new user:
javascript
- Read users:
javascript
- Update a user:
javascript
- Delete a user:
javascript
Connecting to NoSQL Databases (MongoDB)
Now, let's see how to connect Express to a NoSQL database like MongoDB. For this, we will use mongoose
, an ODM (Object Data Modeling) that simplifies the interaction with MongoDB.
Installing Mongoose
First, let's install the mongoose
package:
bash
Mongoose Configuration
To connect to MongoDB, configure mongoose
as follows:
javascript
Defining Models with Mongoose
Just like with Sequelize, we can define models to represent documents in MongoDB. For example, a user model would be defined like this:
javascript
CRUD Operations with Mongoose
Performing CRUD operations with Mongoose is similar to Sequelize, but with some differences in syntax.
- Create a new user:
javascript
- Read users:
javascript
- Update a user:
javascript
- Delete a user:
javascript
Conclusion
In this chapter, we have seen how to connect Express to both SQL and NoSQL databases, and how to perform CRUD operations with Sequelize and Mongoose. The ability to interact with databases is essential for any backend application.
- Introduction to Express JS
- Express Fundamentals
- Request and Response Management
- Estructura de Proyectos en Express
- Authentication and Authorization in Express
- Connecting Express with Databases
- Error Handling and Logging in Express
- Sending Emails in Express
- Security in Express Applications
- Advanced Middleware in Express
- Creating REST APIs with Express
- WebSocket Implementation in Express
- Implementation of Webhooks in Express
- Testing Express Applications
- Deployment of Express Applications
- Performance Optimization in Express
- Monitoring and Maintenance of Express Applications
- Best Practices and Scalability in Express
- Course Conclusion: Express JS
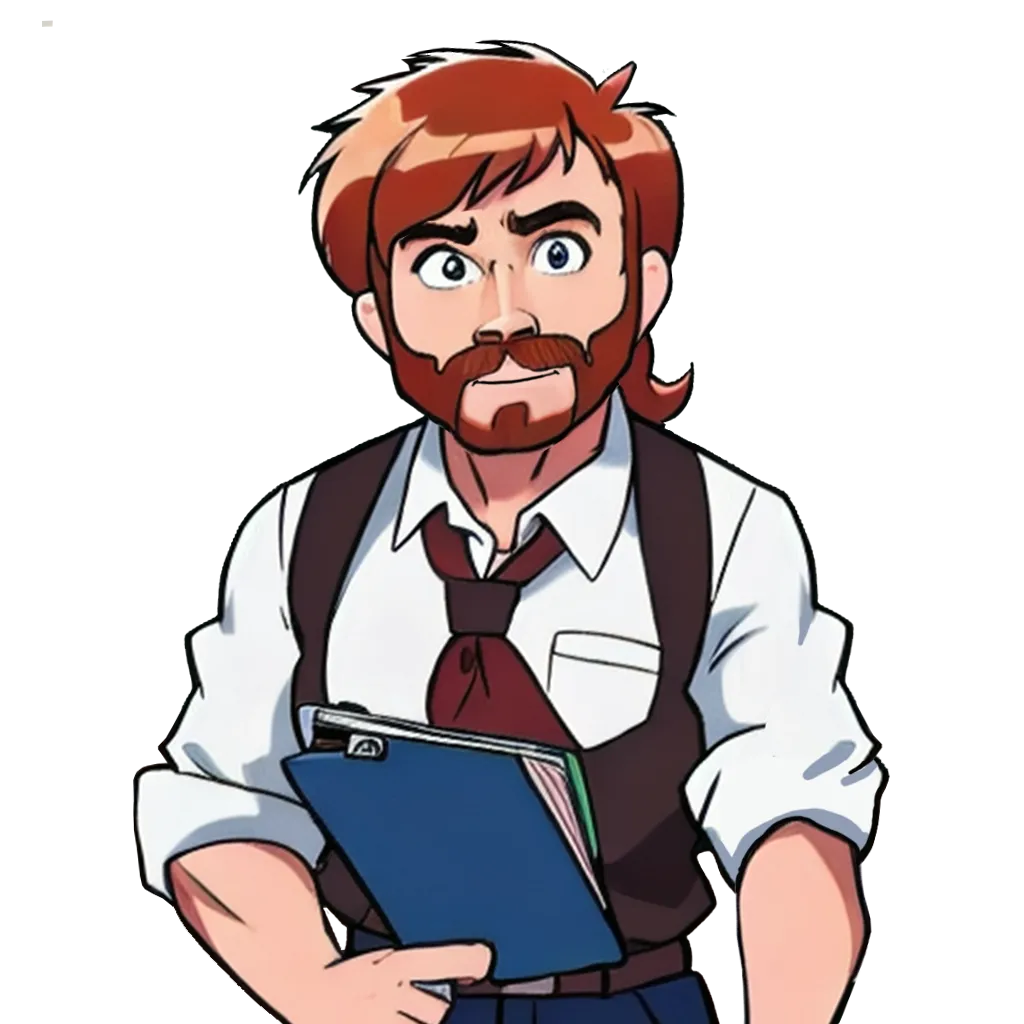