Next.js
Implementing Layouts in the Page Router
In this chapter, we will learn how to implement layouts in a Next.js 13 application using the Page Router. Although Next.js 13's App Router introduced a native system for working with layouts, the Page Router also allows for creating reusable layouts using custom components.
Layouts enable creating a consistent structure across the application, including common elements like headers, footers, or navigation bars, without having to repeat code on each page.
Creating Layouts in the Page Router
In the Page Router, layouts do not have native support like in the App Router, but we can manually organize them as reusable components. Next, we'll see how to create a basic layout that applies to several pages of our application.
Basic Layout
Let's create a basic layout that includes a header and a footer. This layout will wrap the content of our pages.
javascript
The layout Layout.js
can be used on various pages to maintain UI consistency.
Applying the Layout to a Page
Once we have created the layout, we can apply it to pages by importing and wrapping the content of each page within the Layout
component.
javascript
This pattern allows reusing the layout across multiple pages without duplicating code.
Nested Layouts
In some applications, it can be useful to have nested layouts, where certain UI components are shared between different routes, but other components vary. To implement nested layouts in the Page Router, we can combine multiple layout components.
Example of Nested Layout
Let's assume we have a general layout for the entire application, but we want a specific layout for the admin section (/admin
).
javascript
javascript
In this example, we reuse the general layout for the entire application, but add a specific layout with a side navigation bar for the admin section.
Advantages of Using Layouts in the Page Router
Although the Page Router does not have native support for layouts like the App Router, implementing custom layouts has several advantages:
-
Code Reuse: Layouts allow keeping common components, like headers and footers, in one place.
-
UI Consistency: Applying a global layout to all pages ensures the application has a consistent appearance.
-
Flexibility: Nested layouts allow adapting different sections of the application with their own specific components.
Layout Optimization
To improve application performance when using layouts, we can apply techniques like dynamic loading of components that are not critical for the initial render.
Example of Dynamic Loading
We can use Next.js's dynamic()
function to load components dynamically. This is useful for parts of the layout that are not essential at the moment of the first render.
javascript
This can help to reduce the initial load time, especially if the footer or any other part of the layout contains content that is not critical.
Conclusion
In this chapter, we have learned how to implement reusable and nested layouts in a Next.js 13 application using the Page Router. Layouts allow us to maintain UI consistency and reduce code duplication. Although the Page Router does not have native support for layouts like the App Router, we can create custom layouts that fit the needs of our application.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
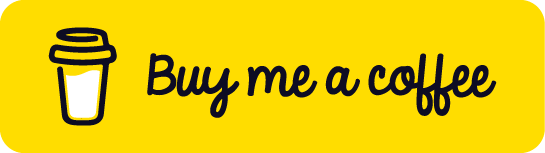
Chat with Chuck
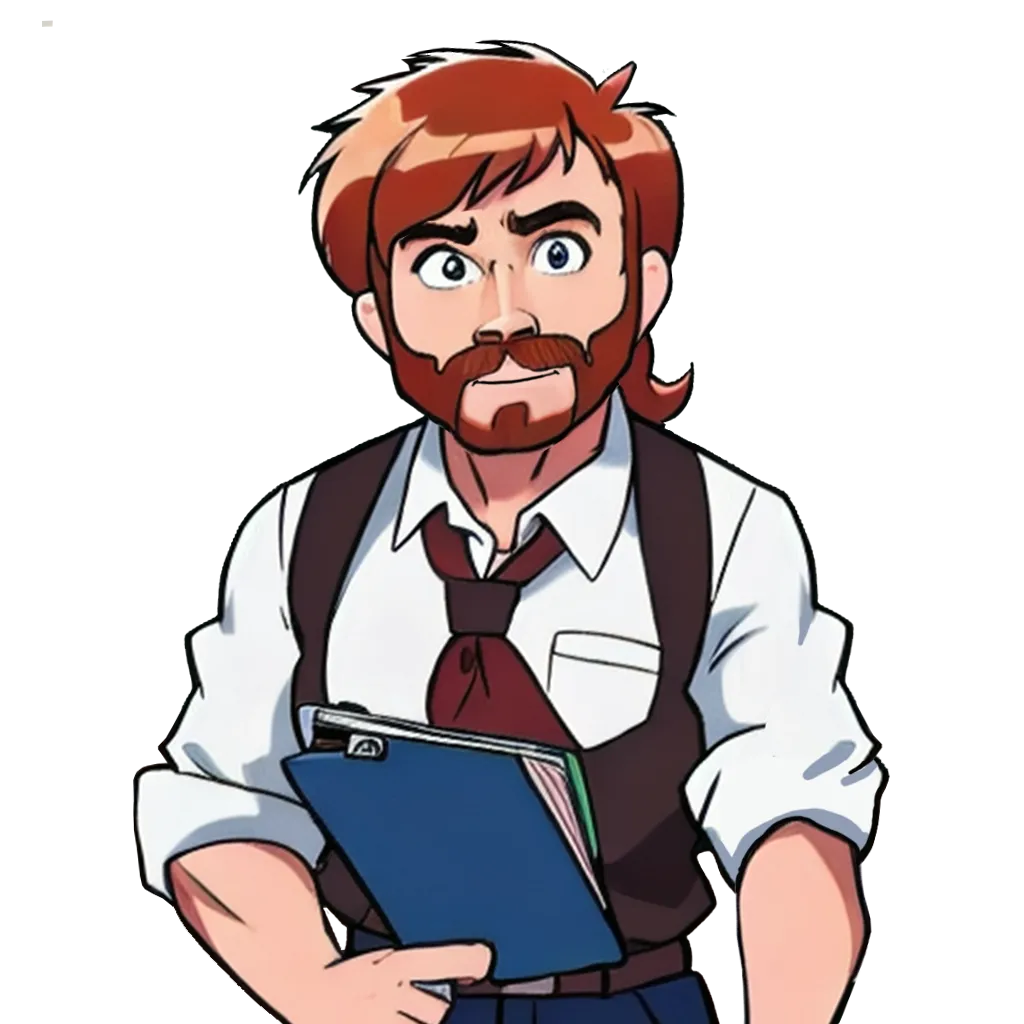
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13