Next.js
Routes and Navigation in the Page Router
In this chapter, we will dive deeper into how to manage routes and navigation using the Page Router in Next.js 13. We'll explore how to work with static, dynamic, and nested routes, as well as how to implement navigation within an application using the tools provided by Next.js.
Static Routes
Static routes in Next.js are simple and straightforward. As we mentioned in the previous chapter, each file inside the pages/
folder represents a route in the application. Here is a basic example of a static page handling the /about
route:
javascript
This about.js
file creates a static page accessible from the URL /about
without any additional configuration.
Dynamic Routes
In the Next.js Page Router, dynamic routes are handled by creating files whose names are enclosed in square brackets []
. These files allow part of the URL to be dynamic and captured as a parameter.
For example, if we want to create a profile page for different users, we can create a file named [username].js
in the pages/profile/
folder:
javascript
Here, params.username
is captured directly from the URL. If the user visits /profile/john
, john
will be passed as the value of params.username
.
Nested Routes
The Page Router also allows handling nested routes. Nested routes are used when a section of an application has its own sub-routes.
Suppose we want to create a "blog" page that contains a list of posts and, within each post, a dynamic route to display the content of that post. We can organize the routes as follows:
javascript
The /blog
route shows a list of posts, and the sub-routes /blog/1
, /blog/2
, etc., will handle the specific content of each post.
Navigation in the Page Router
To handle navigation between routes, Next.js uses the Link
component, which allows users to move from one page to another without reloading the entire application.
Navigation Example
Let's add a navigation component to our application:
javascript
The Link
component replaces traditional <a>
tags to enable reload-free navigation, which enhances the user experience by making navigation smoother.
404 Route Handling
Next.js allows handling non-existing routes by creating a custom 404 error page. To do this, simply create a file named 404.js
in the pages/
folder.
javascript
The 404 page will automatically be displayed when a user accesses a URL not defined in the application's routes.
Redirects in the Page Router
In Next.js, we can manage redirects using the getServerSideProps
function or by configuring redirects directly in the next.config.js
file.
Redirection with getServerSideProps
Here is an example of redirecting the user to another page using getServerSideProps
:
javascript
This redirection is useful when you want to move pages or change URLs without breaking the user experience.
Conclusion
The Page Router in Next.js 13 offers a clear and straightforward way to manage static, dynamic, and nested routes. With the use of the Link
component for navigation, and features like redirects and 404 route handling, we can efficiently build complete applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
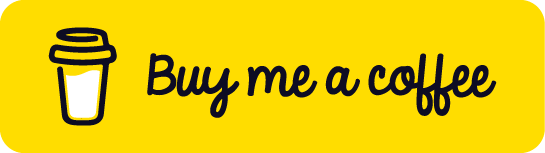
Chat with Chuck
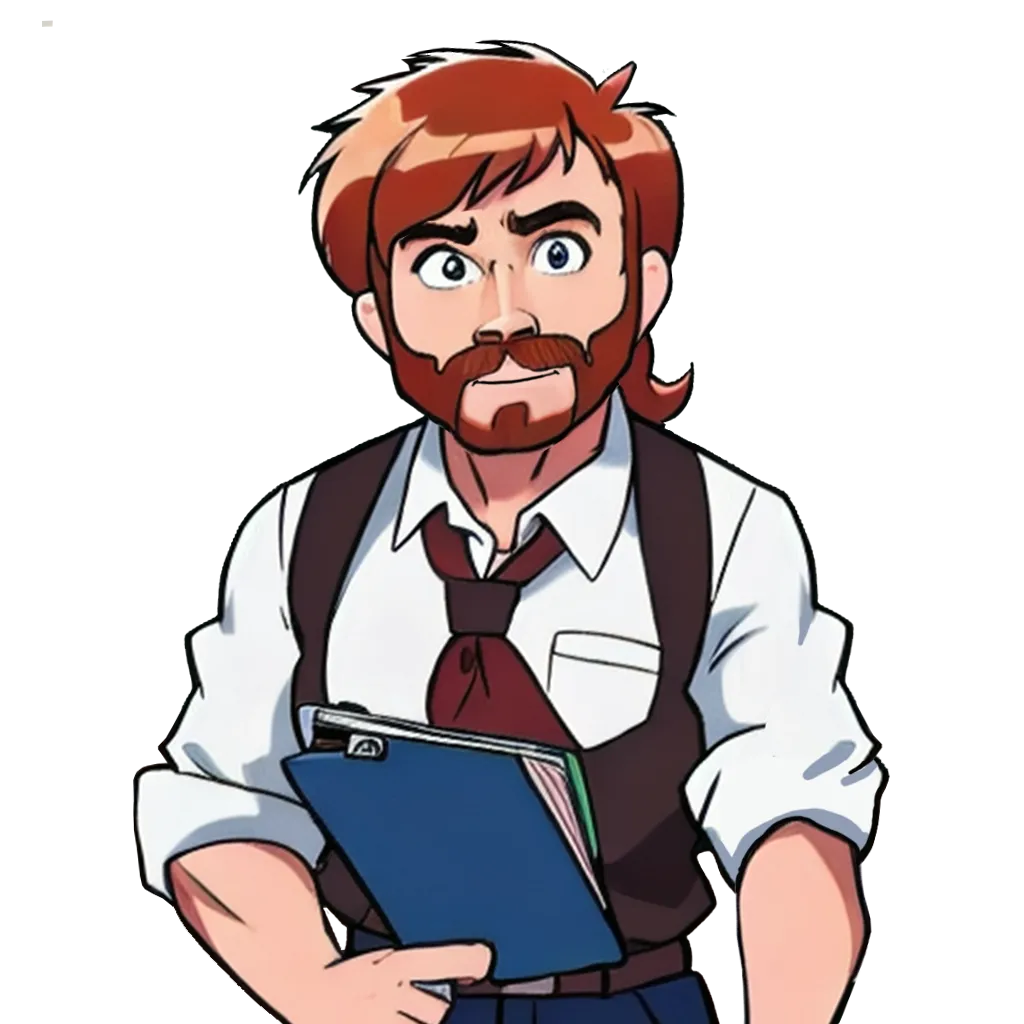
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13