Next.js
Optimization and Performance in Next.js 13 (App Router)
Performance optimization is crucial to ensure that our applications are fast, efficient, and provide a smooth user experience. In this chapter, we will learn some best practices for optimizing a Next.js 13 application using the App Router, taking advantage of the built-in tools that Next.js provides.
Rendering Optimization
Proper Use of SSR, SSG, and CSR
One of the first steps to optimize a Next.js application is to choose the correct rendering method for each page. We have already seen the differences between Server-Side Rendering (SSR), Static Site Generation (SSG), and Client-Side Rendering (CSR) in previous chapters. Using the appropriate approach is key to optimizing performance:
- SSR: Ideal for pages where data changes frequently and it is crucial that they are always updated.
- SSG: Useful for pages whose content does not change frequently and where loading speed is a priority.
- CSR: Suitable for dynamic interactions that do not require server-side pre-rendering.
An example where we might choose SSG to optimize the speed of a page:
javascript
By using SSG with Incremental Static Regeneration (ISR), we can ensure that our static pages regenerate periodically to keep the content updated without affecting performance.
Image Loading Optimization
One of the most useful features of Next.js is its image optimization system. Next.js offers the Image component that helps load images in an optimized and adaptive way.
javascript
The Image
component includes optimizations such as lazy loading by default for non-critical images, support for different image formats, and automatic generation of adaptive sizes.
Code Splitting
Next.js facilitates code splitting into smaller chunks that are only loaded when needed. This improves the initial load time of the page.
Dynamic Component Loading
We can use the dynamic()
function from Next.js to dynamically load components, which helps reduce the size of the initial JavaScript sent to the client.
javascript
Dynamic loading is useful for components that are not essential at the initial render, thus improving the application's performance.
Bundle Optimization with Package Analysis
Next.js includes tools that allow analyzing the size of the generated bundle, facilitating the identification of unnecessary or heavy dependencies. We can enable bundle analysis by adding a configuration in the next.config.js
file:
javascript
By using this tool, we can identify potential improvements and remove unnecessary code that may be affecting our application's performance.
Minification and Compression
Next.js automatically handles minification of JavaScript and CSS files as well as Gzip compression to improve the speed of data transfer to the client. Ensure that these options are enabled in Next.js configuration:
javascript
Caching and Cache Control
Next.js offers native support for cache management, allowing pages or data to be stored in the browser's cache or intermediate servers. You can configure custom cache strategies to improve the application's performance, especially when using SSG or SSR.
javascript
This ensures that pages stay up-to-date while still benefiting from cache to enhance performance.
Conclusion
Optimizing a Next.js 13 application requires a careful approach to balance loading speed, bundle size, and data management. In this chapter, we have seen how to choose the best rendering strategies, optimize images, split code, and manage cache to achieve faster and more efficient applications.
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13
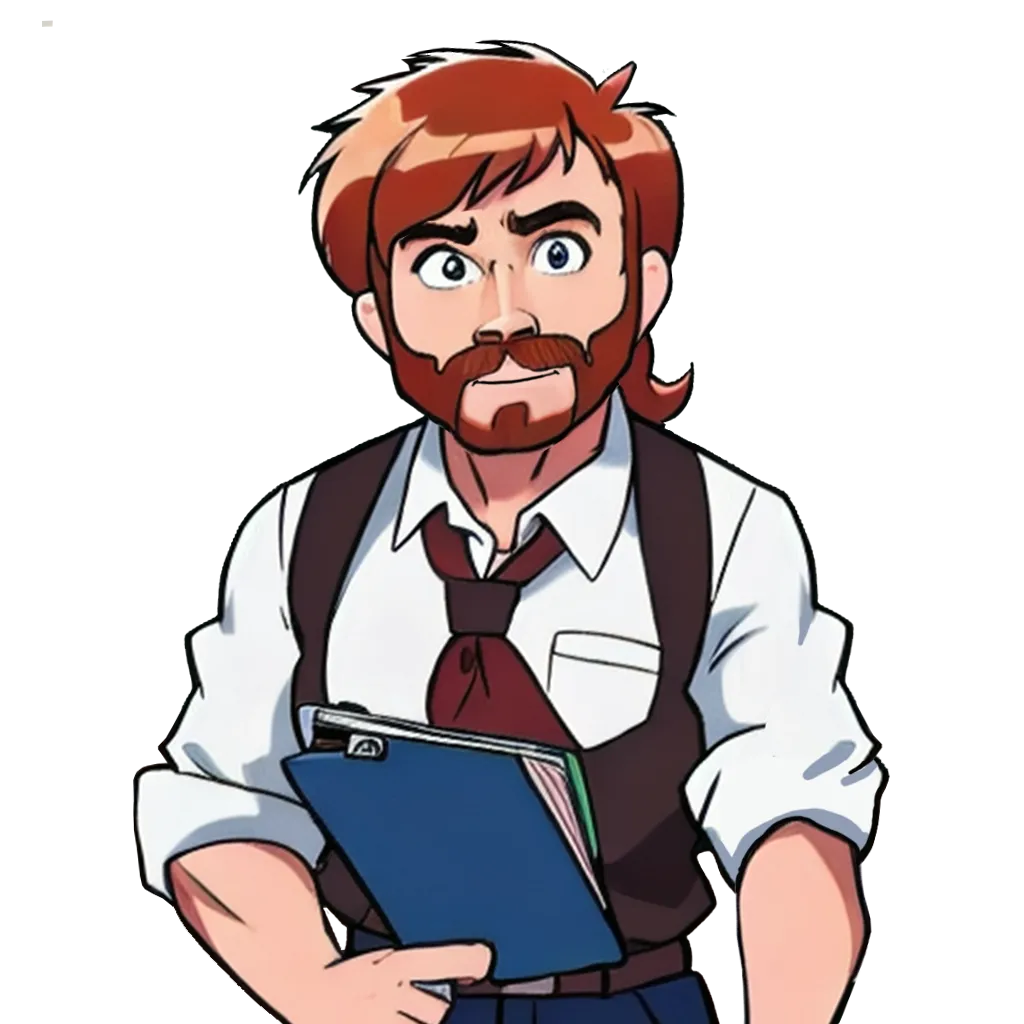