Next.js
Data Fetching in Next.js 13 with App Router
One of the most powerful features of Next.js is its ability to efficiently fetch data. The App Router in Next.js 13 facilitates handling Data Fetching from either the server or the client. In this chapter, we will learn how to fetch data using different strategies, such as Server-Side Rendering (SSR), Static Site Generation (SSG), and Client-Side Rendering (CSR).
Server-Side Rendering (SSR) with getServerSideProps
The getServerSideProps method allows fetching data on the server every time a user accesses a page. This ensures that information is always up-to-date and is useful for pages where data frequently changes.
javascript
SSR is ideal for situations where data needs to be real-time or when data depends on the user's request.
Static Site Generation (SSG) with getStaticProps
Static Site Generation (SSG) is a method where pages are generated statically at build time, allowing for fast load times. Next.js allows the use of getStaticProps
to generate these pages, and along with Incremental Static Regeneration (ISR), we can regenerate static pages periodically.
javascript
The use of SSG is recommended for pages where content doesn’t change frequently and where fast load times are essential.
Client-Side Rendering (CSR)
Client-Side Rendering (CSR) is useful for fetching dynamic data after the page has already been rendered on the client. This is achieved through API calls from the browser. CSR is especially useful for interactions that require real-time data updates, such as product search or content filters.
javascript
CSR is ideal for cases where the page content needs to be updated based on user interactions without reloading the page.
Hybrid Data Fetching
One of the most powerful features of Next.js is its ability to combine different data fetching strategies. You can use SSR to fetch data on the server and CSR to update those data in real-time on the client.
javascript
This hybrid approach offers a more fluid user experience by combining the best of SSR and CSR.
Conclusion
The App Router in Next.js 13 offers incredible flexibility for fetching and handling data. We can choose between Server-Side Rendering, Static Site Generation, Client-Side Rendering, or combine them according to the needs of our application. This allows us to optimize both performance and user experience.
In the next chapter, we will explore how to implement Authentication and Authorization in our Next.js applications using the App Router.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
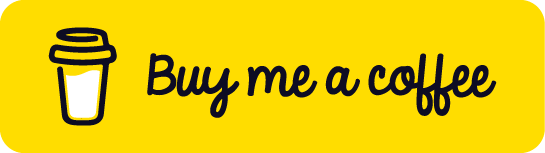
Chat with Chuck
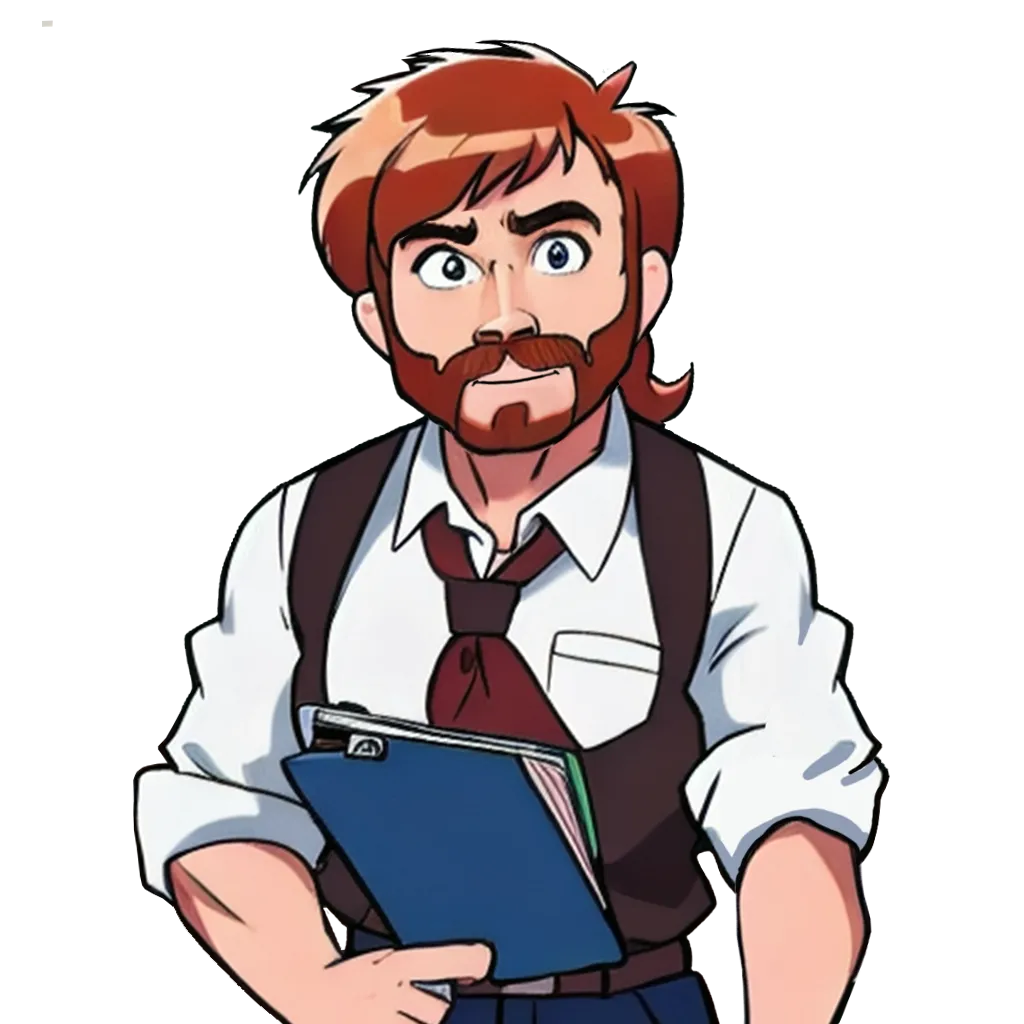
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13