Next.js
Using Layouts in the App Router
In Next.js 13, layouts have become an essential tool for structuring our applications, providing an efficient way to reuse parts of the interface, such as headers, menus, and footers, across multiple pages. In this chapter, we will learn how to use layouts in the App Router of Next.js.
What is a Layout?
A layout is a component used to share a common structure between different pages. This is useful when you have repetitive elements that need to be displayed on multiple routes, like a navigation bar or a footer.
In Next.js 13, you can define layouts at the route level to reuse components on pages under that route. For example, all pages within the app/
folder can share a global layout, while specific pages can have their own layout.
Basic Layout Example
Let's create a global layout that includes a header and a footer. This layout will apply to all pages of our application:
javascript
In this example, the content of each page is injected inside the main
container using the children
property. This allows each individual page to define its own content while sharing the same header and footer structure.
Route-Based Layouts
In addition to having a global layout, we can also define specific layouts for certain routes. For instance, if we want all pages under /blog
to share a different layout, we can do it as follows:
javascript
In this way, all pages under the app/blog/
folder will use this specific layout, while other routes will continue using the global layout.
Nested Layouts
Next.js 13 also allows creating nested layouts, which means you can have a global layout, and within certain routes, add secondary layouts that inherit from the main layout. This is useful for applications that have several sections with different structures but share common elements.
javascript
In this example, the global layout applies to all pages, while the specific dashboard layout adds an additional sidebar only for pages within dashboard/
. This modular approach allows great flexibility in organizing the user interface.
Rendering Layouts with SSR
Layouts in Next.js also work seamlessly with Server-Side Rendering (SSR), meaning they can be rendered on the server before being sent to the client. This is especially useful for elements that depend on real-time data.
We can combine getServerSideProps
with layouts as follows:
javascript
This approach allows customizing the layout based on data dynamically obtained from the server, enhancing the user experience.
Conclusion
Using layouts in Next.js 13 with the App Router allows creating well-structured and easy-to-maintain applications. Layouts can be global, route-based, or even nested, offering great flexibility for organizing the user interface. Additionally, they work seamlessly with server-side rendering, allowing fetching dynamic data before rendering the page.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
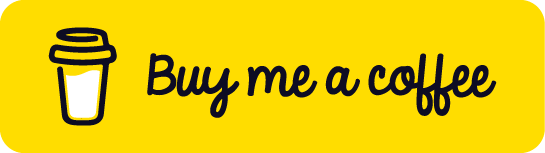
Chat with Chuck
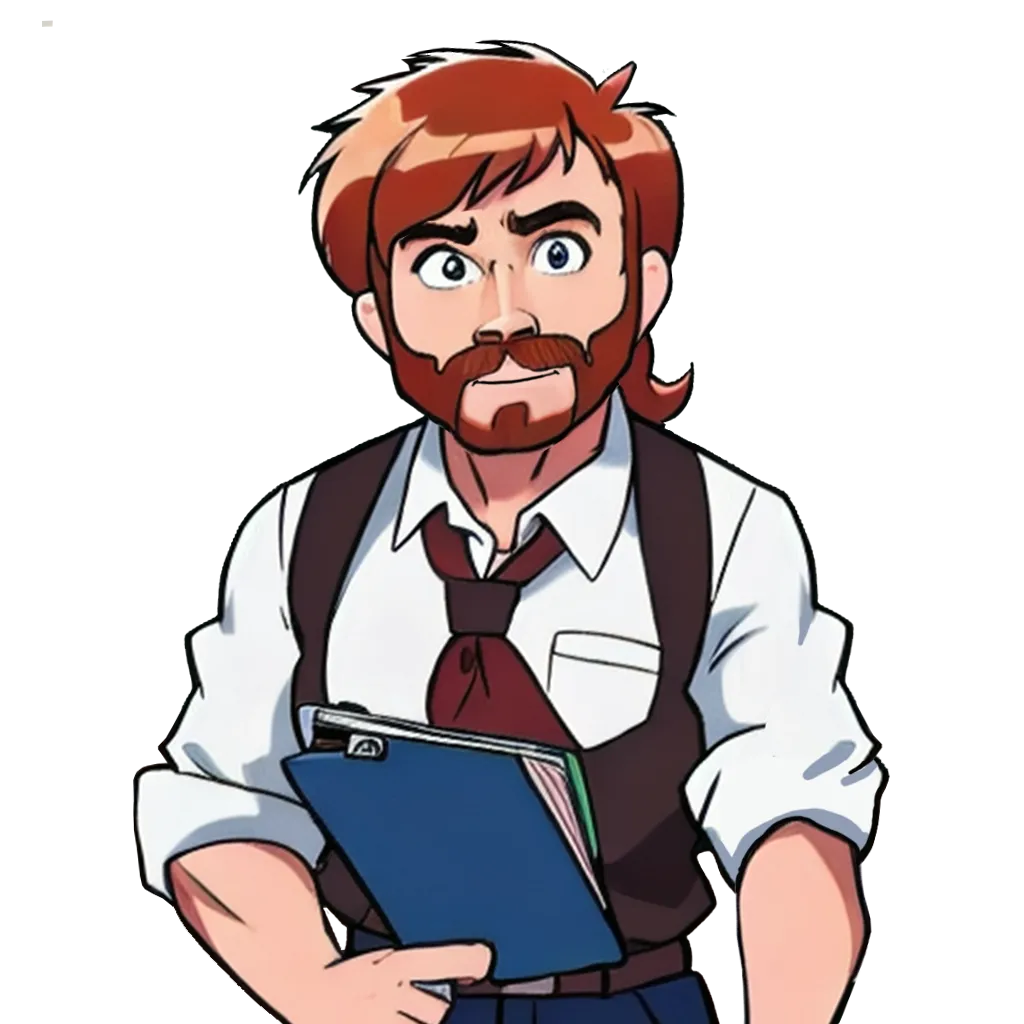
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13