Next.js
Pages and Routes in the App Router
In this chapter, we will focus on how to create and manage routes and pages within the App Router of Next.js 13. One of the most important aspects of any web application is its navigation system, and the App Router provides an intuitive way to organize and define routes.
Static Routes
Static routes are those that do not change and are fixed within the application. In Next.js, creating a static route is as simple as defining a page.js
file within a folder corresponding to the route. For example, to create a static route for a "contact" page, we can do it as follows:
javascript
In this case, the static route /contact
corresponds to the contact/
folder within app/
, and the page is defined in the page.js
file.
Dynamic Routes
In more complex applications, it's common to need dynamic routes, where part of the URL changes depending on the data being displayed. Next.js's App Router allows handling dynamic routes by using brackets in folder names.
For example, if we want to create a profile page where the URL includes the username, we could do the following:
javascript
In this example, the folder [username]
indicates that the route is dynamic, and the username is captured from the URL. The value is passed to the page via the params
object.
Nested Routes
Another important concept in the App Router's routing system is the ability to create nested routes. Nested routes allow a main route to contain sub-routes within itself. This is useful for creating sections of a page that contain subsections.
Suppose we have a "blog" page that contains several individual entries:
javascript
In this case, /blog
is the main blog page, while /blog/[postId]
handles individual pages for each blog entry.
Handling 404 Routes
Next.js also allows creating a custom page to handle non-existent routes, that is, a 404 error page. To do this, simply create a not-found.js
file within the app/
folder.
javascript
This page will automatically be displayed when a user attempts to access a route that is not defined.
Conclusion
In this chapter, we have seen how to create and manage static, dynamic, nested routes, and how to handle non-existing routes using a 404 error page. The routing system in the App Router of Next.js 13 is powerful and flexible, making it easy to handle complex URLs in our applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
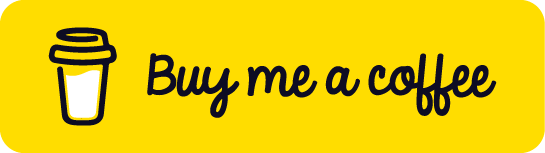
Chat with Chuck
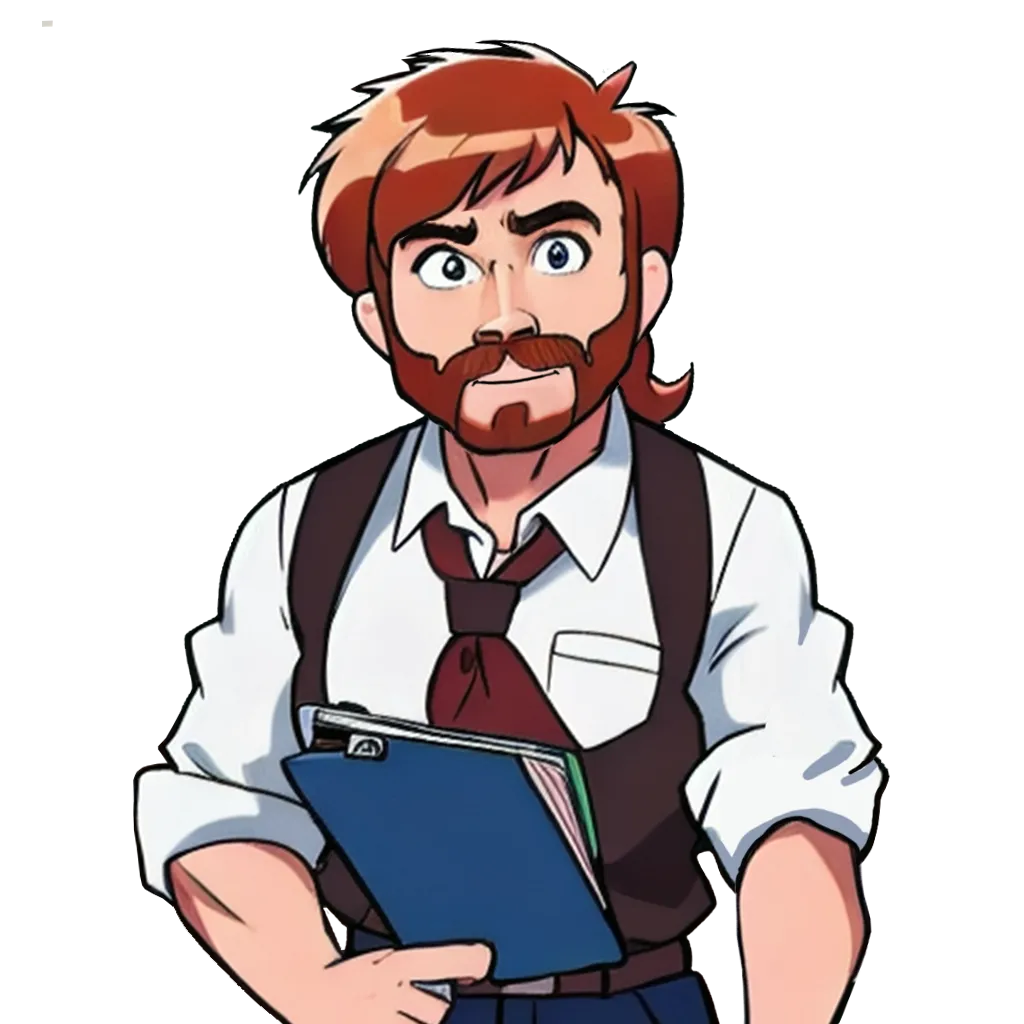
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13