Next.js
Redirects and Rewrites in the Page Router
In this chapter, we will explore how to implement redirects and rewrites in a Next.js 13 application using the Page Router. These techniques are useful for controlling how users and search engines access our routes, allowing us to redirect traffic from one URL to another or rewrite routes transparently.
Redirects in Next.js
Redirects allow you to send users from one URL to another. This is useful when you change the route structure of your application or when you want to redirect traffic from an old URL to a new one.
Implementing Redirects in next.config.js
In Next.js, you can configure redirects directly in the next.config.js
file. Let's see how to implement a simple redirect.
javascript
With this configuration, any request to /old-page
will automatically redirect to /new-page
.
Types of Redirects
- Permanent Redirect (301): Used when the original URL has been permanently moved to a new URL. Search engines will update their indices to reflect the new URL.
- Temporary Redirect (302): Used when the change is temporary. Search engines will not update their indices.
Temporary Redirect Example
javascript
Rewrites in Next.js
Rewrites allow you to modify routes visible to the user without changing the URL in the browser. While redirects send the user to a new URL, rewrites maintain the original URL while serving different content.
Implementing Rewrites in next.config.js
Like redirects, rewrites are configured in the next.config.js
file. Here is an example of how to rewrite a route:
javascript
In this case, users can view and navigate via the URL /blog/:slug
, but the content is fetched from /posts/:slug
. This is useful to keep URLs clean or user-friendly while serving content from a different internal structure.
Complete Example: Redirects and Rewrites
We can combine redirects and rewrites in the same next.config.js
file to have complete control over our application's routes.
javascript
Using Parameters in Rewrites
Rewrites also allow the use of dynamic parameters, which is useful when handling dynamic routes. Here's an example:
javascript
Best Practices for Redirects and Rewrites
-
Use permanent redirects when necessary: Use permanent (301) redirects to move content definitively. This is beneficial for SEO.
-
Keep URLs clean with rewrites: Use rewrites to keep visible URLs as clean and descriptive as possible while organizing internal routes as needed.
-
Avoid too many redirects: Having many chained redirects can affect application performance and user experience. Try to minimize unnecessary redirects.
Conclusion
Using redirects and rewrites in Next.js 13 with the Page Router allows you to fully control your application's URL structure, enhancing both user experience and SEO. Redirects are useful for moving traffic from one URL to another, while rewires allow you to change how routes are handled without changing the visible URL.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
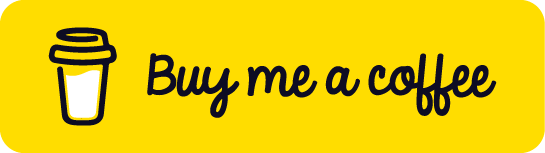
Chat with Chuck
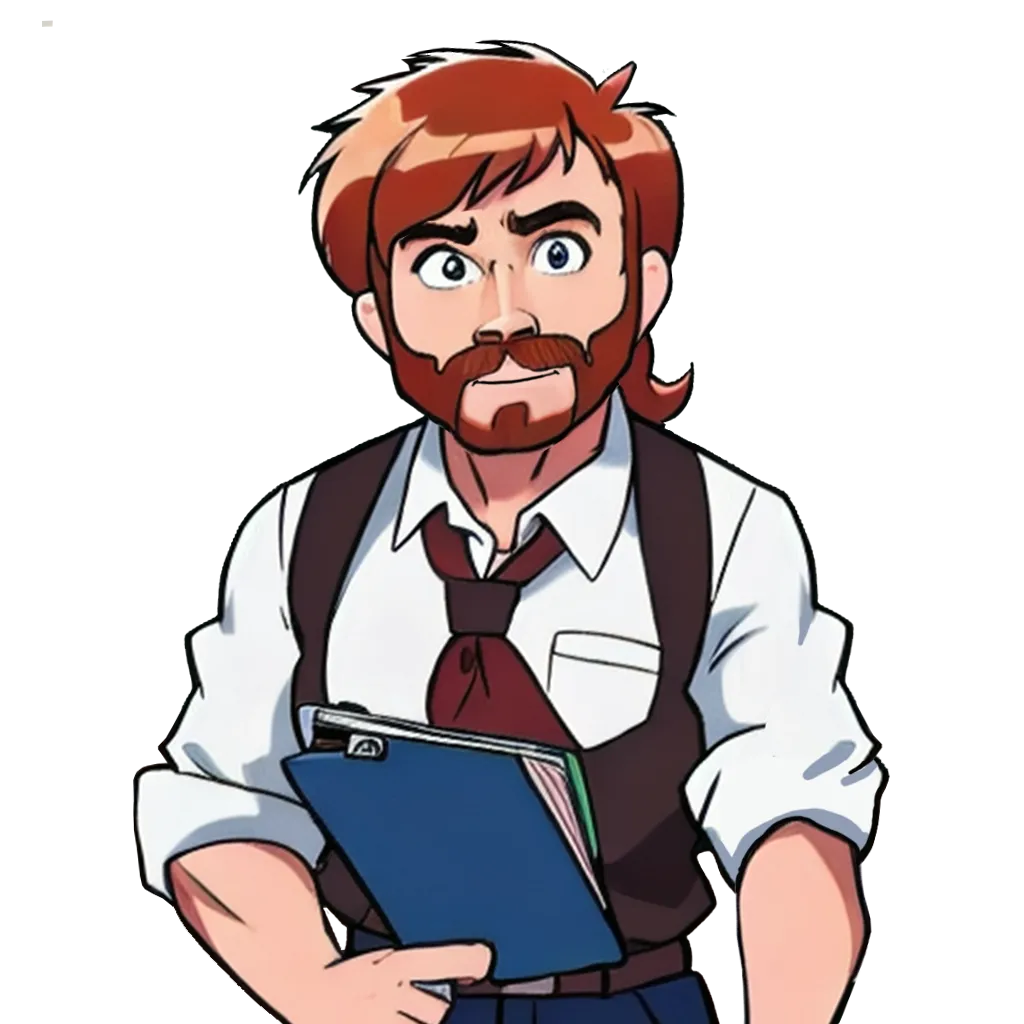
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13