Express JS
Authentication and Authorization in Express
Authentication and authorization are two fundamental aspects to ensure the security of any web application. Authentication is the process of verifying a user's identity, while authorization refers to the permissions that user has within the application. In this chapter, we will learn how to implement both concepts in Express using different methods and tools.
Basic Authentication
To begin, let's look at an example of how to implement basic authentication in Express using sessions. We will use the express-session
package to handle user sessions.
Installing express-session
First, install the necessary package:
bash
Then, we configure the session middleware in our project.
javascript
Verifying Sessions
Next, we will see how to create protected routes that only allow access to authenticated users.
javascript
Authentication with JWT (JSON Web Tokens)
For modern applications, it is common to use JSON Web Tokens (JWT) for authentication, especially in APIs. JWTs allow user credentials to be securely passed between the client and server without needing to maintain sessions on the server.
Installing jsonwebtoken
First, install the necessary package to work with JWT:
bash
Generating and Verifying Tokens
Next, we will see how to generate a JWT token when a user logs in and how to verify it in protected routes.
javascript
Authorization of Routes
In addition to authenticating users, we can restrict access to certain routes based on the user's role or permissions.
javascript
Best Practices in Authentication and Authorization
- Store Tokens Securely: JWT tokens should be stored securely on the client, preferably in
localStorage
orsessionStorage
. - HTTPS Mandatory: Always use HTTPS to protect credentials and tokens from being intercepted.
- Token Expiration: Set an expiration for JWT tokens and provide a token renewal mechanism if necessary.
- Roles and Permissions: Implement clear logic for roles and permissions for different users in your application.
Conclusion
In this chapter, we have seen how to implement authentication and authorization in Express using both sessions and JWT. These are essential components for the security of any web application.
- Introduction to Express JS
- Express Fundamentals
- Request and Response Management
- Estructura de Proyectos en Express
- Authentication and Authorization in Express
- Connecting Express with Databases
- Error Handling and Logging in Express
- Sending Emails in Express
- Security in Express Applications
- Advanced Middleware in Express
- Creating REST APIs with Express
- WebSocket Implementation in Express
- Implementation of Webhooks in Express
- Testing Express Applications
- Deployment of Express Applications
- Performance Optimization in Express
- Monitoring and Maintenance of Express Applications
- Best Practices and Scalability in Express
- Course Conclusion: Express JS
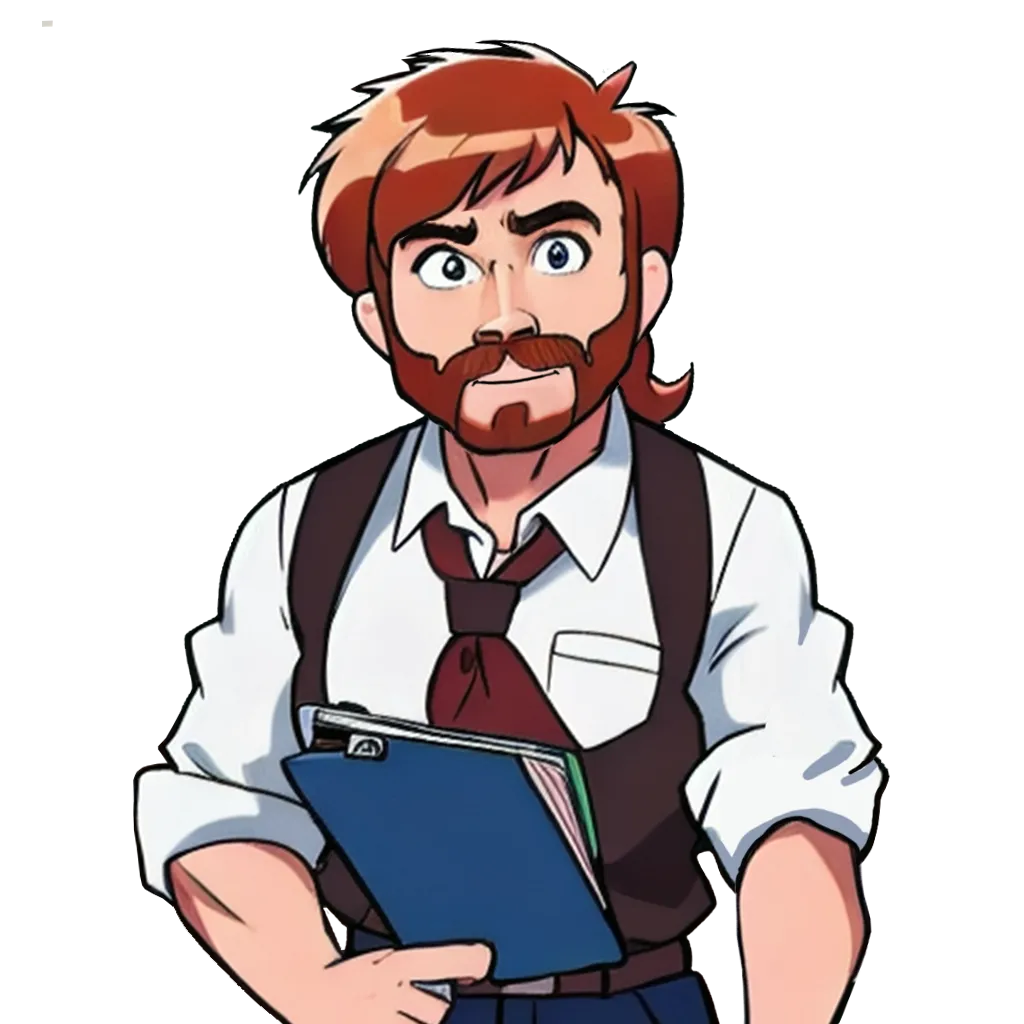