Next.js
Page Rendering and SSR in the App Router
In this chapter, we will explore how page rendering works in Next.js 13 and how Server-Side Rendering (SSR) is carried out. The App Router in Next.js 13 facilitates the handling of different rendering strategies, optimizing both performance and user experience.
What is Server-Side Rendering (SSR)?
Server-Side Rendering (SSR) is a method where pages are rendered on the server instead of the client (browser). This means that the full content of a page is generated on the server before being sent to the client, improving search engine optimization (SEO) and initial load speed.
In Next.js, SSR is handled via the getServerSideProps
function. This function allows you to fetch data on the server before rendering the page and sending it to the component to be displayed.
Basic example of SSR
Below is a basic example of how to implement SSR on a page:
javascript
In this case, every time a user visits a profile page, the data is fetched from the server, and the page is dynamically generated.
Static Rendering (SSG) with Incremental Static Regeneration (ISR)
In addition to SSR, Next.js also allows static rendering through the Static Site Generation (SSG) method. With SSG, pages are statically generated at build time, resulting in very fast load times.
In Next.js 13, we can combine SSG with Incremental Static Regeneration (ISR), which allows regenerating static pages after they have been built, based on certain time intervals or data changes.
Here's an example:
javascript
With ISR, we can benefit from the speed of SSG while keeping the data up-to-date without the need to recompile the entire application.
Client-Side Rendering (CSR)
Although SSR and SSG are fundamental in Next.js, we must not forget that we can also use Client-Side Rendering (CSR). In this case, dynamic content is rendered directly on the client, which is useful for certain interactions that do not require pre-rendering on the server.
javascript
Client-Side Rendering (CSR) is ideal for highly interactive functionalities that depend on user interaction, such as searches or filters.
Conclusion
Next.js 13 offers a very flexible approach to page rendering, allowing you to use SSR, SSG, ISR, and CSR as needed. This allows optimizing both user experience and application performance.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
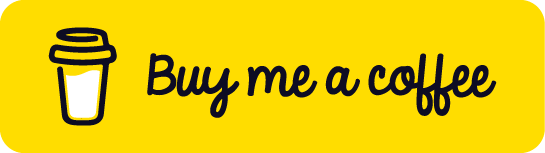
Chat with Chuck
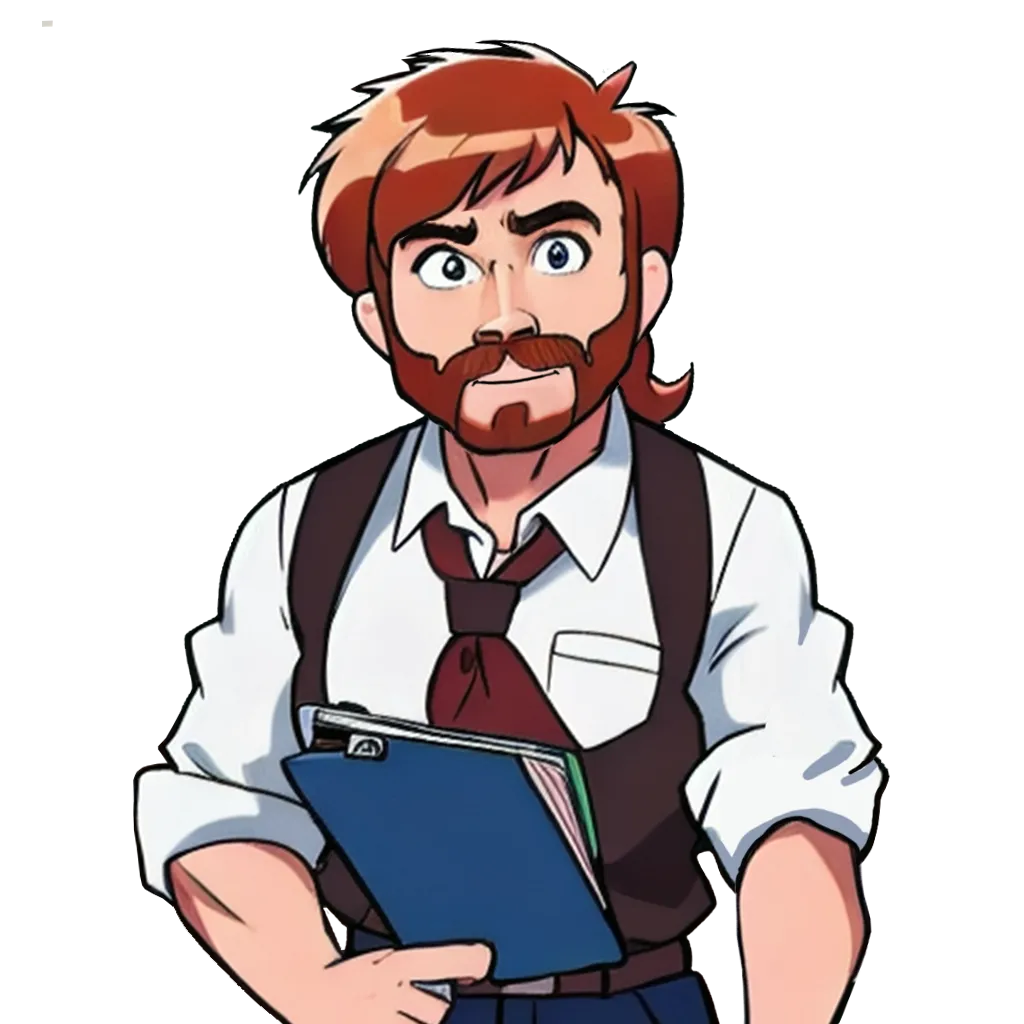
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13