Next.js
Handling Static Files and Assets in the Page Router
In this chapter, we will learn how to handle static files and assets in a Next.js 13 application using the Page Router. Next.js provides an easy way to serve files like images, fonts, CSS files, and other static resources from the public
folder, making them easily accessible and manageable.
The public
Folder in Next.js
The public/
folder in Next.js is where we store all static files. These files are served directly to the client and can be accessed using routes based on their structure within the folder.
For example, if you have a logo.png
file in the public/images
folder, it will be accessible from the URL /images/logo.png
.
Static File Example
Suppose we have an image named logo.png
in the public/images
folder. We can access this image directly from the browser using:
And we can use it within our components as follows:
javascript
This approach allows easy access to static files without the need for additional configurations.
Serving Custom CSS and JavaScript Files
In addition to images, we can store CSS and JavaScript files in the public
folder to be used throughout the application. To include a CSS or JavaScript file, simply link it in your layout or page file.
Including a CSS File
If we have a CSS file in public/styles/global.css
, we can include it in our pages using the <link>
tag in the _document.js
file or directly in the pages.
javascript
Including External JavaScript Files
Similarly, we can link external JavaScript files to be available on our pages. For example, if you have a custom script file scripts/main.js
in the public
folder, you can include it like this:
javascript
This is useful for adding custom functionalities to your application without having to depend on third-party libraries.
Managing Custom Fonts
If you need to use custom fonts in your application, you can store them in the public
folder and use them in your CSS files or directly in your components.
Custom Font Example
If you have a font named MyFont.ttf
in the public/fonts
folder, you can include it in your CSS file as follows:
css
In this way, you can customize the typography of your application without relying on external fonts.
Downloadable Files
If you want users to download files like PDFs or documents from your application, you can place these files in the public
folder and link them directly.
Downloadable File Example
javascript
Files stored in the public
folder can be downloaded directly without additional configurations.
Best Practices for Handling Static Files
It's important to follow some best practices when handling static files and resources in a Next.js application to optimize performance:
-
Optimize images: Use the Next.js
Image
component to take advantage of automatic image optimization. -
Minimize CSS and JavaScript files: Whenever possible, minify and compress your CSS and JavaScript files to improve load times.
-
Avoid unnecessary static files: Do not store large or unnecessary files in the
public
folder. This can increase your application's bundle size and affect performance. -
Use relative paths: Always use relative paths when linking files within the
public
folder to ensure that files are accessible across all routes.
Conclusion
Handling static files and assets in Next.js 13 with the Page Router is very straightforward thanks to the public
folder. From images to CSS files, JavaScript, and custom fonts, Next.js provides a clear and efficient structure to manage these resources. By following best practices, we can ensure that our application is fast and efficient when handling static files.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
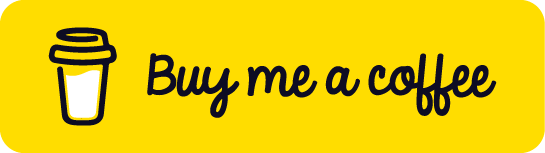
Chat with Chuck
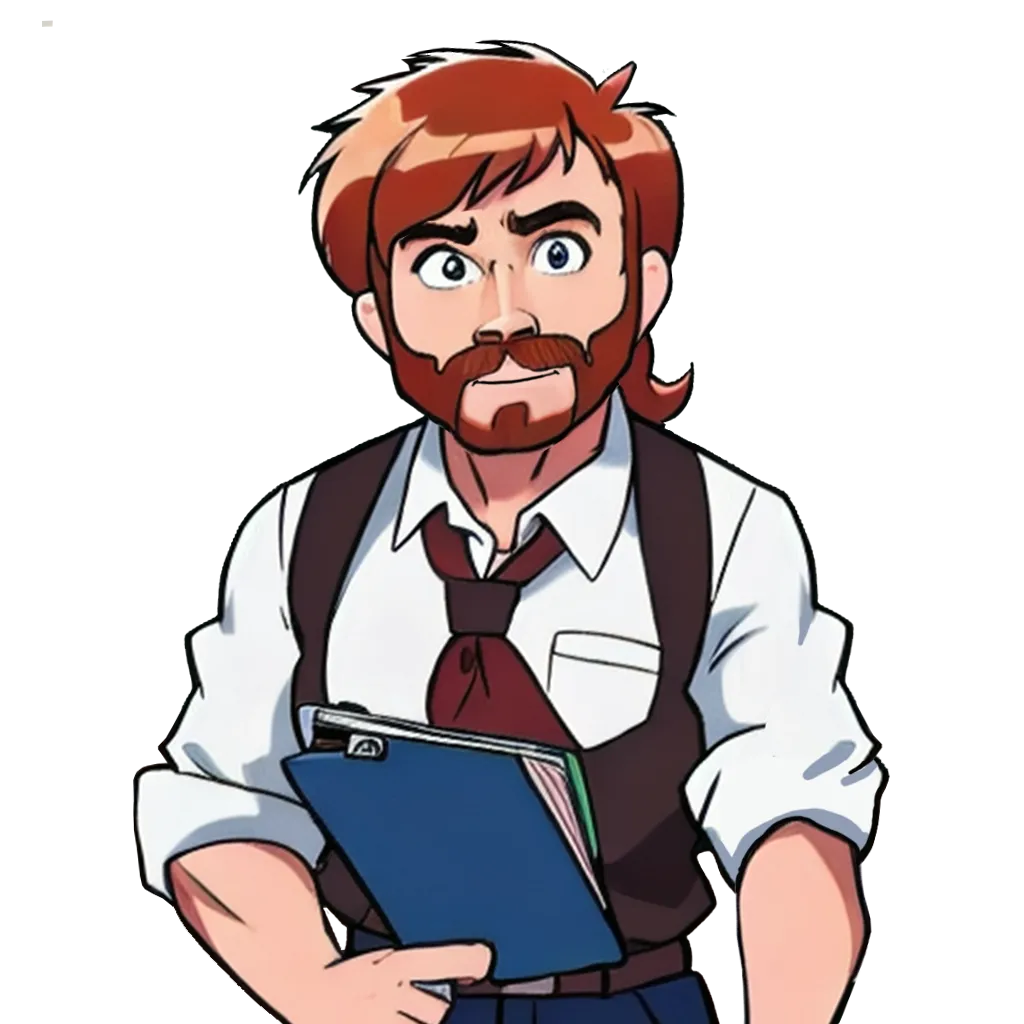
- Introduction to Next.js 13 and the App Router
- Project Structure in Next.js with App Router
- Pages and Routes in the App Router
- Page Rendering and SSR in the App Router
- Using Layouts in the App Router
- Data Fetching in Next.js 13 with App Router
- Uso de Hooks y Context API con el App Router
- Authentication and Authorization in the App Router
- Optimization and Performance in Next.js 13 (App Router)
- Internationalization (i18n) in Next.js 13 with App Router
- Introduction to Page Router and Differences with App Router
- Routes and Navigation in the Page Router
- Data Fetching in the Page Router
- Pages with SSR and SSG in the Page Router
- Implementing Layouts in the Page Router
- Handling Static Files and Assets in the Page Router
- Redirects and Rewrites in the Page Router
- Error Handling and States in the Page Router
- Migration of Projects between App Router and Page Router
- Deployment and Best Practices in Next.js 13