Next.js
Project Structure in Next.js with App Router
In this chapter, we will explore how a project is organized in Next.js using the App Router. The correct folder and file structure is essential for keeping the code organized and facilitating its scalability as the project grows. Next.js 13 introduces a new structure that allows managing routes, layouts, and components more efficiently.
Basic Structure of a Project in Next.js
The structure of a project with the App Router follows a clear pattern. Below is an example of the typical organization of folders:
bash
Breakdown of Main Folders
-
app/: This is where the routing logic lives. Within this folder, you can create folders corresponding to your application routes, and in each, a
page.js
file that defines the corresponding page. -
public/: This folder contains publicly accessible static files, such as images, fonts, and other resources that do not go through the Next.js build process.
-
styles/: Folder where global styles or specific styles for different parts of the application are housed.
-
package.json: Configuration file that manages the project's dependencies and scripts.
-
next.config.js: Configuration file to customize Next.js's behavior.
Routes in Next.js 13
The routing system in Next.js's App Router is very intuitive. Each folder within app/
represents a route. For example, the about/
folder within app/
corresponds to the /about
route. Below is how to define a basic route:
javascript
As observed, Next.js automatically associates the route with the corresponding folder, simplifying route management.
Layouts in Next.js 13
One of the great benefits of the App Router is the ability to define layouts easily. Layouts are defined in layout.js
files and can be reused in multiple pages. A layout can contain common elements like headers or footers.
javascript
This layout will be applied to all routes within the app/
folder, making it easy to maintain presentation consistency throughout the application.
Additional Configuration in Next.js
Next.js allows additional configurations through the next.config.js
file. For example, it's possible to customize routing behavior, add performance optimizations, and more. Here is a basic example:
javascript
This configuration file is key to adjusting application behavior according to project needs.
Conclusion
The structure of a project in Next.js 13 with the App Router enables a clear and scalable organization, ideal for developing modern applications. In the upcoming chapters, we will delve into topics like data handling, creating dynamic routes, and optimizing our application.
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13
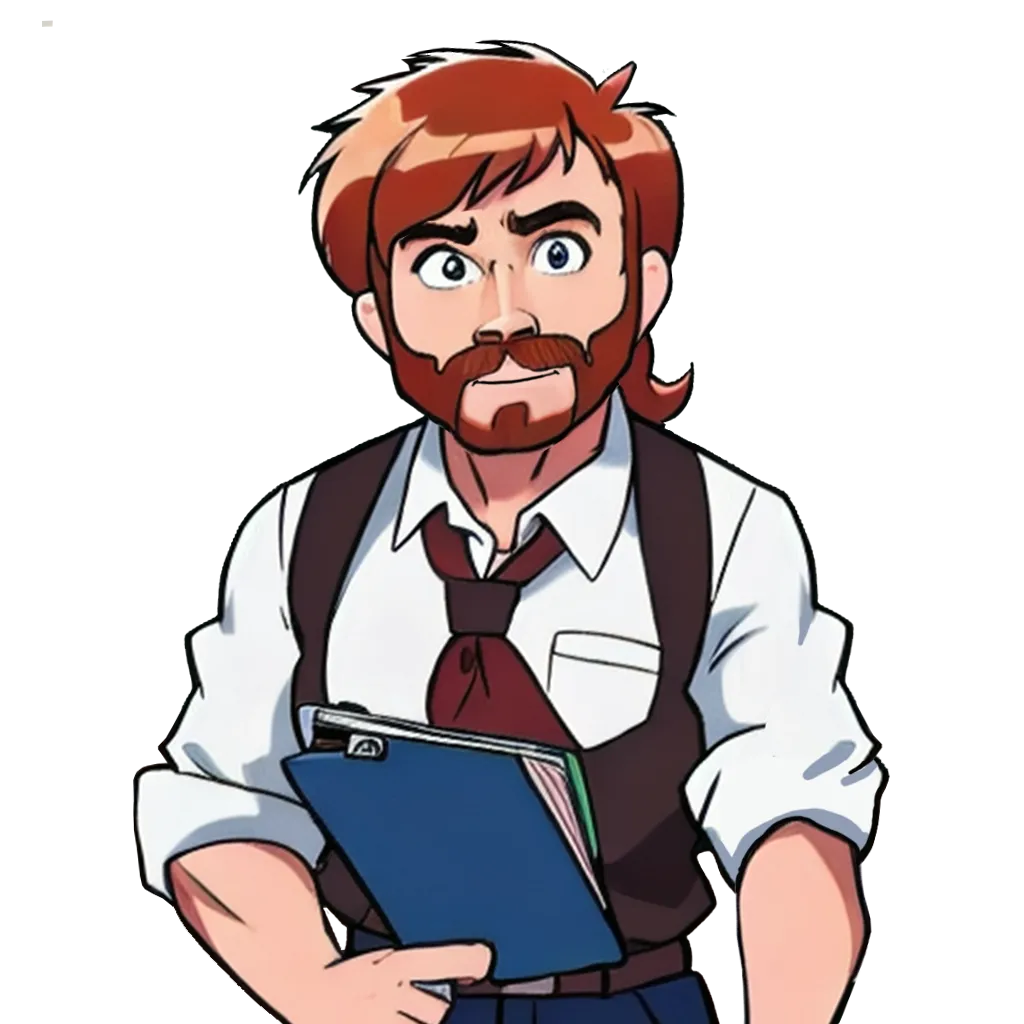