Next.js
Pages with SSR and SSG in the Page Router
In this chapter, we will delve into how to use Server-Side Rendering (SSR) and Static Site Generation (SSG) in the Page Router of Next.js 13. These two techniques are essential for enhancing the performance and optimization of our web applications, allowing us to generate dynamic and static content as needed.
What is Server-Side Rendering (SSR)?
Server-Side Rendering (SSR) is a technique where pages are rendered on the server before being sent to the client. This ensures that the content is up-to-date and immediately visible to the user, which improves both SEO and the overall experience.
Implementing SSR with getServerSideProps
The getServerSideProps
function is the method used in Next.js to fetch data on the server before rendering the page. Each time a user accesses the page, a request is made to the server.
javascript
In this example, product data is fetched from the server each time the page is accessed, ensuring they are always up-to-date.
What is Static Site Generation (SSG)?
Static Site Generation (SSG) is a technique where pages are statically generated during the application's build time. This means that the pages are created once and then served quickly to all users. It is ideal for content that does not change frequently.
Implementing SSG with getStaticProps and getStaticPaths
When using SSG, we can generate static pages using the getStaticProps
and getStaticPaths
functions. This is useful for generating multiple dynamic pages with static content.
javascript
In this case, each blog post page is generated statically and automatically regenerated after a period of time to keep data updated.
Choosing between SSR and SSG
The choice between SSR and SSG depends on the application's needs:
-
SSR: Use SSR when you need data to always be up-to-date on each request, such as in personalized pages or where the data changes frequently.
-
SSG: Use SSG when pages have content that doesn't change frequently or when loading speed is a priority, such as in blogs or documentation sites.
Combining SSR and SSG
In some cases, you might want to combine both techniques in the same application. For example, you could use SSR for pages that require real-time updated data, like dashboards, and SSG for static pages like blog articles.
Performance Optimization with SSR and SSG
Both SSR and SSG improve the overall performance of the application in different ways:
-
SSR enhances the user experience by ensuring the content is always up-to-date but can increase the server response time.
-
SSG significantly improves initial loading speed by serving static pages but requires regenerating pages when data changes.
Enhancing User Experience
By combining SSR and SSG, we can offer a fast and smooth user experience. For example, static pages can load quickly while dynamic pages fetch updated content on the server.
Conclusion
Both SSR and SSG are powerful techniques in Next.js 13 that enable efficient page rendering management. In this chapter, we have seen how to implement these techniques using the Page Router and how to choose the right strategy depending on our application's needs.
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13
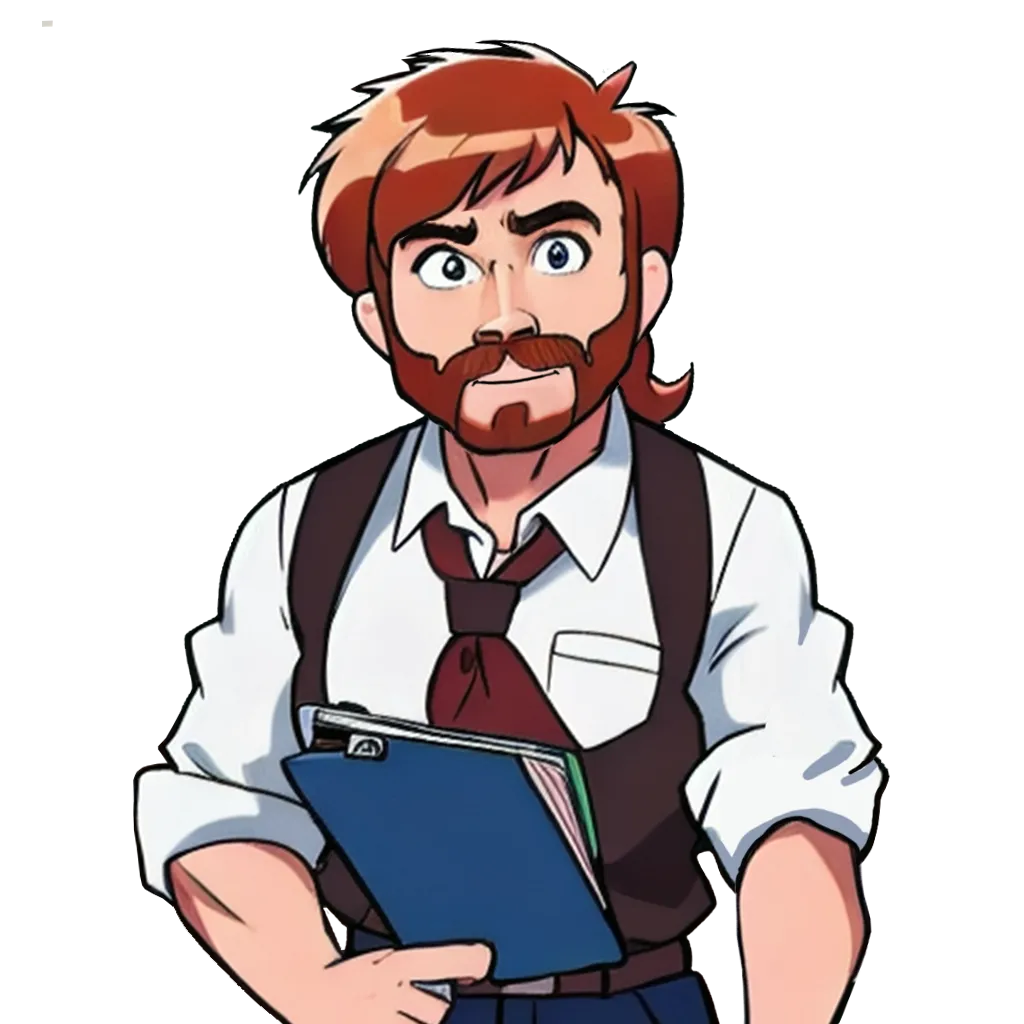