Next.js
Deployment and Best Practices in Next.js 13
In this chapter, we will learn how to deploy a Next.js 13 application and review some best practices that we should follow to ensure a successful deployment and optimal performance in production.
Preparation for Deployment
Before deploying a Next.js application, it's essential to ensure everything is optimized and ready for production. Here are some key steps you should follow.
Verify the Production Environment
Make sure the production environment is correctly configured. Next.js uses the NODE_ENV
variable to differentiate between development and production environments.
bash
The command npm run build
generates optimized static files for production, minimizing JavaScript and CSS files and setting up page prerendering as needed.
Optimize Images and Resources
Next.js supports automatic image optimization using the Image
component. Ensure that all images in the application use this component to take advantage of size and format optimization for images in production.
javascript
Deployment on Vercel
The most common and recommended method for deploying Next.js applications is using Vercel, the platform where Next.js was built. Vercel offers native integration with Next.js, simplifying the deployment process.
Steps to Deploy on Vercel
-
Create an Account on Vercel: If you don't have an account, sign up at vercel.com.
-
Connect the Repository: Vercel allows you to connect your GitHub, GitLab, or Bitbucket repository. Simply select your Next.js project's repository.
-
Configure the Project: During the configuration process, Vercel automatically detects that you are using Next.js and suggests the appropriate deployment configuration.
-
Deploy: Once the configuration is complete, Vercel automatically deploys your project and creates a URL so you can view your application in production.
Vercel also offers the option to create custom domains and efficiently manage deployments, providing support for SSR, SSG, and ISR applications.
Deployment on Other Servers
In addition to Vercel, Next.js can be deployed on other servers and platforms, such as Netlify, Heroku, or any server that supports Node.js.
Deployment on a Node.js Server
If you prefer to deploy your Next.js application on a Node.js server, you can do so by following these steps:
- Build the application for production:
bash
- Run the production server:
bash
In this case, the application will run as a traditional Node.js server, handling both Server-Side Rendering (SSR) and pages generated with Static Site Generation (SSG).
Deployment on Netlify or Heroku
Both Netlify and Heroku offer support for deploying Next.js applications. The deployment process is similar to Vercel, connecting your repository and allowing the platform to handle deployments.
-
Netlify: Allows deployment of applications with SSG and supports lambda functions for handling SSR.
-
Heroku: Requires configuring a
Procfile
to specify how to run the Next.js application in production.
Best Practices in Deployment
1. Enable Compression
To improve performance in production, ensure you enable file compression using gzip
or Brotli
. Next.js already includes compression by default, but you can configure your server to ensure all resources are served compressed.
2. Implement Redirects and Rewrites
If you've changed the routes of your application during development, ensure you implement redirects and rewrites in production to avoid 404 errors.
javascript
3. Monitor Performance
Once the application is deployed, it's essential to monitor performance to detect load issues or failures in production. Tools like Vercel Analytics allow you to monitor your application's usage and performance in real time.
4. Use Incremental Static Regeneration (ISR)
To improve performance and ensure pages are automatically updated, use ISR on your static pages.
javascript
ISR is a powerful technique to keep static content updated without compromising application performance.
Conclusion
Deploying Next.js 13 applications is a straightforward and efficient process, especially when using a platform like Vercel, which is optimized for Next.js. By following best practices, such as enabling compression, using redirects, and monitoring performance, you can ensure your application performs optimally in production.
This has been the last chapter of the course on Next.js 13. Congratulations on completing the course! You now have all the tools needed to develop, deploy, and maintain modern and scalable Next.js applications. Keep building and exploring the world of web development!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
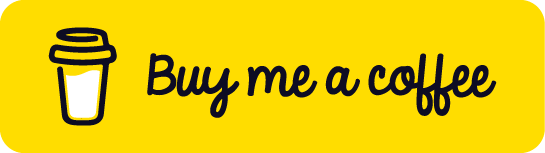
Chat with Chuck
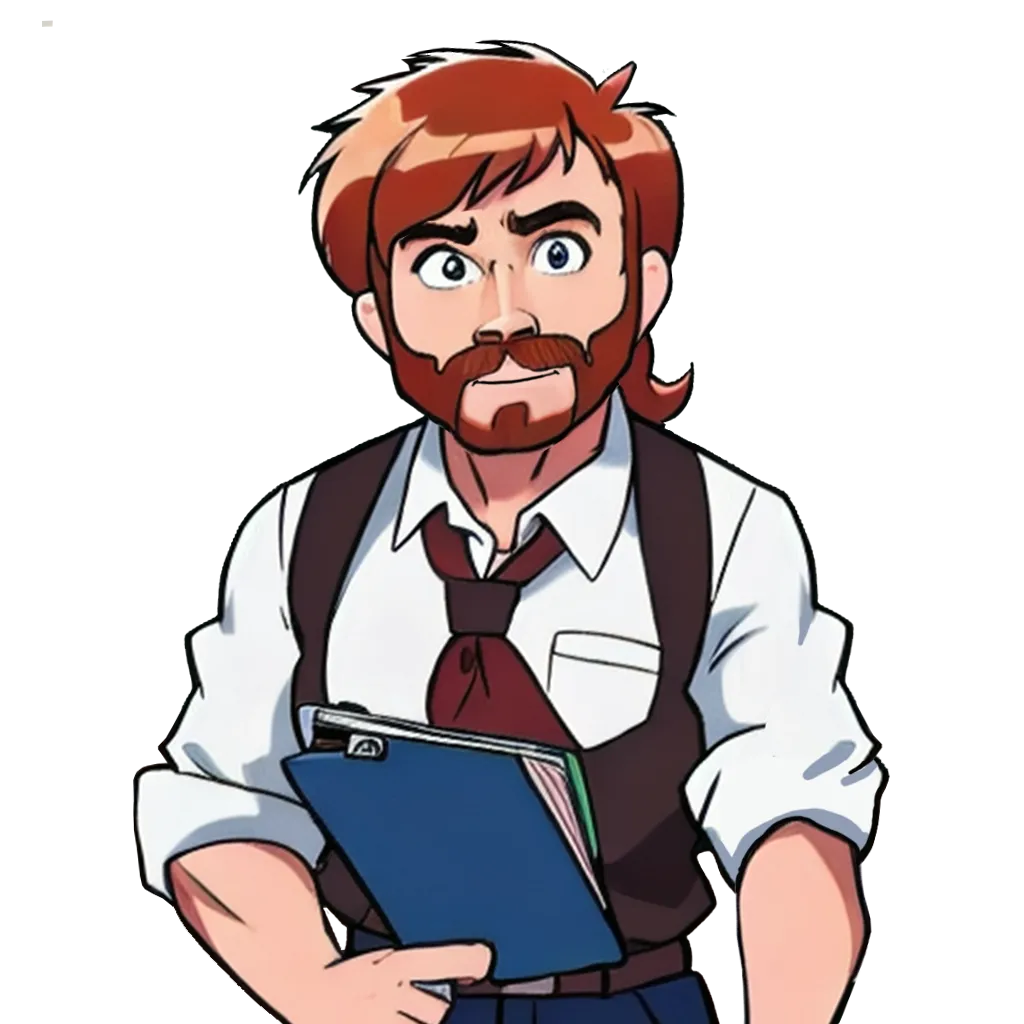
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13