Next.js
Authentication and Authorization in the App Router
Authentication and authorization are key components in any modern web application. In this chapter, we will learn how to implement authentication and authorization in a Next.js 13 application using the App Router. We will see how to protect routes and efficiently manage user sessions.
Authentication with Next.js
To implement authentication, we can use several libraries. One of the most common is NextAuth.js, which offers a ready-made solution to handle different authentication providers like Google, GitHub, among others.
Configuring NextAuth.js
First, we need to install NextAuth.js in our project:
bash
After installing the library, we can configure the authentication provider. Below is how to configure NextAuth.js to use authentication with Google.
javascript
In this case, we have set up the Google provider and created the API to handle authentication. Remember to store the credentials in environment variables.
Protecting a Route
To protect a route, we can use the useSession
hook that NextAuth.js provides to verify if the user is authenticated. If the user is not authenticated, we can redirect them to the login page.
javascript
This approach is ideal for protecting routes that should only be accessible to authenticated users.
Role-Based Authorization
Authorization goes a step beyond authentication, as it involves checking if the user has the necessary permissions to access certain resources. For example, we might have an application with different user roles, such as "admin" and "user", and only allow administrators to access certain routes.
javascript
This example demonstrates how to implement role-based authorization, ensuring that only authorized users can access certain pages.
Session Management
NextAuth.js automatically manages sessions, but you can also customize their duration and behavior. For example, you can define the session lifespan in the configuration file:
javascript
Such configurations allow you to tailor the user experience and ensure session security.
Conclusion
Implementing authentication and authorization in Next.js 13 using the App Router is a relatively straightforward process thanks to tools like NextAuth.js. In this chapter, we have seen how to protect routes, manage user roles, and handle sessions. These concepts are essential to ensure that only authorized users can access the private parts of the application.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
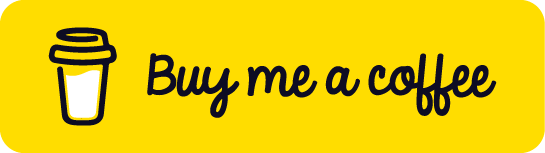
Chat with Chuck
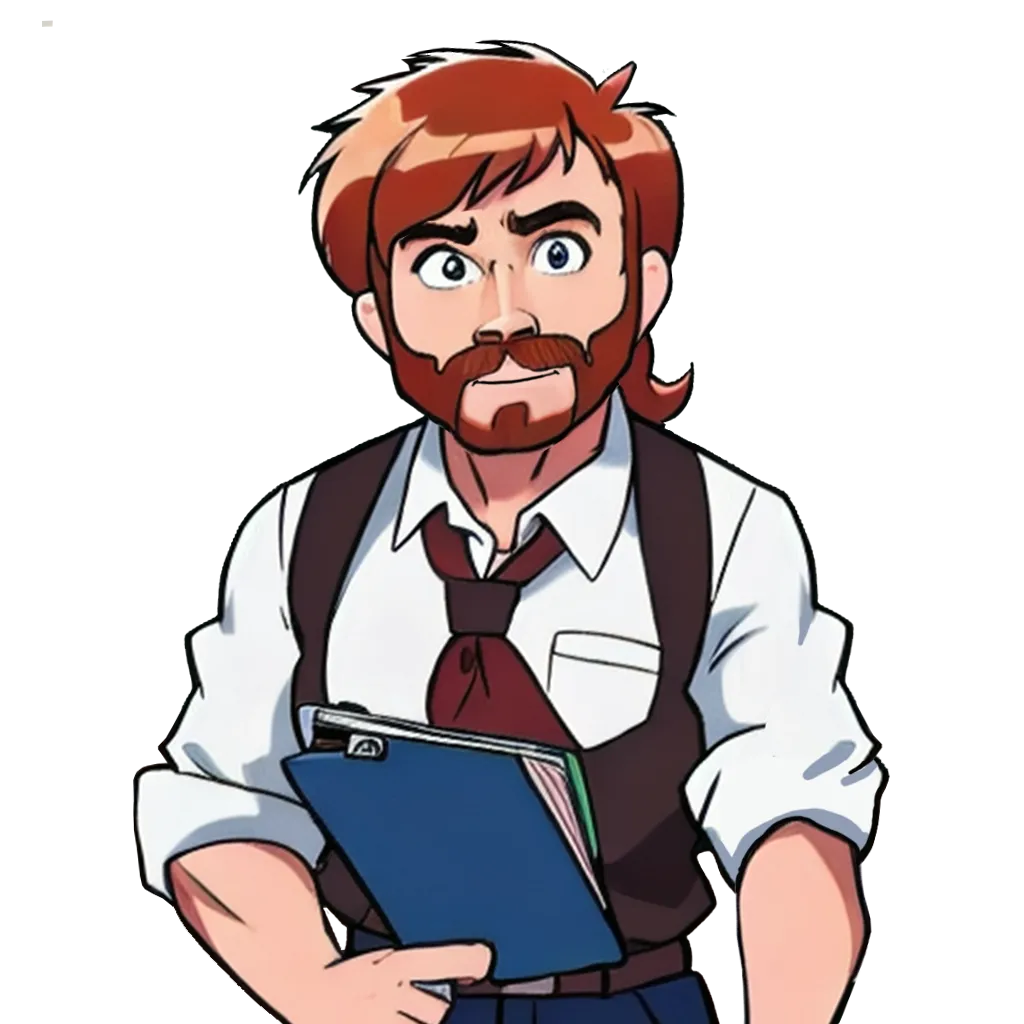
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13