Next.js
Data Fetching in the Page Router
In this chapter, we will focus on how to fetch data in a Next.js application using the Page Router. Next.js offers different ways to handle data fetching depending on the application's needs, such as Server-Side Rendering (SSR), Static Site Generation (SSG), and Client-Side Rendering (CSR).
Server-Side Rendering (SSR) with getServerSideProps
The getServerSideProps function is useful when we need to fetch data on every page request directly from the server. This ensures that the data is always up-to-date and available before the page is sent to the client.
SSR Example
javascript
Using SSR is ideal when the data changes frequently or is specific to the user making the request.
Static Site Generation (SSG) with getStaticProps
For pages that do not change frequently, we can use Static Site Generation (SSG), which allows generating the pages statically at build time. This improves loading speed by avoiding data fetching on every request.
SSG Example
javascript
Using SSG with Incremental Static Regeneration (ISR) allows regenerating static pages at defined time intervals, maintaining a balance between loading speed and updated data.
Client-Side Rendering (CSR)
When we need to fetch dynamic data on the client, we can use Client-Side Rendering (CSR). In this approach, data is fetched after the page has been rendered on the client through API calls from the browser.
CSR Example
javascript
CSR is ideal for cases where the content of the page depends on user interactions and does not require fetching data on the server.
Choosing the Right Data Fetching Strategy
- SSR: Use SSR when the data changes frequently and needs to be up-to-date on every request.
- SSG: Use SSG for pages whose content does not change frequently and where loading speed is essential.
- CSR: Use CSR for interactive features that depend on the user, such as search or content filters.
In many cases, you can combine several data fetching strategies within the same application, using SSR for some pages, SSG for others, and CSR for interactive functions.
Conclusion
The Page Router in Next.js 13 offers great flexibility for handling data fetching, allowing us to choose between SSR, SSG, and CSR depending on our application's needs. In this chapter, we have seen how to fetch data from both the server and client sides, and how to optimize the user experience using the appropriate strategy.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
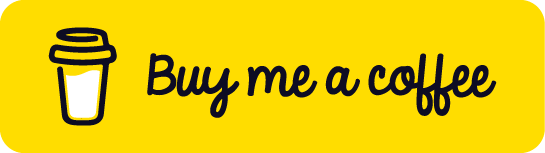
Chat with Chuck
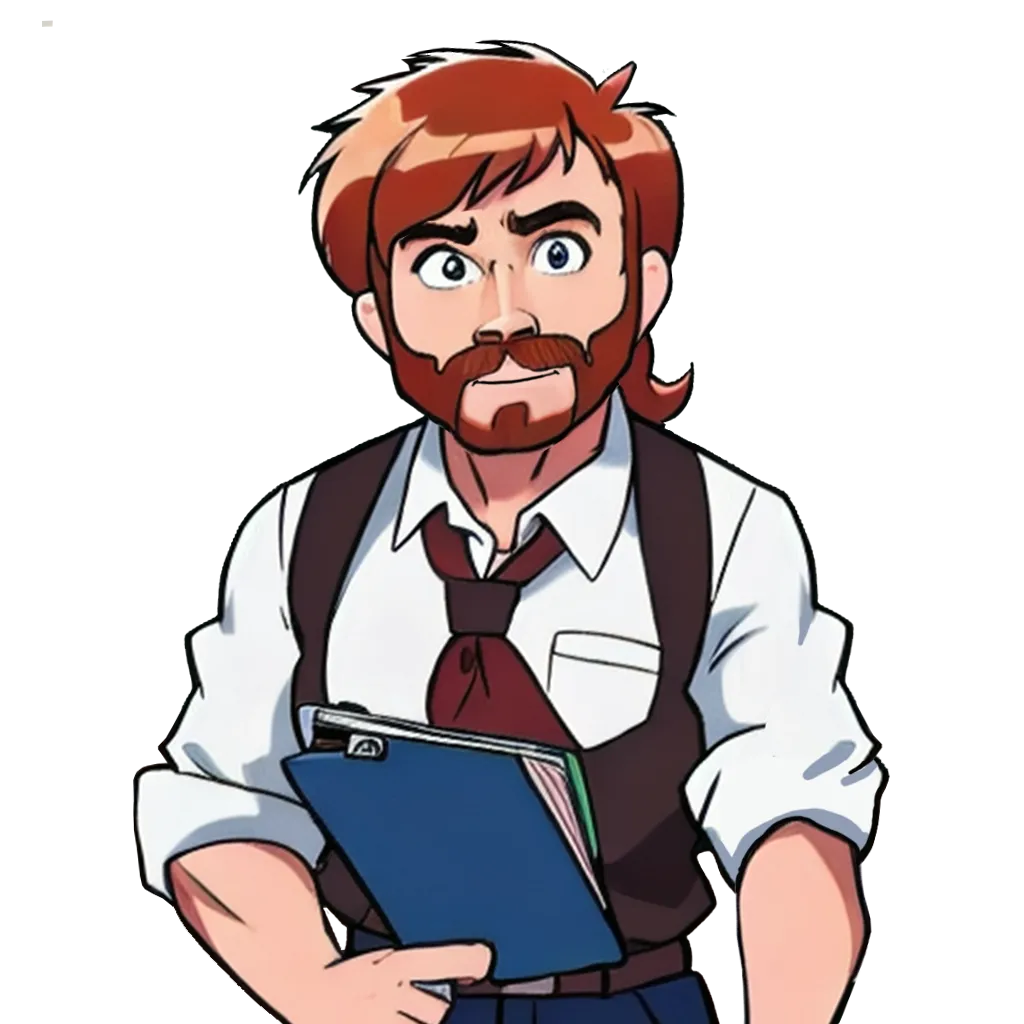
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13